HTML Tables
HTML tables are essential for displaying data in a structured, tabular format, making it easier to present information like schedules, statistics, and comparative data.
The following figure illustrates the components of a table in HTML.
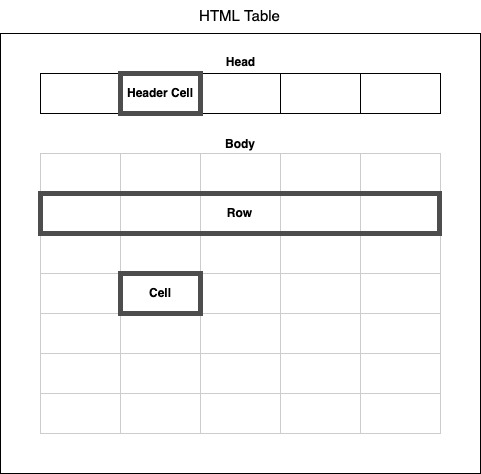
- Table contains Rows, and Columns.
- Each column can have a heading (Header Cell) which are grouped in Table Head.
- Each Row contains multiples Cells.
In this tutorial, we will cover the creation, styling, and best practices for using HTML tables effectively.
1 Understanding HTML Tables
An HTML table is defined using the <table>
element, which contains rows (<tr>
) and cells (<td>
for data cells and <th>
for header cells). This structure allows for organized data presentation in rows and columns.
1.1 Basic Table Structure
Here’s an example of a simple HTML table:
<table>
<tr>
<th>Name</th>
<th>Age</th>
<th>City</th>
</tr>
<tr>
<td>Alice</td>
<td>30</td>
<td>New York</td>
</tr>
<tr>
<td>Bob</td>
<td>25</td>
<td>Los Angeles</td>
</tr>
</table>
In this example:
<table>
defines the table.<tr>
defines a table row.<th>
defines a header cell.<td>
defines a standard data cell.
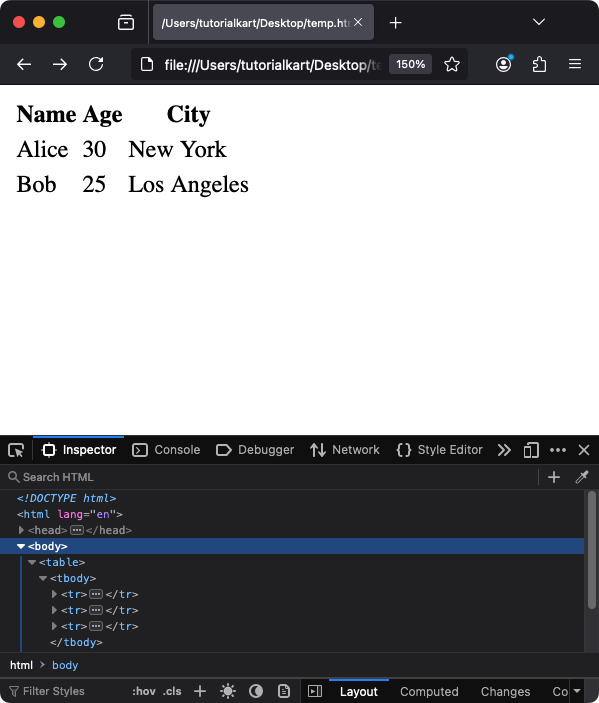
2 Table Headers
Table headers are created using the <th>
element and are typically used to label columns or rows, providing context for the data.
2.1 Column Headers
Column headers are placed within the first row of the table:
<tr>
<th>Name</th>
<th>Age</th>
<th>City</th>
</tr>
Here’s a complete example using the <tr>
, <th>
, and <td>
elements to create a simple table with a header row and a data row where the first cell in the data row uses <th>
as a row header:
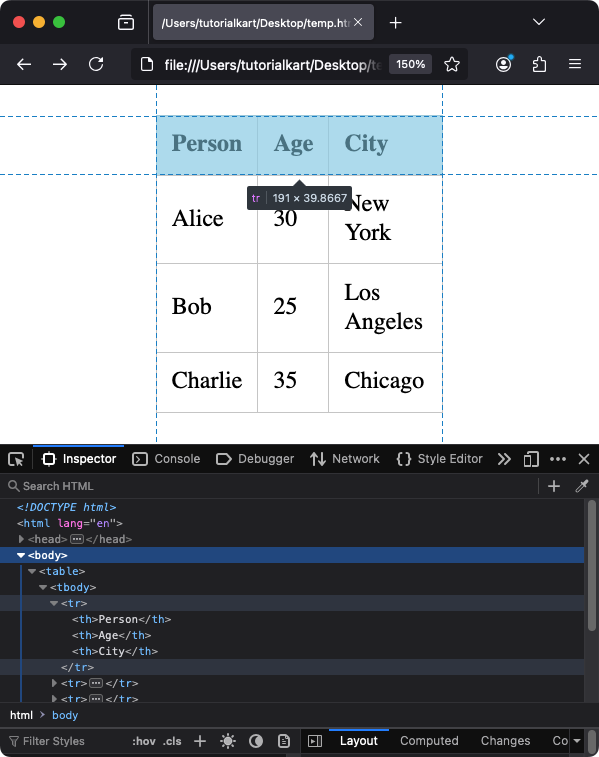
2.2 Row Headers
Row headers can be placed in the first cell of each row:
<tr>
<th>Alice</th>
<td>30</td>
<td>New York</td>
</tr>
Here’s a complete example using the <tr>
, <th>
, and <td>
elements to create a simple table with a header row and a data row where the first cell in the data row uses <th>
as a row header:
<style>
table {
width: 50%;
border-collapse: collapse;
margin: 20px auto;
