HTML <table> Tag
The HTML <table>
tag is used to create tables in a webpage. Tables are used to organize data into rows and columns, providing a structured layout for displaying information. The <table>
element contains child elements such as <tr>
(table rows), <th>
(table headers), and <td>
(table data cells).
Basic Syntax of HTML <table> Tag
The basic structure of an HTML table is:
<table>
<tr>
<th>Header 1</th>
<th>Header 2</th>
</tr>
<tr>
<td>Data 1</td>
<td>Data 2</td>
</tr>
</table>
The <tr>
element defines a table row, <th>
defines a table header, and <td>
defines a table data cell.
Example of a Simple HTML Table
Here’s an example of a simple table displaying student scores:
index.html
<!DOCTYPE html>
<html>
<body>
<h2>Student Scores</h2>
<table border="1">
<tr>
<th>Name</th>
<th>Subject</th>
<th>Score</th>
</tr>
<tr>
<td>Alice</td>
<td>Math</td>
<td>85</td>
</tr>
<tr>
<td>Bob</td>
<td>Science</td>
<td>90</td>
</tr>
</table>
</body>
</html>
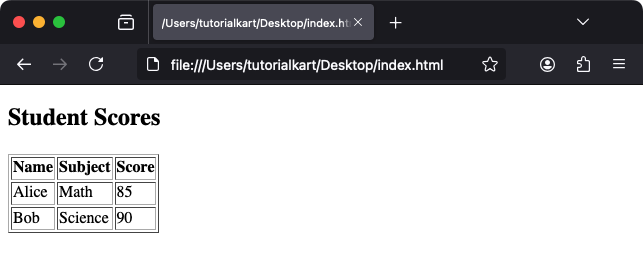
Explanation: The border="1"
attribute adds a simple border around the table for better visibility.
Adding Table Captions
The <caption>
element provides a title for the table:
index.html
<!DOCTYPE html>
<html>
<body>
<table border="1">
<caption>Monthly Sales Report</caption>
<tr>
<th>Product</th>
<th>Quantity</th>
<th>Price</th>
</tr>
<tr>
<td>Laptops</td>
<td>50</td>
<td>$500</td>
</tr>
</table>
</body>
</html>
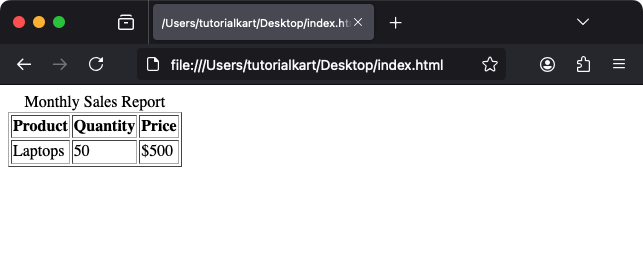
Explanation: The <caption>
tag provides a heading or summary for the table, which improves accessibility and usability.
Merging Cells in Table with colspan and rowspan
You can merge cells horizontally using colspan
and vertically using rowspan
:
index.html
<!DOCTYPE html>
<html>
<body>
<h2>Class Schedule</h2>
<table border="1">
<tr>
<th colspan="2">Monday</th>
</tr>
<tr>
<td>Math</td>
<td>9:00 - 10:00 AM</td>
</tr>
<tr>
<th rowspan="2">Tuesday</th>
<td>Science</td>
<td>10:00 - 11:00 AM</td>
</tr>
<tr>
<td>History</td>
<td>11:00 - 12:00 PM</td>
</tr>
</table>
</body>
</html>
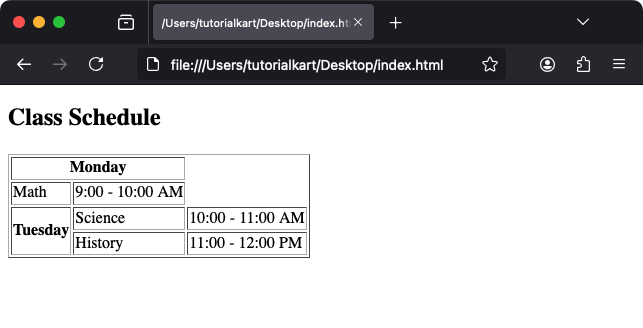
Explanation: The colspan
attribute merges columns, and the rowspan
attribute merges rows to create more complex table layouts.
Styling Tables with CSS
Tables can be styled to enhance their appearance using CSS:
index.html
<!DOCTYPE html>
<html>
<head>
<style>
table {
border-collapse: collapse;
width: 100%;
}
th, td {
border: 1px solid #ddd;
padding: 8px;
text-align: center;
}
th {
background-color: #f4f4f4;
font-weight: bold;
}
</style>
</head>
<body>
<h2>Styled Table</h2>
<table>
<tr>
<th>Name</th>
<th>Age</th>
<th>City</th>
</tr>
<tr>
<td>John</td>
<td>25</td>
<td>New York</td>
</tr>
</table>
</body>
</html>
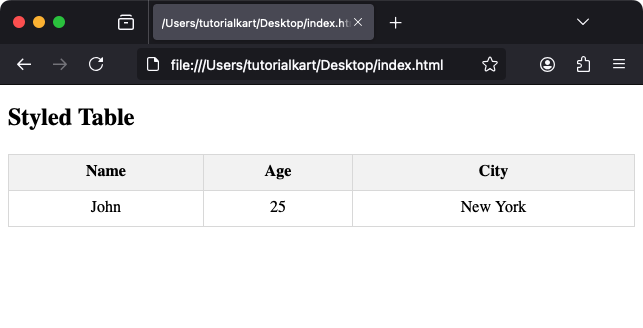
Explanation: The CSS styles enhance the table’s appearance by collapsing borders, adding padding, and styling headers differently.
Practical Applications of the <table> Tag
- Data Presentation: Display structured data such as reports, schedules, or scores.
- Financial Tables: Create tables for budgets, invoices, or expense tracking.
- Comparison Charts: Display side-by-side comparisons of products or services.
- Calendars: Build simple calendar layouts or date-specific schedules.