HTML <canvas> Tag
The HTML <canvas>
tag is used to draw graphics via scripting (usually JavaScript). It provides a drawable region defined in height and width attributes and allows you to render shapes, images, text, and animations dynamically.
The <canvas>
tag does not display anything by itself. All drawing operations must be performed via JavaScript.
Basic Example of HTML <canvas> Tag
Here’s a simple example of drawing a rectangle on a canvas:
index.html
<!DOCTYPE html>
<html>
<head>
<title>Canvas Example</title>
</head>
<body>
<h2>Canvas Example</h2>
<canvas id="myCanvas" width="400" height="200" style="border:1px solid #000000;">
Your browser does not support the canvas element.
</canvas>
<script>
const canvas = document.getElementById("myCanvas");
const ctx = canvas.getContext("2d");
ctx.fillStyle = "#FF0000";
ctx.fillRect(50, 50, 150, 100);
</script>
</body>
</html>
Explanation: The getContext('2d')
method provides a 2D drawing context for the canvas, allowing shapes and graphics to be rendered.
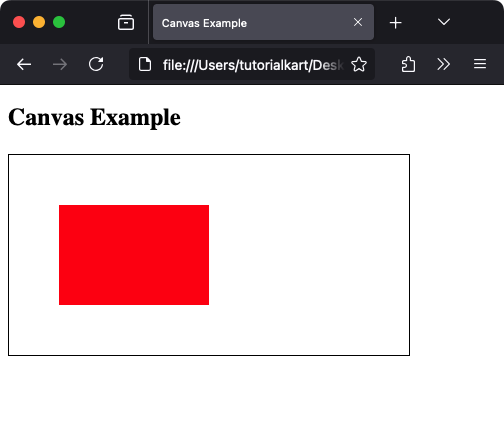
Attributes of HTML <canvas> Tag
- width: Specifies the width of the canvas in pixels (default is 300px).
- height: Specifies the height of the canvas in pixels (default is 150px).
These attributes set the size of the canvas area. If not defined, default values are used. You can also set dimensions using CSS, but that only scales the canvas and does not change its drawing surface size.
Drawing on the Canvas
Here are some common drawing operations using the canvas
element:
Drawing Shapes on Canvas
You can draw rectangles, circles, and paths using the canvas context:
<!DOCTYPE html>
<html>
<body>
<canvas id="myCanvas" width="400" height="200" style="border:1px solid #000000;"></canvas>
<script>
const canvas = document.getElementById("myCanvas");
const ctx = canvas.getContext("2d");
// Draw a rectangle
ctx.fillStyle = "#FF0000";
ctx.fillRect(50, 50, 150, 100);
// Draw a circle
ctx.beginPath();
ctx.arc(250, 100, 50, 0, 2 * Math.PI);
ctx.fillStyle = "#00FF00";
ctx.fill();
// Draw a line
ctx.beginPath();
ctx.moveTo(50, 150);
ctx.lineTo(350, 150);
ctx.strokeStyle = "#0000FF";
ctx.stroke();
</script>
</body>
</html>
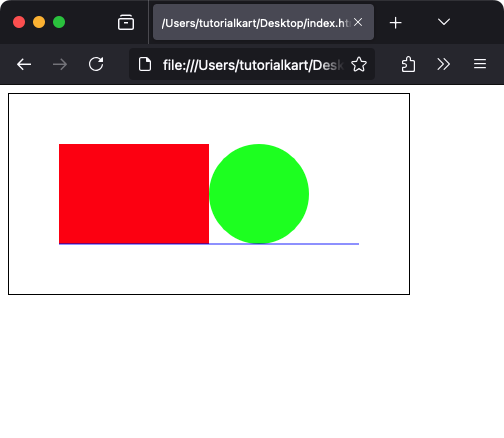
Drawing Text on Canvas
You can also render text on the canvas:
<!DOCTYPE html>
<html>
<body>
<canvas id="textCanvas" width="400" height="200" style="border:1px solid #000000;"></canvas>
<script>
const canvas = document.getElementById("textCanvas");
const ctx = canvas.getContext("2d");
ctx.font = "20px Arial";
ctx.fillStyle = "black";
ctx.fillText("Hello, Canvas!", 50, 100);
</script>
</body>
</html>
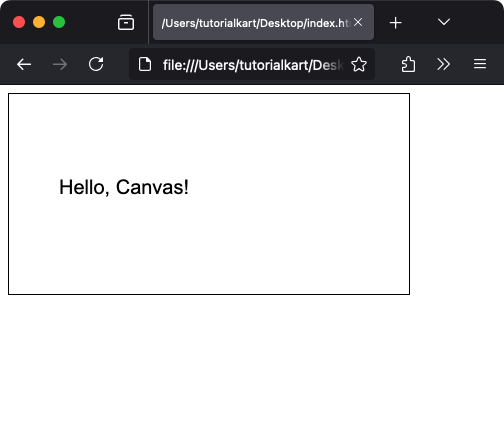
Special Cases for HTML <canvas> Tag
Using Images on Canvas
You can draw images on the canvas using the drawImage
method:
<!DOCTYPE html>
<html>
<body>
<canvas id="imageCanvas" width="400" height="200" style="border:1px solid #000000;"></canvas>
<script>
const canvas = document.getElementById("imageCanvas");
const ctx = canvas.getContext("2d");
const img = new Image();
img.onload = function() {
ctx.drawImage(img, 50, 50, 100, 100);
};
img.src = "image.jpg";
</script>
</body>
</html>
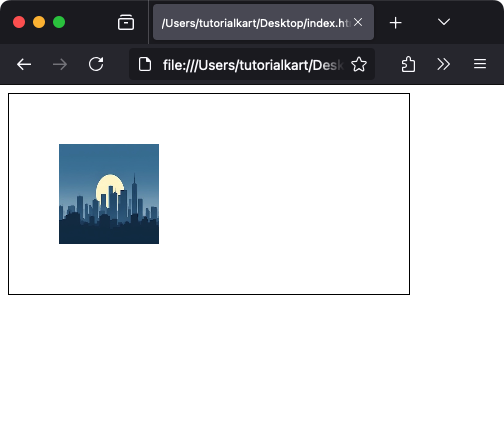
Animation on Canvas
You can create animations using the requestAnimationFrame
function:
<!DOCTYPE html>
<html>
<body>
<canvas id="animationCanvas" width="400" height="200" style="border:1px solid #000000;"></canvas>
<script>
const canvas = document.getElementById("animationCanvas");
const ctx = canvas.getContext("2d");
let x = 0;
function animate() {
ctx.clearRect(0, 0, canvas.width, canvas.height);
ctx.fillStyle = "#FF0000";
ctx.fillRect(x, 100, 50, 50);
x += 2;
if (x > canvas.width) x = -50;
requestAnimationFrame(animate);
}
animate();
</script>
</body>
</html>
The <canvas>
tag is a powerful tool for creating dynamic, interactive, and visually appealing web content.