}
th, td {
border: 1px solid #ccc;
padding: 10px;
text-align: left;
}
th {
background-color: #f4f4f4;
}
</style>
<table>
<thead>
<tr>
<th>Person</th>
<th>Age</th>
<th>City</th>
</tr>
</thead>
<tbody>
<tr>
<th>Alice</th>
<td>30</td>
<td>New York</td>
</tr>
<tr>
<th>Bob</th>
<td>25</td>
<td>Los Angeles</td>
</tr>
<tr>
<th>Charlie</th>
<td>35</td>
<td>Chicago</td>
</tr>
</tbody>
</table>
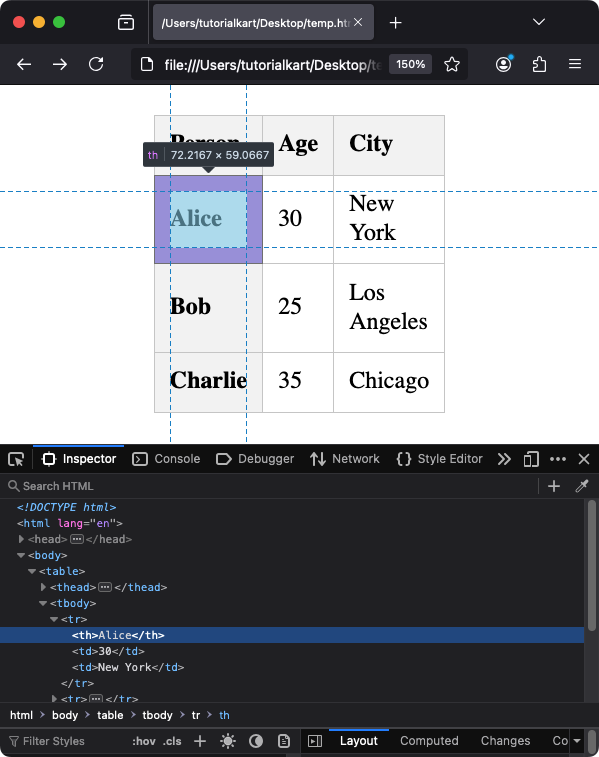
3 Spanning Rows and Columns
To merge cells across multiple rows or columns, use the rowspan
and colspan
attributes.
3.1 Column Spanning with colspan
The colspan
attribute allows a cell to span multiple columns:
<tr>
<td colspan="2">Merged Cell</td>
<td>Regular Cell</td>
</tr>
Here’s a complete example demonstrating the use of <td colspan="2">
to merge two columns within a row:
<table>
<thead>
<tr>
<th>Column 1</th>
<th>Column 2</th>
<th>Column 3</th>
</tr>
</thead>
<tbody>
<tr>
<td colspan="2">Merged Cell</td>
<td>Regular Cell</td>
</tr>
<tr>
<td>Data 1</td>
<td>Data 2</td>
<td>Data 3</td>
</tr>
</tbody>
</table>
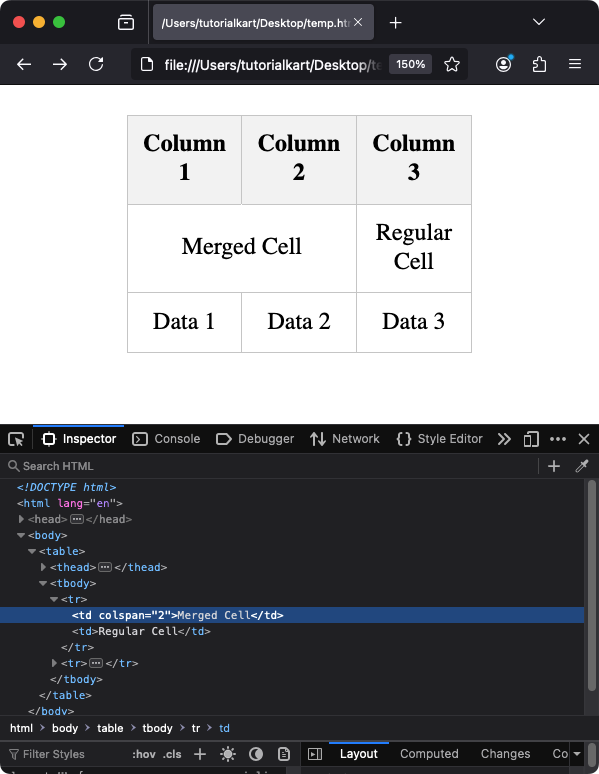
3.2 Row Spanning with rowspan
The rowspan
attribute allows a cell to span multiple rows:
<tr>
<td rowspan="2">Merged Cell</td>
<td>Regular Cell</td>
</tr>
<tr>
<td>Regular Cell</td>
</tr>
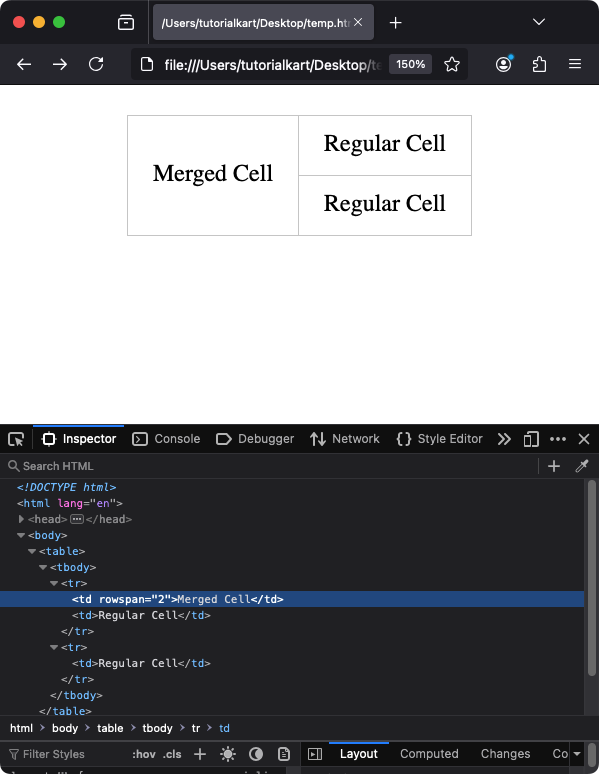
4 Grouping Table Content
HTML provides elements to group table content, enhancing readability and accessibility.
4.1 Table Head, Body, and Foot
Use the following elements to group table sections:
<thead>
: Groups the header content.<tbody>
: Groups the body content.<tfoot>
: Groups the footer content.
Example:
<table>
<thead>
<tr>
<th>Name</th>
<th>Age</th>
<th>City</th>
</tr>
</thead>
<tbody>
<tr>
<td>Alice</td>
<td>30</td>
<td>New York</td>
</tr>
<tr>
<td>Bob</td>
<td>25</td>
<td>Los Angeles</td>
</tr>
</tbody>
<tfoot>
<tr>
<td colspan="3">End of Table</td>
</tr>
</tfoot>
</table>

Using <thead>
, <tbody>
, and <tfoot>
helps organize the table structure, making it easier to style and manage.
5 Styling Tables
