JavaScript – Get HTML Text of an Element
To get the HTML text of an Element, using JavaScript, get reference to this HTML element, and read the outerHTML
property of this HTML Element.
outerHTML
property returns the HTML markup of this HTML Element as String.
In the following example, we will get the HTML text of an Element, which is selected by id "myElement"
and print it to console.
example.html
</>
Copy
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<style>
#myElement{
border: 1px solid green;
display: inline-block;
padding: 5px;
}
</style>
</head>
<body>
<h2>Get HTML text of Element using JavaScript</h2>
<div id="myElement">
<p>HTML Element Part 1.</p>
<p>HTML Element Part 2.</p>
</div>
<br><br>
<button type="button" onclick="execute()">Click Me</button>
<script>
function execute(){
var element = document.getElementById('myElement');
var result = element.outerHTML;
console.log(result);
}
</script>
</body>
</html>
Try this html file online, and click on the Click Me
button. The script gets the HTML text inside the Element #myElement
, and prints it to console as shown in the following screenshot.
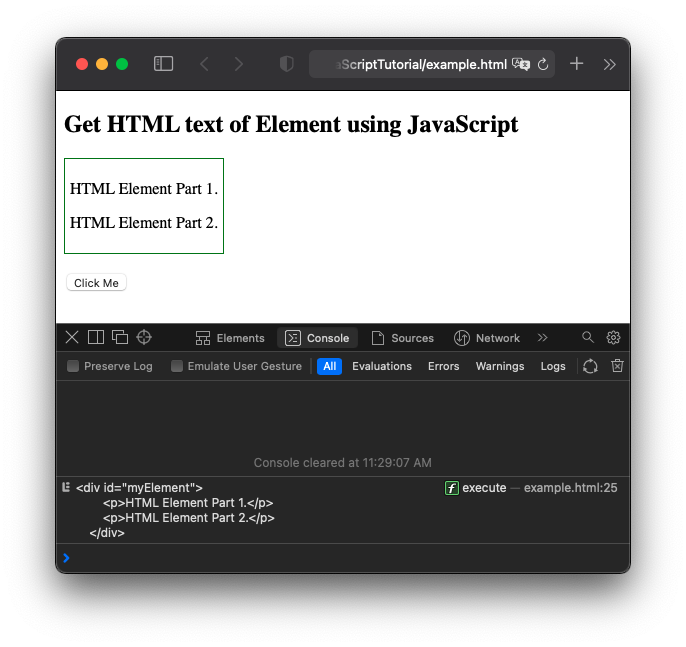
Conclusion
In this JavaScript Tutorial, we learned how to get the HTML text inside an Element, using JavaScript.