HTML <form> Tag
The HTML <form>
tag is used to create a form for collecting user input. It acts as a container for various form controls such as text fields, checkboxes, radio buttons, dropdowns, and buttons. Forms are essential for interactive web applications, enabling data collection and submission to a server for processing.
The <form>
tag includes attributes that specify where to send the data, the HTTP method to use, and how the data should be encoded.
Basic Syntax of HTML <form> Tag
The basic structure of the <form>
tag is:
<form action="URL" method="POST or GET">
Form controls go here
</form>
The action
attribute specifies the URL where the form data will be sent, and the method
attribute defines the HTTP method (usually POST
or GET
).
Attributes of HTML <form> Tag
- action: Specifies the URL to which the form data will be submitted.
- method: Defines the HTTP method to be used for form submission. Common values are:
GET
: Sends data as URL parameters.POST
: Sends data in the request body (more secure).
- enctype: Specifies how form data should be encoded when submitted. Common values are:
application/x-www-form-urlencoded
: Default encoding.multipart/form-data
: Used for file uploads.text/plain
: Sends data as plain text.
- target: Specifies where to display the response (e.g.,
_self
,_blank
). - autocomplete: Enables or disables browser autofill (
on
oroff
). - novalidate: Disables HTML form validation.
- Global Attributes: Supports all global attributes, such as
id
,class
, andstyle
.
Basic Example of HTML <form> Tag
Here’s a basic form example that collects a user’s name and email:
index.html
<!DOCTYPE html>
<html>
<body>
<h2>Basic Form Example</h2>
<form action="/submit-form" method="POST">
<label for="name">Name:</label>
<input type="text" id="name" name="name"><br><br>
<label for="email">Email:</label>
<input type="email" id="email" name="email"><br><br>
<button type="submit">Submit</button>
</form>
</body>
</html>
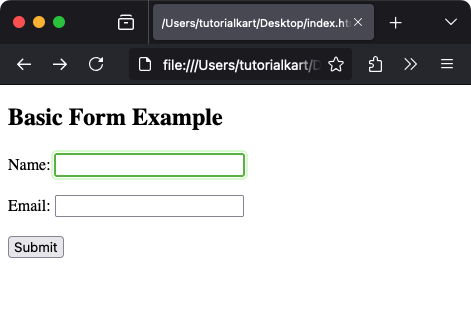
Explanation: The form collects the user’s name and email and submits the data to /submit-form
using the POST
method.
Using the enctype Attribute of form
The enctype
attribute is used for file uploads. Here’s an example:
index.html
<!DOCTYPE html>
<html>
<body>
<h2>File Upload Form</h2>
<form action="/upload" method="POST" enctype="multipart/form-data">
<label for="file">Choose a file:</label>
<input type="file" id="file" name="file"><br><br>
<button type="submit">Upload</button>
</form>
</body>
</html>
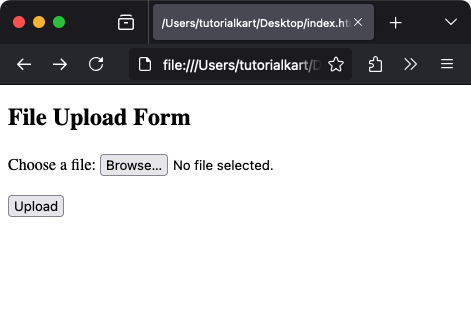
Explanation: This form allows users to upload a file, which is sent to the server at /upload
.
Styling the <form> Tag with CSS
Forms can be styled with CSS to improve appearance and layout. Here’s an example:
index.html
<!DOCTYPE html>
<html>
<head>
<style>
form {
background-color: #f9f9f9;
padding: 20px;
border: 1px solid #ccc;
border-radius: 5px;
max-width: 400px;
margin: auto;
}
label {
display: block;
margin-bottom: 10px;
font-weight: bold;
}
input {
width: 100%;
padding: 8px;
margin-bottom: 15px;
border: 1px solid #ccc;
border-radius: 4px;
}
button {
background-color: #007BFF;
color: white;
padding: 10px 15px;
border: none;
border-radius: 4px;
cursor: pointer;
}
button:hover {
background-color: #0056b3;
}
</style>
</head>
<body>
<h2>Styled Form</h2>
<form action="/submit-form" method="POST">
<label for="username">Username:</label>
<input type="text" id="username" name="username">
<label for="password">Password:</label>
<input type="password" id="password" name="password">
<button type="submit">Login</button>
</form>
</body>
</html>
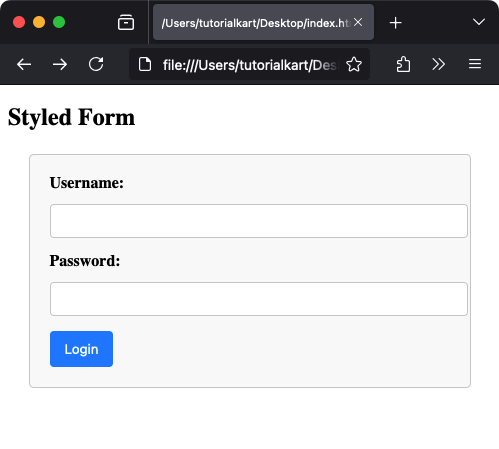
Result: The form has a styled container with padding, rounded corners, and responsive inputs for a professional look.
Practical Applications of the <form> Tag
- Login Forms: Collect user credentials like username and password.
- Registration Forms: Gather information from users to create accounts.
- Feedback Forms: Allow users to submit comments, suggestions, or inquiries.
- Search Forms: Collect search queries for processing by a search engine.
- File Uploads: Enable users to upload files, such as images or documents.