Go Slice with Unique Values
In Go, slices can contain duplicate elements, but sometimes you need a slice with only unique values. Achieving this requires filtering the slice to remove duplicates. Go does not provide a built-in function for this, but you can implement it using maps for efficient duplicate removal.
In this tutorial, we will explore how to create a slice with unique values in Go using practical examples and detailed explanations.
Steps to Create a Slice with Unique Values
- Use a Map: Create a map to track elements that have already been added to the result slice.
- Iterate Through the Slice: Check each element against the map to determine if it is a duplicate.
- Append Unique Elements: Add elements to the result slice only if they are not already in the map.
Examples of Creating a Slice with Unique Values
1. Remove Duplicates from a Slice of Integers
This example demonstrates how to remove duplicates from a slice of integers:
</>
Copy
package main
import "fmt"
// Function to create a slice with unique values
func uniqueSlice(slice []int) []int {
uniqueMap := make(map[int]bool)
result := []int{}
for _, v := range slice {
if !uniqueMap[v] {
uniqueMap[v] = true
result = append(result, v)
}
}
return result
}
func main() {
// Declare and initialize a slice with duplicate values
numbers := []int{10, 20, 30, 20, 40, 10, 50}
// Create a slice with unique values
uniqueNumbers := uniqueSlice(numbers)
// Print the result
fmt.Println("Original Slice:", numbers)
fmt.Println("Unique Slice:", uniqueNumbers)
}
Explanation
- Initialize Map: A map is created to track elements that have already been added to the result slice.
- Iterate Through Slice: Each element of the slice is checked against the map.
- Filter Duplicates: If an element is not present in the map, it is added to the result slice and marked as seen in the map.
- Return Result: The result slice containing only unique elements is returned.
Output
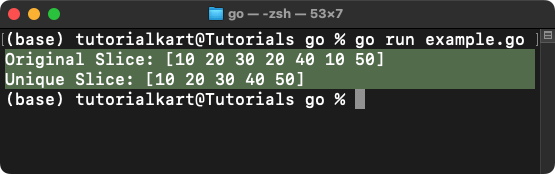
2. Remove Duplicates from a Slice of Strings
This example demonstrates how to remove duplicates from a slice of strings:
</>
Copy
package main
import "fmt"
// Function to create a slice with unique values
func uniqueSlice(slice []string) []string {
uniqueMap := make(map[string]bool)
result := []string{}
for _, v := range slice {
if !uniqueMap[v] {
uniqueMap[v] = true
result = append(result, v)
}
}
return result
}
func main() {
// Declare and initialize a slice with duplicate values
words := []string{"apple", "banana", "cherry", "apple", "date", "banana"}
// Create a slice with unique values
uniqueWords := uniqueSlice(words)
// Print the result
fmt.Println("Original Slice:", words)
fmt.Println("Unique Slice:", uniqueWords)
}
Explanation
- Initialize Map: A map is created to track elements that have already been added to the result slice.
- Iterate Through Slice: Each element of the slice is checked against the map.
- Filter Duplicates: If an element is not present in the map, it is added to the result slice and marked as seen in the map.
- Return Result: The result slice containing only unique elements is returned.
Output
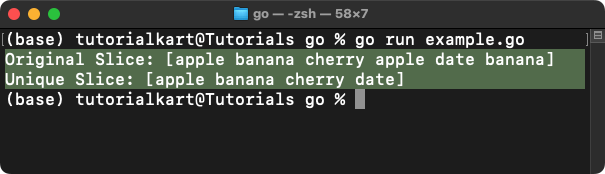
Points to Remember
- Efficient Deduplication: Using a map ensures efficient removal of duplicates with a time complexity of
O(n)
, wheren
is the length of the slice. - Preserve Order: The order of elements in the result slice matches their order of first occurrence in the original slice.
- Generic Logic: The same approach can be applied to slices of other types, such as integers, strings, or custom structs.
- Memory Consideration: Using a map requires additional memory proportional to the number of unique elements in the slice.