Go – Sum of Three Numbers
In this tutorial, we will learn how to find the sum of three numbers in the Go programming language (Golang). We’ll explore two methods: using variables directly and implementing a function for reusability. Each example will include the syntax, a program, and a detailed explanation to ensure a clear understanding of the concepts.
Syntax to Find Sum of Three Numbers
The basic syntax for adding three numbers in Go is:
var sum = num1 + num2 + num3
Here, num1
, num2
, and num3
are the numbers to be added, and sum
holds the result of the addition.
Example 1: Sum of Three Numbers Using Variables
In this example, we will define three integer variables, add them, and store the result in another variable. Finally, we will print the result to the console.
Program – example.go
package main
import "fmt"
func main() {
// Declare three variables
num1 := 10
num2 := 20
num3 := 30
// Find the sum
sum := num1 + num2 + num3
// Print the result
fmt.Println("The sum of", num1, ",", num2, "and", num3, "is", sum)
}
Explanation of Program
1. We use the :=
syntax to declare and initialize three integer variables, num1
, num2
, and num3
, with values 10, 20, and 30, respectively.
2. The expression num1 + num2 + num3
calculates the sum of the three variables.
3. The result is stored in the sum
variable.
4. The fmt.Println
function is used to print the result to the console, along with descriptive text.
Output
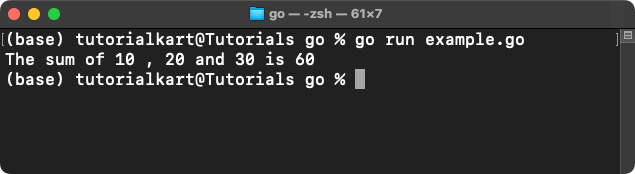
Example 2: Sum of Three Numbers Using a Function
In this example, we will write a function to calculate the sum of three numbers. This approach makes the code reusable, as the function can be called multiple times with different inputs.
Program – example.go
package main
import "fmt"
// Function to find the sum of three numbers
func findSum(a int, b int, c int) int {
return a + b + c
}
func main() {
// Call the function with three numbers
num1 := 15
num2 := 25
num3 := 35
sum := findSum(num1, num2, num3)
// Print the result
fmt.Println("The sum of", num1, ",", num2, "and", num3, "is", sum)
}
Explanation of Program
1. A function findSum
is defined, which takes three integer parameters a
, b
, and c
, and returns their sum.
2. Inside the main
function, three integers, num1
, num2
, and num3
, are initialized with values 15, 25, and 35.
3. The findSum
function is called with num1
, num2
, and num3
as arguments, and the result is stored in the sum
variable.
4. The fmt.Println
function is used to print the result to the console, along with descriptive text.
Output
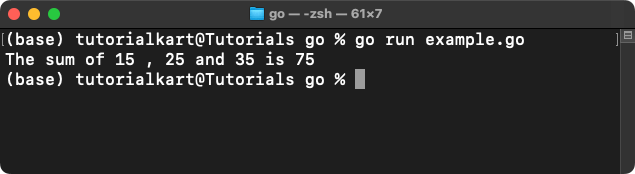