Go – Swap Two Numbers
In this tutorial, we will learn how to swap two numbers using the Go programming language (Golang). Swapping two numbers means exchanging their values. This task demonstrates the use of variables and assignment operators in programming. We will provide a simple program to implement this functionality and explain it step by step.
Logic to Swap Two Numbers
The logic for swapping two numbers involves using a temporary variable to hold the value of one number while exchanging their values:
- Store the First Number: Save the value of the first number in a temporary variable.
- Assign the Second Number: Assign the value of the second number to the first number.
- Restore the First Number: Assign the value of the temporary variable (which holds the original first number) to the second number.
This logic ensures that the values of the two numbers are swapped without being lost.
Example Program to Swap Two Numbers
Program – swap_two_numbers.go
</>
Copy
package main
import "fmt"
func main() {
// Declare variables to hold two numbers
var num1, num2 int
// Prompt the user to enter two numbers
fmt.Print("Enter two integers separated by space: ")
fmt.Scan(&num1, &num2)
// Display the original values
fmt.Println("Before swapping:")
fmt.Println("num1 =", num1, ", num2 =", num2)
// Swap the numbers using a temporary variable
temp := num1
num1 = num2
num2 = temp
// Display the swapped values
fmt.Println("After swapping:")
fmt.Println("num1 =", num1, ", num2 =", num2)
}
Explanation of Program
- Declare Variables: Two integer variables,
num1
andnum2
, are declared to store the user input. - Prompt the User: The program prompts the user to enter two integers separated by space using the
fmt.Print
function. - Read the Inputs: The
fmt.Scan
function reads the inputs and stores them innum1
andnum2
. - Display Original Values: The original values of the numbers are displayed using
fmt.Println
. - Swap the Numbers: A temporary variable
temp
is used to hold the value ofnum1
. Thennum2
is assigned tonum1
, andtemp
(which holds the original value ofnum1
) is assigned tonum2
. - Display Swapped Values: The swapped values of the numbers are displayed using
fmt.Println
.
Output
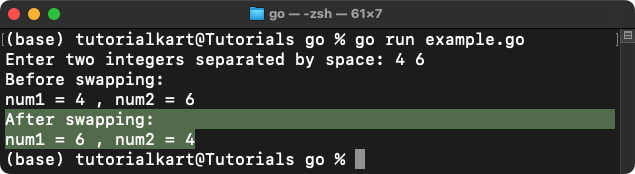
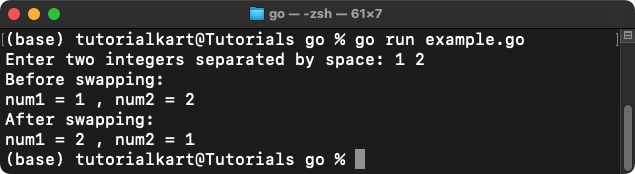
This program successfully swaps the values of two numbers using a temporary variable and displays the result.