Go – Smallest of Three Numbers
In this tutorial, we will learn how to find the smallest of three numbers using the Go programming language (Golang). Identifying the smallest number among three inputs demonstrates the use of conditional logic in programming. We will write a simple program with detailed explanations to achieve this.
Logic to Find the Smallest of Three Numbers
The logic for finding the smallest of three numbers involves comparing the three numbers using conditional statements:
- Compare the First Two Numbers: Check if the first number is smaller than or equal to the second.
- Compare the Result with the Third Number: If the first number is smaller than the second, compare it with the third number. Otherwise, compare the second number with the third.
- Determine the Smallest: The smallest number is the result of these comparisons.
Example Program to Find the Smallest of Three Numbers
In this example, we will take three numbers, use logical operators and conditional statements, to find smallest of the three numbers.
Program – example.go
</>
Copy
package main
import "fmt"
func main() {
// Declare variables to hold three numbers
var num1, num2, num3 int
// Prompt the user to enter three numbers
fmt.Print("Enter three integers separated by space: ")
fmt.Scan(&num1, &num2, &num3)
// Find the smallest number
var smallest int
if num1 <= num2 && num1 <= num3 {
smallest = num1
} else if num2 <= num1 && num2 <= num3 {
smallest = num2
} else {
smallest = num3
}
// Print the smallest number
fmt.Println("The smallest number is:", smallest)
}
Explanation of Program
- Declare Variables: Three integer variables,
num1
,num2
, andnum3
, are declared to store the user input. - Prompt the User: The program prompts the user to enter three integers separated by spaces.
- Read the Inputs: The
fmt.Scan
function reads the inputs and stores them in the declared variables. - Find the Smallest: Conditional statements are used to compare the three numbers. The variable
smallest
stores the smallest number based on the comparisons. - Print the Smallest: The program prints the smallest number to the console.
Output
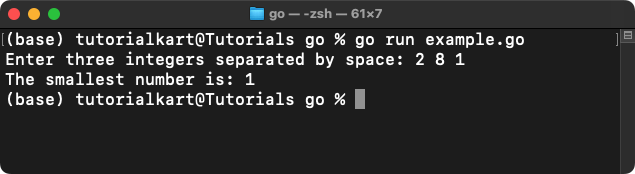
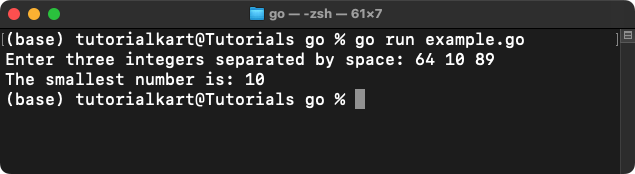
This program efficiently finds the smallest number among three inputs using conditional statements and displays the result.