Go Variables
In this tutorial, we will explore variables in the Go programming language (Golang).
Variables are essential components of any programming language, as they are used to store and manipulate data.
We will cover the declaration, initialization, and usage of variables in Go with detailed explanations and examples.
What is a Variable?
A variable is a named storage location in memory that holds a value of a specific type. Variables in Go are strongly typed, meaning their type is defined when they are declared and cannot change.
Variable Declaration
In Go, variables can be declared using the var
keyword or shorthand syntax. The syntax for variable declaration is:
var variableName type
Here, variableName
is the name of the variable, and type
is the data type of the variable.
Examples of Variable Declaration
package main
import "fmt"
func main() {
// Declare a variable with type
var name string
var age int
// Assign values to the variables
name = "John"
age = 25
// Print the values
fmt.Println("Name:", name)
fmt.Println("Age:", age)
}
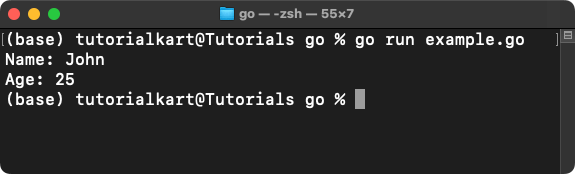
Variable Initialization
Variables can be initialized at the time of declaration. The syntax is:
var variableName type = value
If the type can be inferred from the value, it is optional:
var variableName = value
Examples of Variable Initialization
package main
import "fmt"
func main() {
// Declare and initialize variables
var city string = "New York"
var temperature = 28
// Print the values
fmt.Println("City:", city)
fmt.Println("Temperature:", temperature)
}
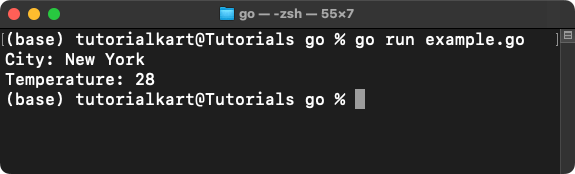
Shorthand Variable Declaration
Go provides a shorthand syntax for declaring and initializing variables using the :=
operator. This method is only allowed inside functions.
variableName := value
Example of Shorthand Variable Declaration
package main
import "fmt"
func main() {
// Shorthand declaration
name := "Alice"
age := 30
// Print the values
fmt.Println("Name:", name)
fmt.Println("Age:", age)
}
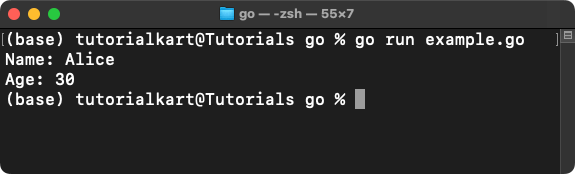
Multiple Variable Declaration
Go allows the declaration of multiple variables in a single statement:
var x, y, z int
Variables can also be initialized simultaneously:
x, y, z := 1, 2, 3
Example of Multiple Variable Declaration
package main
import "fmt"
func main() {
// Declare and initialize multiple variables
x, y, z := 1, 2, 3
// Print the values
fmt.Println("x:", x, "y:", y, "z:", z)
}
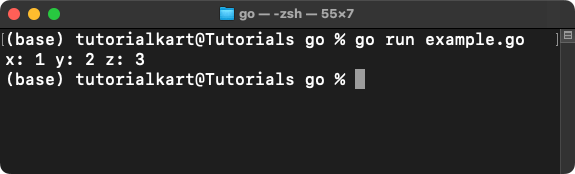
Default Values
If a variable is declared but not initialized, it is assigned a default value based on its type:
- int: 0
- float64: 0.0
- string: “” (empty string)
- bool: false
Example of Default Values
package main
import "fmt"
func main() {
// Declare variables without initialization
var a int
var b float64
var c string
var d bool
// Print default values
fmt.Println("Default int:", a)
fmt.Println("Default float64:", b)
fmt.Println("Default string:", c)
fmt.Println("Default bool:", d)
}
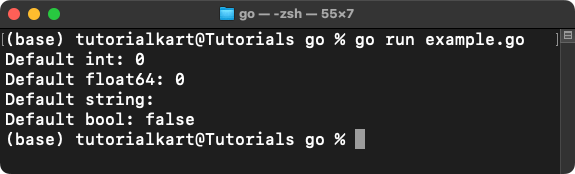
Conclusion
Variables are fundamental building blocks in Go. Understanding their declaration, initialization, and default values is essential for effective programming. Go offers flexibility with shorthand syntax and multiple variable declarations, making it easier to write concise and readable code.