HTML tables can be styled using CSS to improve their appearance and usability. Below are some common CSS properties for table styling.
5.1 Adding Borders
table, th, td {
border: 1px solid black;
border-collapse: collapse;
}
This adds a solid border to the table, rows, and cells, and ensures the borders don’t overlap by collapsing them.
5.2 Adjusting Padding and Alignment
th, td {
padding: 10px;
text-align: center;
}
Padding adds space inside the cells, while text-align
centers the content.
5.3 Adding Row Colors
tr:nth-child(even) {
background-color: #f2f2f2;
}
tr:hover {
background-color: #ddd;
}
This CSS applies alternating row colors for better readability and highlights a row when hovered over.
6 Responsive Tables
Responsive tables adapt to different screen sizes. You can make tables scrollable on smaller screens by wrapping them in a container:
<div style="overflow-x: auto;">
<table>
<tr>
<th>Name</th>
<th>Age</th>
<th>City</th>
</tr>
<tr>
<td>Alice</td>
<td>30</td>
<td>New York</td>
</tr>
</table>
</div>
For more advanced responsiveness, use CSS frameworks like Bootstrap, which provide responsive table classes.
7 Accessible Tables
To make tables accessible to screen readers, use the following practices:
- Scope Attribute: Use the
scope
attribute in<th>
to specify if it applies to a row or column. - Caption: Add a
<caption>
to describe the table’s purpose.
<table>
<caption>Employee Data</caption>
<thead>
<tr>
<th scope="col">Name</th>
<th scope="col">Age</th>
<th scope="col">City</th>
</tr>
</thead>
</table>
HTML Table Tutorials
The following list of tutorials cover different use-cases specific to HTML tables.
- HTML Table Borders
- HTML Table Caption
- HTML Table Column Group
- HTML Table Column Span
- HTML Table Header for Multiple Columns
- HTML Table Row Span
- HTML Table Column Width
- HTML Table Cell Spacing
Conclusion
HTML tables are powerful tools for presenting data effectively. By using attributes, styling, and responsiveness, you can create tables that are visually appealing, accessible, and functional. Understanding the basics and applying advanced features will help you design better data presentations for your web projects.