HTML <fieldset> Tag
The HTML <fieldset>
tag is used to group related elements within a form. It provides a way to logically organize form controls like input fields, dropdowns, and buttons, making the form more accessible and easier to navigate.
Additionally, the <fieldset>
tag can be paired with the <legend>
tag to provide a caption or title for the grouped form elements.
Basic Syntax of HTML <fieldset> Tag
The basic structure of the <fieldset>
tag is:
<fieldset>
<legend>Group Title</legend>
Form controls go here.
</fieldset>
The <legend>
tag provides a descriptive caption for the fieldset, making it more meaningful for users and screen readers.
Attributes of HTML <fieldset> Tag
- disabled: Disables all form elements within the fieldset, making them uneditable.
- form: Specifies the ID of the form the fieldset belongs to (used for advanced form setups).
- Global Attributes: The
<fieldset>
tag supports all global attributes, such asid
,class
, andstyle
.
Basic Example of HTML <fieldset> Tag
Here’s a simple example of using the <fieldset>
tag to group form elements:
index.html
<!DOCTYPE html>
<html>
<body>
<h2>Fieldset Example</h2>
<form>
<fieldset>
<legend>Personal Information</legend>
<label for="name">Name:</label>
<input type="text" id="name" name="name"><br><br>
<label for="email">Email:</label>
<input type="email" id="email" name="email">
</fieldset>
</form>
</body>
</html>
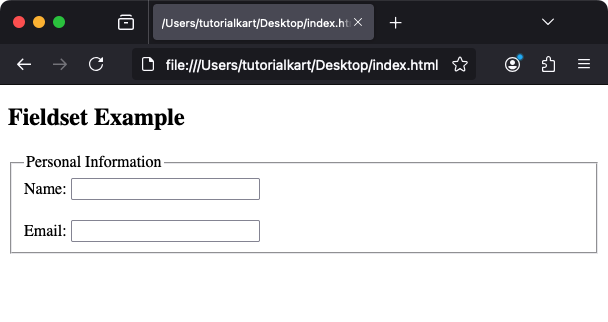
Explanation: The <fieldset>
tag groups the “Name” and “Email” fields together under the “Personal Information” heading, making the form more organized.
Using the disabled Attribute with fieldset
The disabled
attribute makes all form controls inside the <fieldset>
uneditable:
index.html
<!DOCTYPE html>
<html>
<body>
<h2>Disabled Fieldset Example</h2>
<form>
<fieldset disabled>
<legend>Account Information</legend>
<label for="username">Username:</label>
<input type="text" id="username" name="username"><br><br>
<label for="password">Password:</label>
<input type="password" id="password" name="password">
</fieldset>
</form>
</body>
</html>
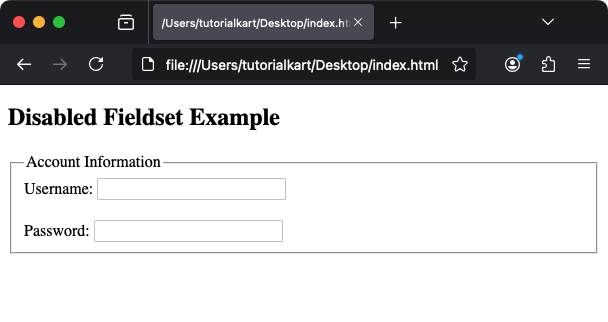
Explanation: All the form controls inside the fieldset are disabled and cannot be interacted with by the user.
Styling the <fieldset> Tag with CSS
The <fieldset>
and <legend>
tags can be styled with CSS to improve their appearance:
index.html
<!DOCTYPE html>
<html>
<head>
<style>
fieldset {
border: 2px solid #007BFF;
border-radius: 5px;
padding: 20px;
margin: 10px 0;
background-color: #f9f9f9;
}
legend {
font-weight: bold;
color: #007BFF;
}
</style>
</head>
<body>
<h2>Styled Fieldset Example</h2>
<form>
<fieldset>
<legend>Contact Information</legend>
<label for="phone">Phone:</label>
<input type="tel" id="phone" name="phone"><br><br>
<label for="address">Address:</label>
<input type="text" id="address" name="address">
</fieldset>
</form>
</body>
</html>
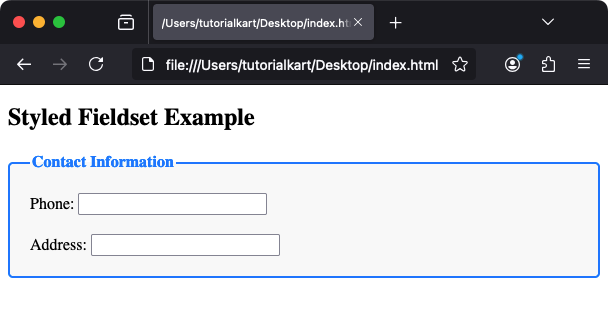
Result: The fieldset has a blue border, rounded corners, and a light background, while the legend text is styled in bold blue.
Nested Fieldsets
You can nest <fieldset>
tags to create hierarchical form sections:
index.html
<!DOCTYPE html>
<html>
<body>
<h2>Nested Fieldsets Example</h2>
<form>
<fieldset>
<legend>User Information</legend>
<label for="username">Username:</label>
<input type="text" id="username" name="username"><br><br>
<fieldset>
<legend>Preferences</legend>
<label>
<input type="checkbox" name="newsletter"> Subscribe to newsletter
</label><br>
<label>
<input type="checkbox" name="updates"> Receive updates
</label>
</fieldset>
</fieldset>
</form>
</body>
</html>
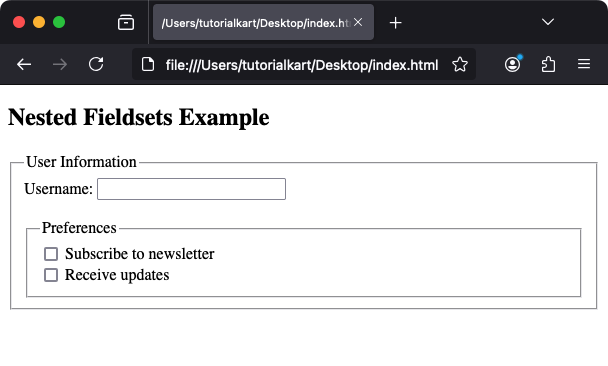
Explanation: The nested fieldset organizes user preferences within the main “User Information” section, improving form clarity and structure.
Practical Applications of the <fieldset> Tag
- Organizing Forms: Group related input fields together for better readability.
- Accessibility: Improve accessibility by providing descriptive legends for form sections.
- Dynamic Form Sections: Use
disabled
fieldsets to toggle sections of a form dynamically with JavaScript.