HTML <script> Tag
The HTML <script>
tag is used to embed or reference executable JavaScript code within an HTML document. It allows you to add interactivity, control page behavior, or dynamically update content. Scripts can either be included inline or referenced externally using the src
attribute.
The <script>
tag is a versatile element essential for adding dynamic features to webpages, such as form validation, animations, and event handling.
Basic Syntax of HTML <script> Tag
The basic structure of the <script>
tag is:
<script>
// JavaScript code goes here
</script>
To reference an external script file:
<script src="script.js"></script>
The src
attribute specifies the URL of the external JavaScript file.
Example of Inline JavaScript
Here’s an example of embedding JavaScript directly within a <script>
tag:
index.html
<!DOCTYPE html>
<html>
<body>
<h2>Hello World Example</h2>
<p id="demo"></p>
<script>
document.getElementById("demo").textContent = "Hello, World!";
</script>
</body>
</html>
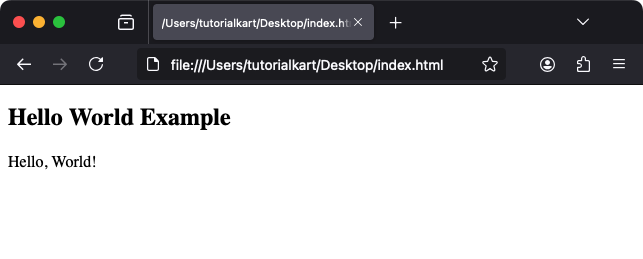
Explanation: The script dynamically updates the content of the paragraph with the ID “demo” to display “Hello, World!”
Example of External JavaScript
Here’s an example of referencing an external JavaScript file:
index.html
<!DOCTYPE html>
<html>
<body>
<h2>External Script Example</h2>
<p id="demo"></p>
<script src="script.js"></script>
</body>
</html>
script.js
document.getElementById("demo").textContent = "Hello from an external file!";
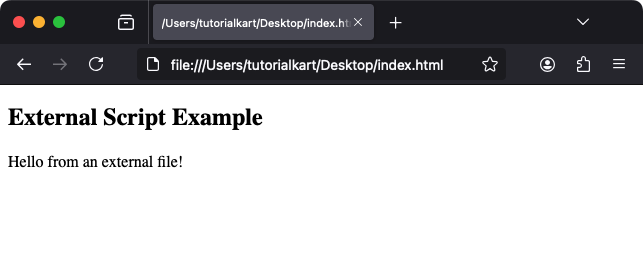
Explanation: The external file “script.js” updates the content of the paragraph with the ID “demo” to “Hello from an external file!”
Attributes of HTML <script> Tag
- src: Specifies the URL of an external JavaScript file.
- type: Specifies the MIME type of the script. The default is
text/javascript
. - async: Indicates that the script should be executed asynchronously (only for external scripts).
- defer: Specifies that the script should be executed after the document has been parsed (only for external scripts).
- crossorigin: Indicates how the script should be handled for cross-origin requests (e.g.,
anonymous
oruse-credentials
).
Difference Between async and defer
- async: The script is downloaded and executed as soon as possible, without blocking the HTML parsing.
- defer: The script is downloaded during HTML parsing but executed only after the document has been fully parsed.
Both attributes are applicable only to external scripts.
Styling and Placement of <script> Tag
Scripts can be placed in different parts of an HTML document:
- In the <head>: Use for scripts that must load before the page is fully rendered.
- At the bottom of the <body>: Preferred for scripts that enhance interactivity after the page is loaded.
<script>
// Example of inline script in the head
</script>
<script src="example.js" defer></script>
Best Practice: Use defer
for external scripts and place inline scripts at the bottom of the <body>
for better page load performance.
Practical Applications of the <script> Tag
- Form Validation: Validate user inputs dynamically without refreshing the page.
- Interactive Content: Add interactivity like animations, dropdown menus, or sliders.
- Dynamic Content: Fetch and display data using APIs (e.g., via AJAX or Fetch API).
- Event Handling: Respond to user actions such as clicks, hovers, or key presses.
- Analytics: Track user behavior with tools like Google Analytics.
The <script>
tag is fundamental for adding dynamic, interactive, and functional features to modern web applications.