Go Slice Union
In Go, the union of two slices refers to combining elements from both slices into a single slice, ensuring that no duplicate elements are included. Go does not provide a built-in function for this operation, but it can be implemented using maps for efficient deduplication.
In this tutorial, we will explore how to compute the union of two slices in Go with practical examples and detailed explanations.
Steps to Compute the Union of Two Slices
- Initialize a Map: Use a map to store elements as keys to avoid duplicates.
- Add Elements: Add all elements from both slices to the map.
- Create the Result: Convert the map keys back into a slice to get the union.
Examples of Calculating Slice Union
1 Union of Two Slices of Integers
This example demonstrates how to compute the union of two slices of integers:
</>
Copy
package main
import "fmt"
// Function to compute the union of two slices
func sliceUnion(slice1, slice2 []int) []int {
unionMap := make(map[int]bool)
result := []int{}
// Add elements from the first slice to the map
for _, v := range slice1 {
if !unionMap[v] {
unionMap[v] = true
result = append(result, v)
}
}
// Add elements from the second slice to the map
for _, v := range slice2 {
if !unionMap[v] {
unionMap[v] = true
result = append(result, v)
}
}
return result
}
func main() {
// Declare and initialize two slices
slice1 := []int{10, 20, 30, 40}
slice2 := []int{30, 40, 50, 60}
// Calculate the union
union := sliceUnion(slice1, slice2)
// Print the result
fmt.Println("Union:", union)
}
Explanation
- Initialize Map: A map is used to track elements that have already been added to the result slice.
- Add Elements from Slice 1: Elements from
slice1
are added to the map and appended to the result slice if they are not already present in the map. - Add Elements from Slice 2: Elements from
slice2
are processed similarly, ensuring no duplicates are added. - Return Result: The result slice contains the union of both slices without duplicates.
Output
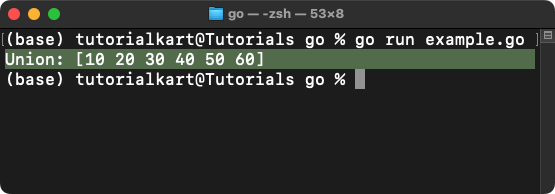
2 Union of Two Slices of Strings
This example demonstrates how to compute the union of two slices of strings:
</>
Copy
package main
import "fmt"
// Function to compute the union of two slices
func sliceUnion(slice1, slice2 []string) []string {
unionMap := make(map[string]bool)
result := []string{}
// Add elements from the first slice to the map
for _, v := range slice1 {
if !unionMap[v] {
unionMap[v] = true
result = append(result, v)
}
}
// Add elements from the second slice to the map
for _, v := range slice2 {
if !unionMap[v] {
unionMap[v] = true
result = append(result, v)
}
}
return result
}
func main() {
// Declare and initialize two slices
slice1 := []string{"apple", "banana", "cherry"}
slice2 := []string{"cherry", "date", "elderberry"}
// Calculate the union
union := sliceUnion(slice1, slice2)
// Print the result
fmt.Println("Union:", union)
}
Explanation
- Initialize Map: A map is used to track elements that have already been added to the result slice.
- Add Elements from Slice 1: Elements from
slice1
are added to the map and appended to the result slice if they are not already present in the map. - Add Elements from Slice 2: Elements from
slice2
are processed similarly, ensuring no duplicates are added. - Return Result: The result slice contains the union of both slices without duplicates.
Output
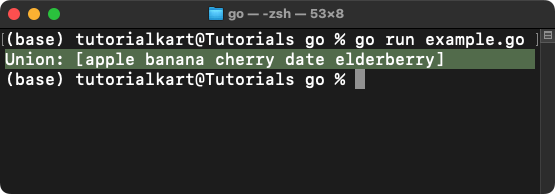
Points to Remember
- Efficiency: Using a map ensures an efficient implementation with a time complexity of
O(n + m)
, wheren
andm
are the lengths of the two slices. - Order Preservation: The order of elements in the result slice matches their order of appearance in the input slices.
- Generic Logic: The same logic can be applied to slices of other types, such as integers, strings, or custom structs.
- Deduplication: Maps are a powerful tool for eliminating duplicates during union operations.