HTML <td> Tag
The HTML <td>
tag defines a standard data cell in an HTML table. It is a child of the <tr>
(table row) element and is used to display data in rows and columns within a <table>
. By default, <td>
elements contain unformatted, left-aligned text unless styled otherwise.
The <td>
tag is a core component of HTML tables, alongside <th>
(header cells) and <tr>
.
Basic Syntax of HTML <td> Tag
The basic structure of a table with <td>
cells is:
<table>
<tr>
<td>Data 1</td>
<td>Data 2</td>
</tr>
<tr>
<td>Data 3</td>
<td>Data 4</td>
</tr>
</table>
Each <td>
represents one data cell in the table.
Example of a Simple Table with <td> Cells
Here’s an example of a table displaying student information:
index.html
<!DOCTYPE html>
<html>
<body>
<h2>Student Information</h2>
<table border="1">
<tr>
<th>Name</th>
<th>Age</th>
<th>Grade</th>
</tr>
<tr>
<td>Alice</td>
<td>14</td>
<td>A</td>
</tr>
<tr>
<td>Bob</td>
<td>15</td>
<td>B</td>
</tr>
</table>
</body>
</html>
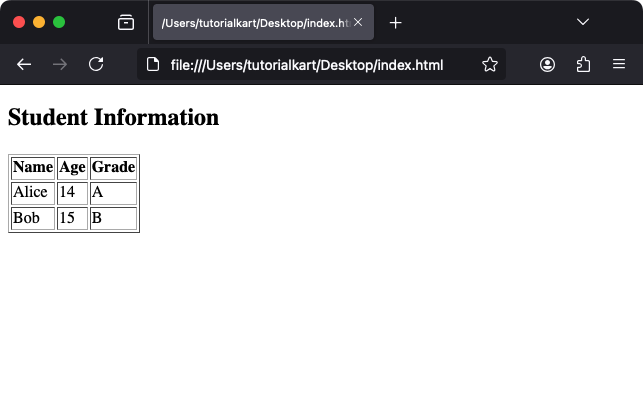
Explanation: Each <td>
cell contains individual pieces of data, organized into rows within the table.
Attributes of HTML <td> Tag
- colspan: Specifies the number of columns a cell should span.
- rowspan: Specifies the number of rows a cell should span.
- headers: Associates the cell with one or more
<th>
elements. - Global Attributes: Supports all global attributes, such as
id
,class
, andstyle
. - Event Attributes: Supports event attributes like
onclick
,onmouseover
, andonfocus
.
Merging Cells Using colspan and rowspan
The colspan
and rowspan
attributes allow you to merge cells:
index.html
<!DOCTYPE html>
<html>
<body>
<h2>Merged Cells Example</h2>
<table border="1">
<tr>
<th>Name</th>
<th>Details</th>
</tr>
<tr>
<td>Alice</td>
<td rowspan="2">Excellent student</td>
</tr>
<tr>
<td>Bob</td>
</tr>
</table>
</body>
</html>
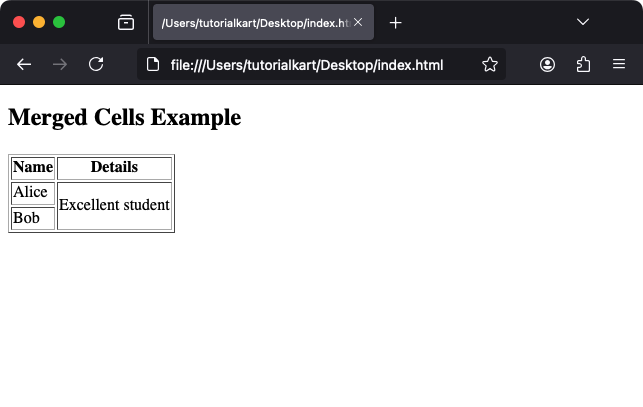
Explanation: The rowspan
attribute merges two rows, and the “Details” column spans multiple cells vertically.
Styling <td> Cells with CSS
Customize the appearance of <td>
cells using CSS:
index.html
<!DOCTYPE html>
<html>
<head>
<style>
table {
border-collapse: collapse;
width: 100%;
}
th, td {
border: 1px solid #ddd;
padding: 8px;
text-align: center;
}
td {
background-color: #f9f9f9;
}
td:hover {
background-color: #f1f1f1;
}
</style>
</head>
<body>
<h2>Styled Table</h2>
<table>
<tr>
<th>Name</th>
<th>Age</th>
</tr>
<tr>
<td>Alice</td>
<td>25</td>
</tr>
<tr>
<td>Bob</td>
<td>30</td>
</tr>
</table>
</body>
</html>
Result: The data cells have a light background color, padding, and hover effects to enhance readability and interactivity.
Practical Applications of the <td> Tag
- Data Representation: Use
<td>
cells to present structured data in rows and columns. - Dynamic Tables: Populate
<td>
cells dynamically with JavaScript or server-side data. - Custom Layouts: Create visually appealing tables by merging cells with
colspan
androwspan
. - Responsive Tables: Combine CSS and
<td>
elements to build responsive, mobile-friendly table layouts.