JSON Numbers
A JSON number represents numeric data and can be integers or floating-point numbers.
Integers are whole numbers (e.g., 1, 100, -50).
Floating-Point Numbers are numbers with decimal points (e.g., 3.14, -0.5, 1.0).
Example:
Consider the following JSON object, where we have two properties: age
and height
.
{
"age": 25,
"height": 5.8
}
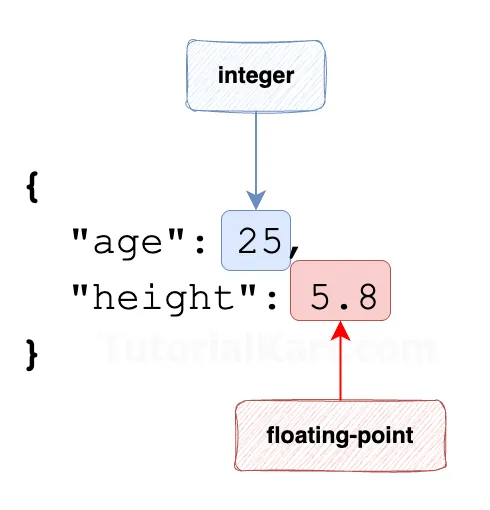
In this example, the property "age"
is assigned with 25
, an integer value, and the property "height"
is assigned with 5.8
, a floating-point value.
Syntax Rules for JSON Numbers
- No Quotes: Numbers in JSON are written without quotes.
- ✅ Correct:
"price": 99.99
- ❌ Incorrect value for a number:
"price": "99.99"
. In this case, the value is of data type string, but not a number.
- ✅ Correct:
- Decimal Representation: Use a period (
.
) as the decimal separator. Commas are not allowed.- ✅ Correct:
"value": 3.14
- ❌ Incorrect:
"value": 3,14
- ✅ Correct:
- Positive and Negative Numbers:
- Positive numbers can be written with or without the
+
sign (e.g.,5
or+5
). - Negative numbers must start with a
-
sign (e.g.,-10
).
- Positive numbers can be written with or without the
- No Leading Zeros: Numbers should not have leading zeros unless they are after the decimal point.
- ✅ Correct:
"value": 42
- ❌ Incorrect:
"value": 042
- ✅ Correct:
FAQs
1. What are JSON Numbers used for?
JSON numbers are used to represent numeric data like age, price, measurements, or any other quantitative values in JSON objects.
2. Can JSON Numbers have quotes?
No, JSON numbers must not have quotes. Writing numbers with quotes will make them strings, not numbers.
3. What types of numbers are supported in JSON?
JSON supports:
- Integers (e.g.,
10
,-42
) - Floating-point numbers (e.g.,
3.14
,-0.5
)
4. Can JSON Numbers be negative?
Yes, JSON numbers can be negative. A minus sign (-
) is used to represent negative numbers.
5. Does JSON allow numbers with leading zeros?
No, JSON numbers cannot have leading zeros. For example, 042
is invalid, but 42
is valid.
6. What is the decimal separator for JSON Numbers?
JSON uses a period (.
) as the decimal separator. Commas are not allowed.
7. Can JSON Numbers include special formats like exponential notation?
Yes, JSON numbers can use exponential notation. For example, 1.2e3
represents 1200
.
8. What happens if a number exceeds the range of supported values?
If a number exceeds the range of supported values (e.g., too large or too small), it may cause parsing errors or undefined behaviour, depending on the implementation.