HTML <textarea> Tag
The HTML <textarea>
tag is used to define a multi-line input field where users can enter large amounts of text. It is commonly used in forms for comments, feedback, or any situation requiring extended user input. Unlike the <input>
tag with type="text"
, the <textarea>
tag allows text wrapping and provides more space for content.
The <textarea>
tag requires an opening and closing tag, and any text between them serves as default content.
Basic Syntax of HTML <textarea> Tag
The basic structure of a <textarea>
element is:
<textarea name="name" rows="rows" cols="cols">
Default text goes here.
</textarea>
The name
attribute specifies the name of the field, while rows
and cols
determine the visible size of the text area.
Example of a Simple <textarea>
Here’s an example of a basic <textarea>
for user feedback:
index.html
<!DOCTYPE html>
<html>
<body>
<h2>Feedback Form</h2>
<form>
<label for="feedback">Your Feedback:</label><br>
<textarea id="feedback" name="feedback" rows="4" cols="50">
Enter your feedback here...
</textarea><br><br>
<button type="submit">Submit</button>
</form>
</body>
</html>
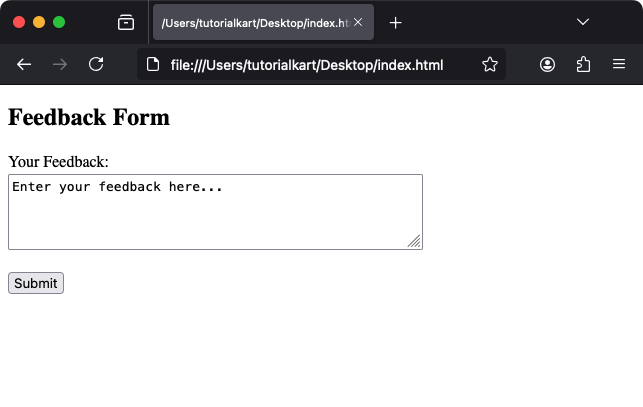
Explanation: The <textarea>
provides a multi-line input field with 4 rows and 50 columns, containing placeholder text by default.
Attributes of HTML <textarea> Tag
- name: Specifies the name of the text area, used for form submission.
- rows: Defines the number of visible text lines.
- cols: Defines the width of the text area in characters.
- placeholder: Displays placeholder text when the text area is empty.
- maxlength: Specifies the maximum number of characters allowed.
- required: Ensures the text area must be filled before form submission.
- readonly: Makes the text area non-editable.
- disabled: Disables the text area.
- Global Attributes: Supports all global attributes like
id
,class
, andstyle
.
Example with Additional Attributes
The following example includes various attributes for better control:
index.html
<!DOCTYPE html>
<html>
<body>
<h2>Contact Us</h2>
<form>
<label for="message">Message:</label><br>
<textarea id="message" name="message" rows="6" cols="40" placeholder="Enter your message here..." maxlength="500" required></textarea><br><br>
<button type="submit">Send</button>
</form>
</body>
</html>
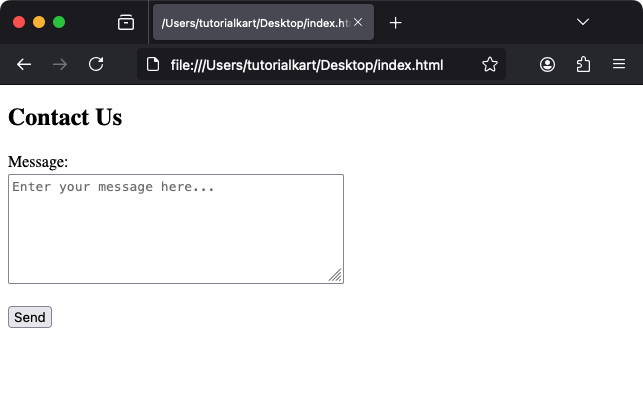
Explanation: This example uses the placeholder
, maxlength
, and required
attributes to enhance functionality.
Styling the <textarea> Tag with CSS
The <textarea>
element can be styled with CSS to improve its appearance:
index.html
<!DOCTYPE html>
<html>
<head>
<style>
textarea {
width: calc(100% - 20px);
height: 150px;
padding: 10px;
font-family: Arial, sans-serif;
font-size: 14px;
border: 1px solid #ccc;
border-radius: 5px;
resize: vertical;
}
</style>
</head>
<body>
<h2>Styled Textarea</h2>
<textarea placeholder="Type your text here..."></textarea>
</body>
</html>
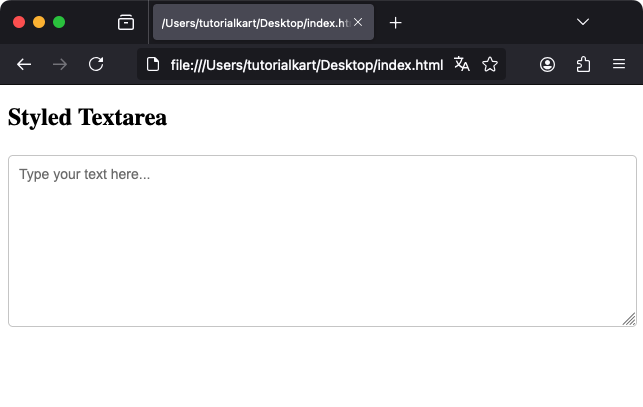
Result: The text area is styled with rounded corners, padding, and a resizable height for better usability.
Practical Applications of the <textarea> Tag
- Feedback Forms: Allow users to submit detailed feedback or comments.
- Messaging Systems: Create input fields for composing messages or emails.
- Code Editors: Use
<textarea>
for writing and editing code snippets. - Dynamic Content: Populate and manipulate text areas dynamically with JavaScript for interactive web applications.