Go Slice Unpack
Unpacking a slice in Go refers to accessing or extracting individual elements of a slice into separate variables. Unlike some other programming languages that provide syntax for unpacking, Go requires you to explicitly access elements by their index or use loops to handle dynamic slice sizes.
In this tutorial, we will explore how to unpack a slice in Go with practical examples and detailed explanations.
Steps to Unpack a Slice
- Access Elements by Index: Use indices to access individual elements of a slice.
- Assign Elements to Variables: Assign the desired elements to variables for further processing.
- Handle Dynamic Slices: Use loops to unpack slices with an unknown or variable size.
Examples of Unpacking a Slice
1 Unpack a Slice of Integers into Variables
This example demonstrates how to unpack a slice of integers into individual variables:
</>
Copy
package main
import "fmt"
func main() {
// Declare and initialize a slice
numbers := []int{10, 20, 30, 40, 50}
// Unpack the first three elements into variables
first, second, third := numbers[0], numbers[1], numbers[2]
// Print the unpacked variables
fmt.Println("First Element:", first)
fmt.Println("Second Element:", second)
fmt.Println("Third Element:", third)
}
Explanation
- Access by Index: The elements at indices
0
,1
, and2
are accessed from the slice. - Assign to Variables: These elements are assigned to the variables
first
,second
, andthird
. - Print Results: The unpacked variables are printed to show the extracted values.
Output
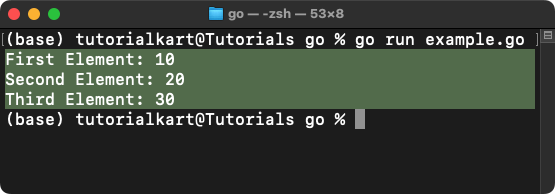
2 Unpack a Slice Dynamically Using a Loop
This example demonstrates how to unpack all elements of a slice dynamically using a loop:
</>
Copy
package main
import "fmt"
func main() {
// Declare and initialize a slice
numbers := []int{10, 20, 30, 40, 50}
// Unpack elements dynamically
for i, v := range numbers {
fmt.Printf("Element %d: %d\n", i, v)
}
}
Explanation
- Use Loop: The
for
loop withrange
iterates over the slice. - Access Elements: In each iteration, the index and value of the current element are retrieved.
- Print Elements: The index and value are printed to show the unpacked elements dynamically.
Output
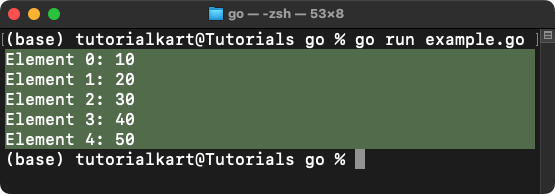
Points to Remember
- Index Access: Use indices to access specific elements for unpacking when the slice size is fixed or known.
- Dynamic Unpacking: Use loops for unpacking slices with variable sizes.
- Memory Sharing: Unpacking elements does not create a new slice; the original slice remains unchanged.
- Bounds Checking: Always ensure indices are within the slice’s bounds to avoid runtime errors.