HTML Input Date
The HTML <input>
element with type="date"
is used to create a date picker input field that allows users to select a date from a calendar interface. This feature simplifies the process of entering date information and ensures uniformity in format, making it an essential tool for form inputs that require date data.
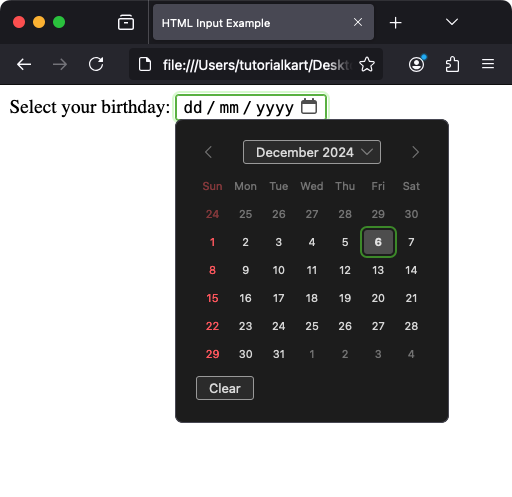
Unlike regular text inputs, <input type="date">
is optimized for date selection and provides a user-friendly interface. It supports attributes for validation and customization, ensuring accurate and meaningful data input.
In this tutorial, we will learn the syntax, attributes, styling, and practical applications of the Input Date.
1 Basic Syntax
The basic syntax for an HTML date input is:
<input type="date" name="birthday">
Here:
type="date"
: Specifies that the input is a date picker.name
: Associates the input with a specific name for form data submission.
2 Adding a Label for Accessibility
It’s a best practice to include a descriptive label for the date input to improve accessibility:
<label for="birthday">Select your birthday:</label>
<input type="date" id="birthday" name="birthday">
Clicking the label focuses the input, making the form more user-friendly.
3 Setting Default Values
You can set a default date for the input using the value
attribute. The value must be in the format YYYY-MM-DD
.
<input type="date" id="startdate" name="startdate" value="2024-01-01">
In this example, the default value is set to January 1, 2024. When the page loads, this date will be preselected.
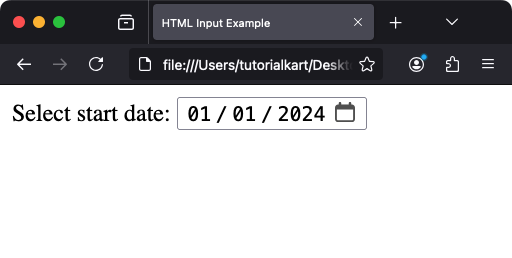
4 Setting Minimum and Maximum Dates
To restrict the date range users can select, use the min
and max
attributes:
<input type="date" id="appointment" name="appointment" min="2024-01-01" max="2024-12-31">
In this example, users can only select dates between January 1, 2024, and December 31, 2024.
5 Validation with Required Attribute
The required
attribute ensures that users cannot submit the form without selecting a date:
<input type="date" id="duedate" name="duedate" required>
When the user tries to submit the form without selecting a date, the browser displays an error message.
index.html
<form>
<label for="duedate">Due date:</label>
<input type="date" id="duedate" name="duedate" required>
<input type="submit" value="Submit">
</form>
Video
6 Styling the Date Input
Although the appearance of the date picker itself is controlled by the browser, you can style the input field using CSS:
<style>
input[type="date"] {
width: 90%;
padding: 10px;
border: 1px solid #ccc;
border-radius: 5px;
font-size: 16px;
}
input[type="date"]:focus {
border-color: #4CAF50;
outline: none;
box-shadow: 0 0 5px #4CAF50;
}
</style>
<label for="datefield">Pick a date:</label>
<input type="date" id="datefield">
This example styles the input field to have rounded corners and a green border when focused.
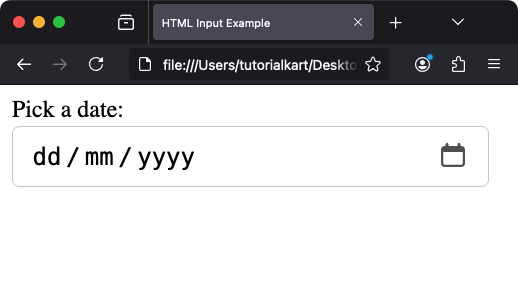
7 Using JavaScript with Date Input
JavaScript can be used to dynamically interact with the date input. For example, you can set the minimum date based on the current date:
<input type="date" id="dynamicDate" name="dynamicDate">
<script>
const today = new Date().toISOString().split('T')[0];
document.getElementById('dynamicDate').setAttribute('min', today);
</script>
This script sets the minimum selectable date to the current date, preventing users from selecting past dates.
8 Accessibility Considerations for Date Input
To ensure your date input is accessible:
- Provide clear and descriptive labels using the
<label>
element. - Use the
aria-label
oraria-describedby
attributes for additional instructions if needed. - Ensure sufficient color contrast for text and borders.
9 Real-World Use Cases for Date Input
- Booking Systems: Allow users to select dates for reservations or appointments.
- Event Registration: Collect event dates for scheduling.
- Deadline Management: Use date inputs for tasks or project deadlines.
Conclusion
The HTML <input type="date">
element is a powerful tool for creating user-friendly and accessible forms. By leveraging its attributes and integrating it with JavaScript, you can build interactive and efficient date inputs for various applications.