JSON Arrays
A JSON array is an ordered collection of values. It can hold multiple types of data, including:
- Strings
- Numbers
- Booleans
- Objects
- Another Array (nested arrays)
- Null
JSON arrays are enclosed in square brackets []
, and the values inside are separated by commas.
["apple", "banana", "cherry"]
The following diagram highlights the composition of a JSON array.
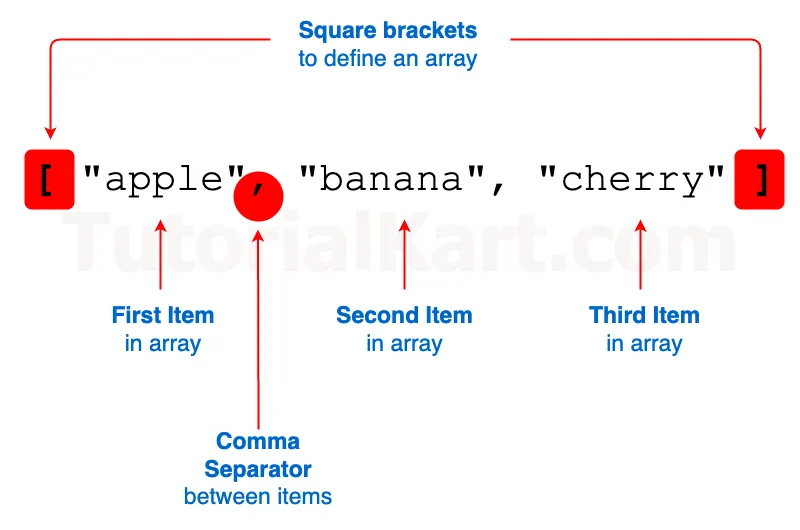
Syntax Rules for JSON Arrays
- Square Brackets: Arrays must begin and end with square brackets (
[]
). - Comma Separation: Each value in the array is separated by a comma.
- Allowable Types: An array can contain any valid JSON data type.
- Mixed Types: Arrays can hold mixed types of data, such as strings, numbers, and objects.
Examples of JSON Arrays
1 Simple Array of Strings:
{
"fruits": ["apple", "banana", "cherry"]
}
2 Array of Numbers:
{
"scores": [95, 88, 76, 100]
}
3 Array with Mixed Data Types:
{
"data": ["text", 42, true, null]
}
4 Array of Objects:
{
"students": [
{ "name": "Alice", "age": 22 },
{ "name": "Bob", "age": 24 }
]
}
5 Nested Arrays:
{
"matrix": [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
]
}
Common Use Cases for JSON Arrays
- Storing Lists: JSON arrays are ideal for storing lists of items, such as product names or categories.
- Structured Data: Arrays of objects can be used to represent structured data, like user profiles or database records.
- Nested Data: Arrays allow nesting for hierarchical or matrix-like data, such as a table or grid.
Tips for Working with JSON Arrays
- Ensure proper syntax by validating JSON using online tools like JSONLint.
- Keep array values consistent when possible (e.g., avoid mixing unrelated data types).
- Use arrays to group related data for easier parsing and manipulation in programming languages.
FAQs for JSON Arrays
1. What is a JSON Array?
A JSON array is an ordered collection of values, enclosed in square brackets ([]
), that can hold multiple data types such as strings, numbers, booleans, objects, other arrays, or null values.
2. Can a JSON Array contain different data types?
Yes, a JSON array can contain mixed data types, such as strings, numbers, booleans, and even nested objects or arrays.
3. How are values separated in a JSON Array?
Values in a JSON array are separated by commas. The last value in the array should not have a trailing comma.
4. Can JSON Arrays contain other arrays?
Yes, JSON arrays can contain nested arrays. This is useful for representing hierarchical or matrix-like data.
5. Are JSON Arrays zero-indexed?
Yes, when accessing JSON arrays in most programming languages, they are zero-indexed, meaning the first element has an index of 0
.
6. What are some common use cases for JSON Arrays?
JSON arrays are commonly used to store lists of data, such as a collection of items, user profiles, or hierarchical data like organisational structures.