JSON Objects
A JSON object is a collection of key-value pairs, enclosed in curly braces {}
. Each key is a string, and each value can be any valid JSON data type, including:
Syntax Rules for JSON Objects
- Curly Braces: JSON objects must begin and end with curly braces
{}
. - Key-Value Pairs: Each key-value pair is separated by a colon (
:
). - Comma Separation: Multiple key-value pairs are separated by commas (
,
). - Keys as Strings: Keys must always be enclosed in double quotes (
""
). - No Trailing Comma: The last key-value pair should not have a trailing comma.
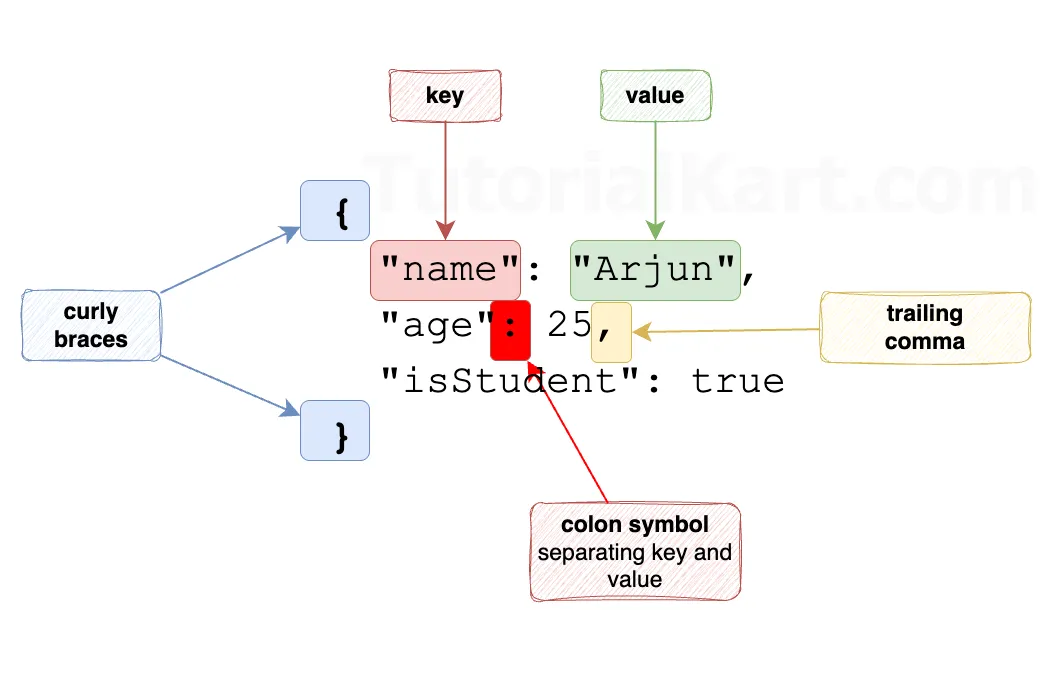
Examples of JSON Objects
1 Simple JSON Object:
{
"name": "Arjun",
"age": 25,
"isStudent": true
}
2 JSON Object with Nested Objects:
{
"person": {
"firstName": "Arjun",
"lastName": "Bheem",
"address": {
"street": "123 Main St",
"city": "New York",
"zip": "10001"
}
}
}
3 JSON Object with Arrays:
{
"product": "Laptop",
"price": 999.99,
"features": ["Lightweight", "Touchscreen", "Long Battery Life"]
}
4 JSON Object with Mixed Data Types:
{
"id": 123,
"name": "Gadget",
"available": true,
"specifications": {
"color": "black",
"weight": "1.2kg"
},
"tags": ["electronics", "portable"]
}
Common Use Cases for JSON Objects
- Storing User Data: Represent user profiles, preferences, and settings.
- API Responses: Return structured data from web APIs.
- Configuration Files: Store settings and parameters for applications.
- Nested Data Structures: Represent hierarchical or relational data.
FAQs for JSON Objects
1. What is a JSON Object?
A JSON object is a collection of key-value pairs enclosed in curly braces ({}
). Each key must be a string, and the value can be any valid JSON data type, such as a string, number, boolean, array, object, or null.
2. Can JSON Objects contain other JSON Objects?
Yes, JSON objects can be nested, meaning they can contain other JSON objects as values.
3. Are keys in a JSON Object case-sensitive?
Yes, keys in a JSON object are case-sensitive. For example, "Name"
and "name"
would be treated as two different keys.
4. Can keys in a JSON Object have spaces?
Yes, keys can have spaces, but they must be enclosed in double quotes. For example:
{
"full name": "Arjun"
}
5. What happens if there are duplicate keys in a JSON Object?
If there are duplicate keys in a JSON object, the value of the last occurrence of the key will overwrite the previous ones.
6. How are JSON Objects used in APIs?
JSON objects are widely used in APIs to represent structured data, such as user profiles, configurations, or responses from a server.
7. Can I include comments in JSON Objects?
No, JSON does not support comments. If you need to document your JSON, use external documentation or keep the comments in your code.