HTML <audio> Tag
The HTML <audio>
tag is used to embed sound content in web pages. This can include music, podcasts, or other audio clips. It provides a standard way to include audio with support for multiple formats.
Basic Example of HTML <audio> Tag
The simplest way to use the <audio>
tag is by embedding an audio file. Here is an example:
index.html
<!DOCTYPE html>
<html>
<body>
<h2>Basic Audio Example</h2>
<audio controls>
<source src="audio-file.mp3" type="audio/mpeg">
<source src="audio-file.ogg" type="audio/ogg">
Your browser does not support the audio element.
</audio>
</body>
</html>
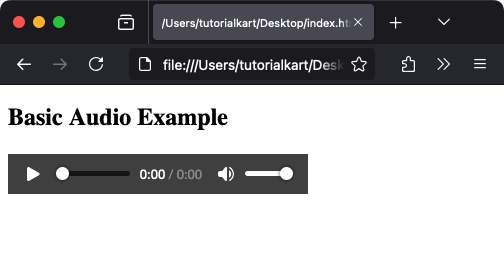
Note: Always include multiple source formats to ensure cross-browser compatibility. Use the controls
attribute to display play, pause, and volume controls.
Attributes of HTML <audio> Tag
The <audio>
tag supports the following attributes:
- controls: Displays audio controls such as play, pause, and volume.
- autoplay: Automatically starts playing the audio when the page loads.
- loop: Replays the audio continuously.
- muted: Mutes the audio by default.
- preload: Specifies how the browser should load the audio. Values can be:
none
: Do not preload the audio.metadata
: Preload only metadata.auto
: Preload the entire audio.
Styling the HTML <audio> Tag
Although the <audio>
element itself cannot be styled, you can customize its placement on the page using CSS. For example:
index.html
<!DOCTYPE html>
<html>
<head>
<style>
audio {
display: block;
margin: 20px auto;
width: 300px;
}
</style>
</head>
<body>
<h2>Styled Audio Player</h2>
<audio controls>
<source src="audio-file.mp3" type="audio/mpeg">
Your browser does not support the audio element.
</audio>
</body>
</html>
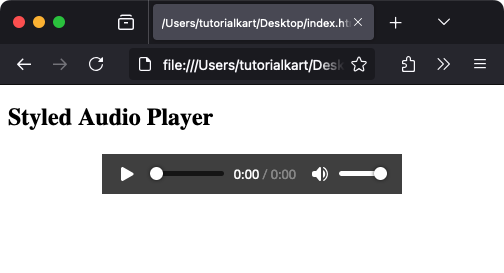
Special Case Examples
Here are some advanced examples demonstrating additional features of the <audio>
tag:
Autoplay with Muted Audio
Some browsers require audio to be muted before allowing it to autoplay.
index.html
<!DOCTYPE html>
<html>
<body>
<h2>Autoplay Muted Audio</h2>
<audio autoplay muted>
<source src="audio-file.mp3" type="audio/mpeg">
Your browser does not support the audio element.
</audio>
</body>
</html>
Custom Audio Player with JavaScript
You can create a custom audio player using JavaScript for advanced control over playback:
index.html
<!DOCTYPE html>
<html>
<head>
<style>
#custom-player {
display: flex;
align-items: center;
gap: 10px;
}
button {
padding: 5px 10px;
}
</style>
</head>
<body>
<h2>Custom Audio Player</h2>
<div id="custom-player">
<button onclick="playAudio()">Play</button>
<button onclick="pauseAudio()">Pause</button>
<audio id="audio-element">
<source src="audio-file.mp3" type="audio/mpeg">
</audio>
</div>
<script>
const audio = document.getElementById('audio-element');
function playAudio() {
audio.play();
}
function pauseAudio() {
audio.pause();
}
</script>
</body>
</html>
Looping Background Music
Use the loop
and autoplay
attributes to create looping background music for your webpage:
index.html
<!DOCTYPE html>
<html>
<body>
<h2>Background Music</h2>
<audio autoplay loop>
<source src="background-music.mp3" type="audio/mpeg">
Your browser does not support the audio element.
</audio>
</body>
</html>