Go Slice Reverse
Reversing a slice in Go involves rearranging its elements in the opposite order. While Go does not provide a built-in function for reversing slices, you can achieve this by implementing a custom function using simple loop logic.
In this tutorial, we will explore how to reverse a slice in Go with practical examples and detailed explanations.
Steps to Reverse a Slice
- Iterate Through the Slice: Use a loop to swap the elements at the beginning and end of the slice.
- Swap Elements: Exchange the positions of elements from the start and end until the middle of the slice is reached.
- In-Place Modification: Perform the reversal directly on the existing slice to avoid creating a new slice.
Examples of Reversing a Slice
1 Reverse a Slice of Integers
This example demonstrates how to reverse a slice of integers in Go:
</>
Copy
package main
import "fmt"
// Function to reverse a slice in place
func reverseSlice(slice []int) {
for i, j := 0, len(slice)-1; i < j; i, j = i+1, j-1 {
slice[i], slice[j] = slice[j], slice[i]
}
}
func main() {
// Declare and initialize a slice
numbers := []int{10, 20, 30, 40, 50}
// Reverse the slice
reverseSlice(numbers)
// Print the reversed slice
fmt.Println("Reversed Slice:", numbers)
}
Explanation
- Define Function: The
reverseSlice
function takes a slice and uses two pointersi
andj
to iterate from the start and end of the slice, respectively. - Swap Elements: The elements at indices
i
andj
are swapped in each iteration. - In-Place Modification: The original slice is modified in place, and no new slice is created.
- Print Result: The reversed slice is printed in the main function.
Output
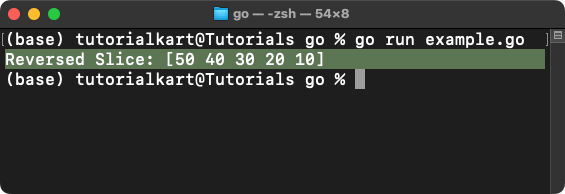
2 Reverse a Slice of Strings
This example demonstrates how to reverse a slice of strings:
</>
Copy
package main
import "fmt"
// Function to reverse a slice in place
func reverseSlice(slice []string) {
for i, j := 0, len(slice)-1; i < j; i, j = i+1, j-1 {
slice[i], slice[j] = slice[j], slice[i]
}
}
func main() {
// Declare and initialize a slice
words := []string{"Go", "is", "awesome"}
// Reverse the slice
reverseSlice(words)
// Print the reversed slice
fmt.Println("Reversed Slice:", words)
}
Explanation
- Define Function: The
reverseSlice
function takes a slice of strings and swaps elements at indicesi
andj
. - Iterate: The loop continues until
i
is greater than or equal toj
, ensuring all elements are swapped. - In-Place Modification: The original slice of strings is reversed in place.
- Print Result: The reversed slice is printed in the main function.
Output
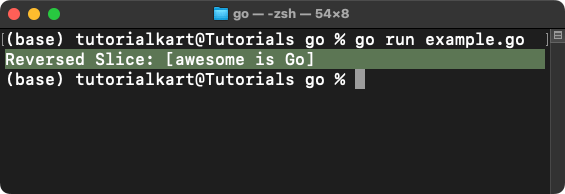
Points to Remember
- No Built-In Functionality: Go does not provide a built-in function to reverse slices, so you must implement it manually.
- In-Place Modification: The reversal operation modifies the original slice instead of creating a new one.
- Performance: Reversing a slice has a time complexity of
O(n)
and does not require additional memory. - Generic Approach: You can use the same logic to reverse slices of different types, such as integers, strings, or custom types.