HTML <th> Tag
The HTML <th>
tag is used to define header cells in an HTML table. These cells are typically located at the top of each column or at the beginning of each row to provide contextual information about the data in the table. By default, text inside a <th>
element is bold and center-aligned.
The <th>
tag enhances table semantics and accessibility, especially when paired with the scope
attribute, which associates the header with specific rows or columns.
Basic Syntax of HTML <th> Tag
The basic structure of a table with header cells is:
<table>
<tr>
<th>Header 1</th>
<th>Header 2</th>
<th>Header 3</th>
</tr>
<tr>
<td>Data 1</td>
<td>Data 2</td>
<td>Data 3</td>
</tr>
</table>
The <th>
cells act as headers for their respective columns.
Example of a Table with <th> Cells
Here’s an example of a table displaying employee information with headers:
index.html
<!DOCTYPE html>
<html>
<body>
<h2>Employee Data</h2>
<table border="1">
<tr>
<th>Name</th>
<th>Department</th>
<th>Salary</th>
</tr>
<tr>
<td>Alice</td>
<td>Engineering</td>
<td>$100,000</td>
</tr>
<tr>
<td>Bob</td>
<td>Marketing</td>
<td>$90,000</td>
</tr>
</table>
</body>
</html>
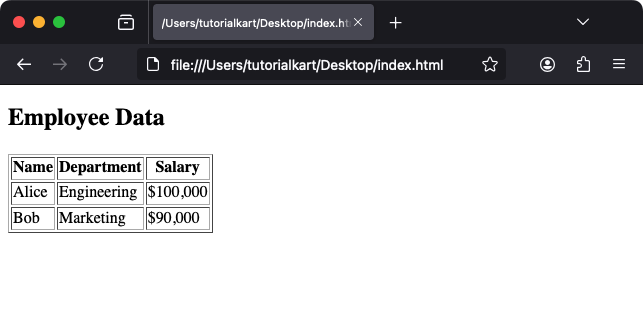
Explanation: The <th>
elements provide headers for the “Name,” “Department,” and “Salary” columns.
Attributes of HTML <th> Tag
- colspan: Specifies the number of columns the header cell should span.
- rowspan: Specifies the number of rows the header cell should span.
- scope: Defines whether the header applies to a row, column, or group of rows/columns (values:
col
,row
,colgroup
,rowgroup
). - abbr: Provides an abbreviated version of the header text for accessibility.
- Global Attributes: Supports all global attributes like
id
,class
, andstyle
. - Event Attributes: Supports event attributes such as
onclick
,onmouseover
, andonfocus
.
Example with colspan, rowspan, and scope
The following example uses advanced attributes like colspan
, rowspan
, and scope
to create a more complex table:
index.html
<!DOCTYPE html>
<html>
<body>
<h2>Sales Report</h2>
<table border="1">
<tr>
<th colspan="2" scope="colgroup">Product</th>
<th rowspan="2" scope="col">Total Sales</th>
</tr>
<tr>
<th scope="col">Name</th>
<th scope="col">Category</th>
</tr>
<tr>
<td>Laptop</td>
<td>Electronics</td>
<td>$10,000</td>
</tr>
<tr>
<td>Chair</td>
<td>Furniture</td>
<td>$5,000</td>
</tr>
</table>
</body>
</html>
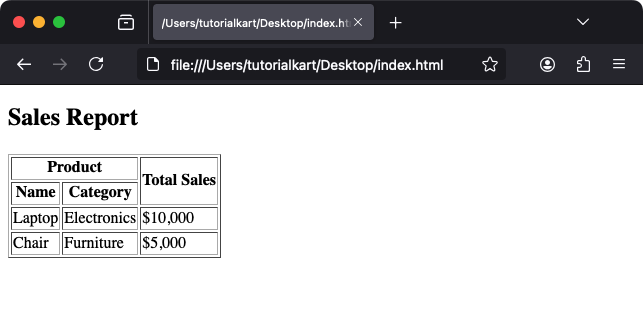
Explanation: The colspan
attribute spans two columns for the “Product” header, and the rowspan
attribute spans two rows for the “Total Sales” header. The scope
attribute improves accessibility by specifying which cells the headers apply to.
Styling <th> Cells with CSS
Customize the appearance of header cells using CSS:
index.html
<!DOCTYPE html>
<html>
<head>
<style>
table {
border-collapse: collapse;
width: 100%;
}
th, td {
border: 1px solid #ddd;
padding: 8px;
}
th {
background-color: #f4f4f4;
font-weight: bold;
text-align: center;
}
</style>
</head>
<body>
<h2>Styled Table Headers</h2>
<table>
<tr>
<th>Name</th>
<th>Age</th>
<th>City</th>
</tr>
<tr>
<td>Alice</td>
<td>25</td>
<td>New York</td>
</tr>
<tr>
<td>Bob</td>
<td>30</td>
<td>Los Angeles</td>
</tr>
</table>
</body>
</html>
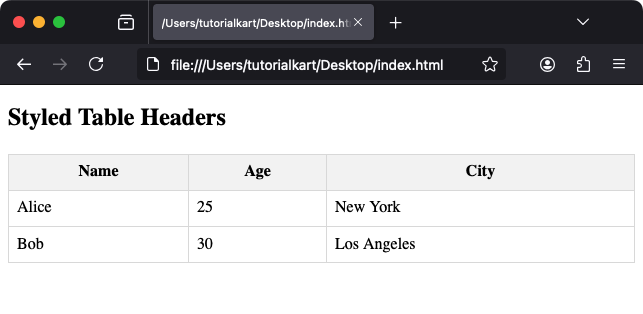
Result: The table headers are styled with a light background and bold text for better visibility and differentiation.
Practical Applications of the <th> Tag
- Data Tables: Use
<th>
to label columns and rows, providing context for tabular data. - Accessible Tables: Enhance screen reader navigation by using
scope
andabbr
attributes. - Styled Headers: Differentiate headers visually to improve table readability and usability.
- Complex Tables: Combine
colspan
androwspan
for multi-level headers in advanced layouts.