HTML <tr> Tag
The HTML <tr>
tag is used to define a row in an HTML table. Each row in a table is created using the <tr>
element, which acts as a container for table cells (<td>
for data and <th>
for headers). The <tr>
tag is a fundamental building block of HTML tables, grouping cells horizontally to form rows.
The <tr>
tag is a child of the <table>
, <thead>
, <tbody>
, or <tfoot>
elements.
Basic Syntax of HTML <tr> Tag
The basic structure of a table row using the <tr>
tag is:
<table>
<tr>
<td>Cell 1</td>
<td>Cell 2</td>
</tr>
</table>
Each <tr>
element represents a single row containing one or more cells.
Example of a Table with Multiple Rows
Here’s an example of a table displaying a list of students and their grades, organized into rows:
index.html
<!DOCTYPE html>
<html>
<body>
<h2>Student Grades</h2>
<table border="1">
<tr>
<th>Name</th>
<th>Subject</th>
<th>Grade</th>
</tr>
<tr>
<td>Alice</td>
<td>Math</td>
<td>A</td>
</tr>
<tr>
<td>Bob</td>
<td>Science</td>
<td>B</td>
</tr>
</table>
</body>
</html>
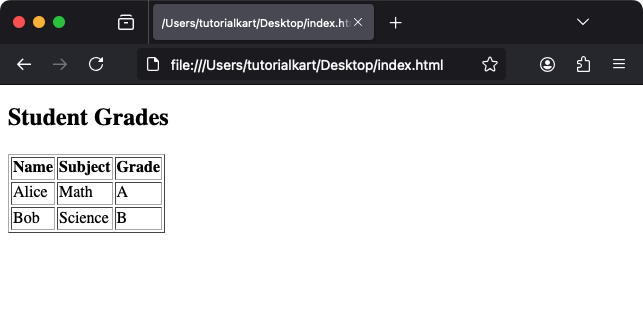
Explanation: The table contains three rows: one header row (<th>
cells inside a <tr>
) and two data rows (<td>
cells inside <tr>
s).
Styling Table Rows with CSS
You can style rows in a table to improve its appearance and usability. For example, alternating row colors can enhance readability:
index.html
<!DOCTYPE html>
<html>
<head>
<style>
table {
border-collapse: collapse;
width: 100%;
}
th, td {
border: 1px solid #ddd;
padding: 8px;
text-align: left;
}
tr:nth-child(even) {
background-color: #f9f9f9;
}
tr:hover {
background-color: #f1f1f1;
}
</style>
</head>
<body>
<h2>Styled Table Rows</h2>
<table>
<tr>
<th>Name</th>
<th>Subject</th>
<th>Grade</th>
</tr>
<tr>
<td>Alice</td>
<td>Math</td>
<td>A</td>
</tr>
<tr>
<td>Bob</td>
<td>Science</td>
<td>B</td>
</tr>
</table>
</body>
</html>
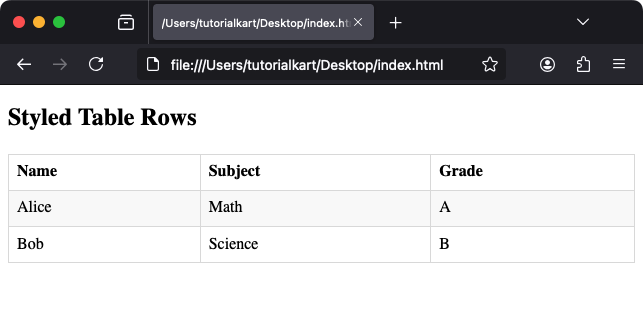
Explanation: The tr:nth-child(even)
selector applies a light background color to even-numbered rows, while tr:hover
highlights the row when hovered over.
Attributes of HTML <tr> Tag
- Global Attributes: Supports all global attributes, such as
id
,class
, andstyle
. - Event Attributes: Allows event handlers such as
onclick
,onmouseover
, andonmouseout
to add interactivity.
Example of Using Event Attributes with <tr>
Here’s an example where the <tr>
tag uses event attributes to provide interactivity:
index.html
<!DOCTYPE html>
<html>
<body>
<h2>Interactive Table Rows</h2>
<table border="1">
<tr onclick="alert('You clicked on row 1!')">
<td>Row 1, Cell 1</td>
<td>Row 1, Cell 2</td>
</tr>
<tr onclick="alert('You clicked on row 2!')">
<td>Row 2, Cell 1</td>
<td>Row 2, Cell 2</td>
</tr>
</table>
</body>
</html>
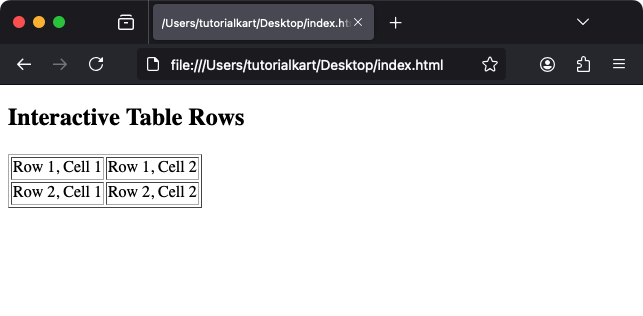
Explanation: Clicking a row triggers an alert with a custom message. This functionality is added using the onclick
attribute.
Practical Applications of the <tr> Tag
- Data Tables: Use
<tr>
to define rows in tabular data for reports, inventories, and comparisons. - Dynamic Rows: Add or remove rows dynamically using JavaScript to create interactive data tables.
- Styling: Style rows independently for improved readability and usability.
- Interactivity: Implement interactive functionalities such as row selection or click-based events.