HTML <div> Tag
The HTML <div>
tag is a block-level container element that is used to group content and apply styling or scripts to it. It is one of the most versatile and commonly used tags in HTML, often serving as a wrapper for sections of a web page.
Although the <div>
tag does not carry any semantic meaning, it is widely used for layout purposes, combining it with CSS for styling and JavaScript for interactivity.
Basic Syntax of HTML <div> Tag
The basic syntax for the <div>
tag is:
<div>
Content goes here
</div>
The <div>
tag is a block-level element, meaning it starts on a new line and takes up the full width of its container by default.
Attributes of HTML <div> Tag
- Global Attributes: The
<div>
tag supports all global attributes, such asid
,class
,style
, anddata-*
. - Event Attributes: The
<div>
tag supports all event attributes, such asonclick
,onmouseover
, andonkeydown
.
Basic Example of HTML <div> Tag
Here’s a simple example of using the <div>
tag to group content:
index.html
<!DOCTYPE html>
<html>
<body>
<h2>Basic Example</h2>
<div>
<p>This is a paragraph inside a div.</p>
<p>This is another paragraph inside the same div.</p>
</div>
</body>
</html>
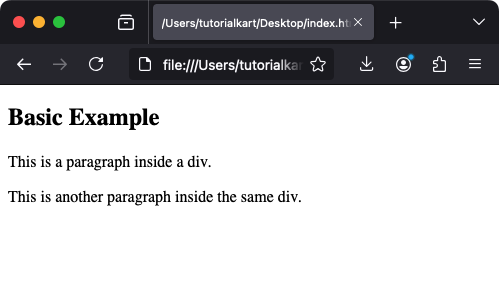
Explanation: In this example, the two paragraphs are grouped inside a single <div>
element, which can be styled or managed as a unit.
Styling the <div> Tag with CSS
The <div>
tag can be styled using CSS to control its appearance:
index.html
<!DOCTYPE html>
<html>
<head>
<style>
.container {
background-color: #f9f9f9;
border: 1px solid #ddd;
padding: 20px;
margin: 10px 0;
}
</style>
</head>
<body>
<h2>Styled Div Example</h2>
<div class="container">
<p>This div has a light gray background, padding, and a border.</p>
</div>
</body>
</html>
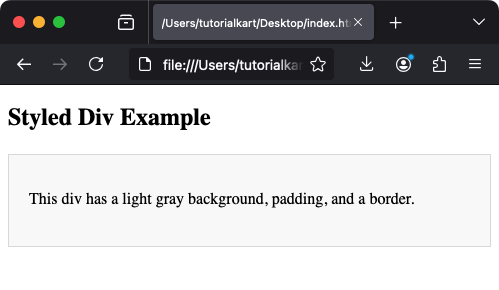
Result: The <div>
element is styled with a background color, padding, and border, making it visually distinct.
Using the <div> Tag for Layout
The <div>
tag is commonly used for layout purposes, often in combination with CSS frameworks or custom styles:
index.html
<!DOCTYPE html>
<html>
<head>
<style>
.row {
display: flex;
}
.column {
flex: 50%;
padding: 10px;
}
</style>
</head>
<body>
<h2>Layout Example</h2>
<div class="row">
<div class="column" style="background-color: #f1f1f1;">
Column 1
</div>
<div class="column" style="background-color: #ddd;">
Column 2
</div>
</div>
</body>
</html>
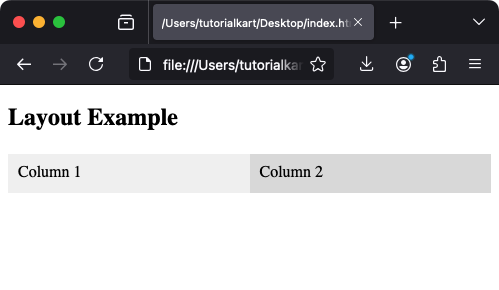
Explanation: This example uses the <div>
tag with CSS Flexbox to create a two-column layout.
Practical Applications of the <div> Tag
- Grouping Elements: Use
<div>
to group related elements, such as sections of a form, articles, or navigation items. - Layout Building: Combine
<div>
with CSS for creating grids, rows, and columns in responsive layouts. - Dynamic Content: Use
<div>
as a container for dynamic content that is manipulated using JavaScript.
Advanced Example: Nested Divs
You can nest multiple <div>
elements to create complex layouts:
index.html
<!DOCTYPE html>
<html>
<head>
<style>
.parent {
display: flex;
justify-content: space-between;
}
.child {
width: 30%;
padding: 10px;
background-color: #f1f1f1;
border: 1px solid #ddd;
}
</style>
</head>
<body>
<h2>Nested Div Example</h2>
<div class="parent">
<div class="child">Box 1</div>
<div class="child">Box 2</div>
<div class="child">Box 3</div>
</div>
</body>
</html>
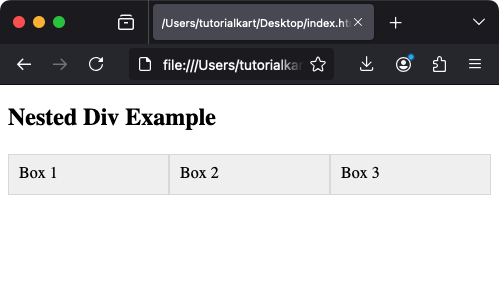
Explanation: This example creates a parent <div>
with three nested child divs arranged in a row using Flexbox.