Go – Subslice
In Go, you can create a subslice from an existing slice by specifying a range of indices. This operation does not copy the underlying data but instead creates a new view into the original slice.
In this tutorial, we will explore how to create subslices in Go with practical examples and detailed explanations.
Steps to Create a Subslice
- Use Slicing Syntax: Use the syntax
slice[start:end]
to specify the range of indices for the subslice. - Understand Index Boundaries: The range includes the
start
index but excludes theend
index. - Preserve the Original Slice: The subslice references the same underlying array as the original slice.
Examples of Creating a Subslice
1. Create a Subslice from a Slice of Integers
This example demonstrates how to create a subslice from a slice of integers:
</>
Copy
package main
import "fmt"
func main() {
// Declare and initialize a slice
numbers := []int{10, 20, 30, 40, 50}
// Create a subslice from index 1 to 4
subslice := numbers[1:4]
// Print the original slice and subslice
fmt.Println("Original Slice:", numbers)
fmt.Println("Subslice:", subslice)
}
Explanation
- Initialize Slice: The slice
numbers
is initialized with five elements. - Create Subslice: The subslice is created using
numbers[1:4]
, which includes elements at indices1
,2
, and3
. - Print Results: The original slice and the subslice are printed to show the result.
Output
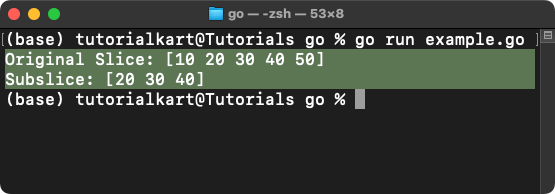
Original Slice: [10 20 30 40 50]
Subslice: [20 30 40]
2. Create a Subslice from a Slice of Strings
This example demonstrates how to create a subslice from a slice of strings:
</>
Copy
package main
import "fmt"
func main() {
// Declare and initialize a slice
words := []string{"apple", "banana", "cherry", "date", "elderberry"}
// Create a subslice from index 2 to the end
subslice := words[2:]
// Print the original slice and subslice
fmt.Println("Original Slice:", words)
fmt.Println("Subslice:", subslice)
}
Explanation
- Initialize Slice: The slice
words
is initialized with five elements. - Create Subslice: The subslice is created using
words[2:]
, which includes elements from index2
to the end of the slice. - Print Results: The original slice and the subslice are printed to show the result.
Output
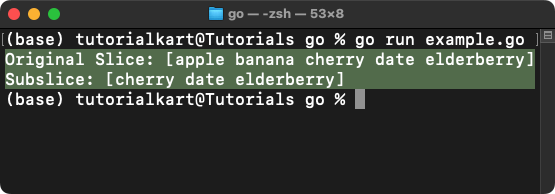
3. Modify the Subslice and Observe the Original Slice
This example demonstrates how modifying a subslice affects the original slice:
</>
Copy
package main
import "fmt"
func main() {
// Declare and initialize a slice
numbers := []int{10, 20, 30, 40, 50}
// Create a subslice
subslice := numbers[1:4]
// Modify the subslice
subslice[0] = 99
// Print the original slice and subslice
fmt.Println("Original Slice after modification:", numbers)
fmt.Println("Subslice after modification:", subslice)
}
Explanation
- Shared Memory: The subslice shares the same underlying array as the original slice.
- Modify Subslice: Changing an element in the subslice also modifies the corresponding element in the original slice.
- Print Results: Both the original slice and the subslice reflect the modification.
Output
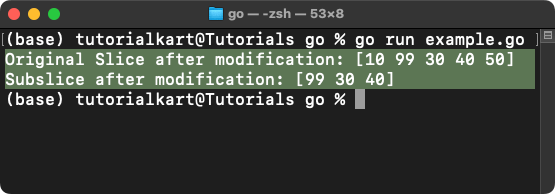
Points to Remember
- Index Boundaries: The slicing syntax includes the start index but excludes the end index.
- Shared Memory: Subslices share the same underlying array as the original slice, so changes to a subslice affect the original slice.
- Performance: Creating a subslice is efficient since it does not copy the underlying data.
- Bounds Checking: Ensure that the indices used for slicing are within the valid range of the original slice.