JUnit – Introduction and Getting Started
This tutorial introduces JUnit, the basic concepts of JUnit, its features, and how to get started with creating and running tests using the framework.
Roadmap to learn JUnit
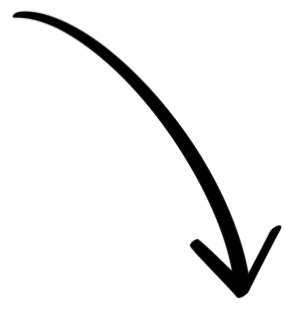
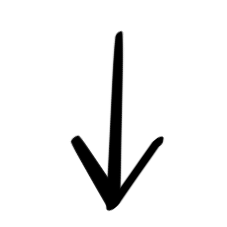
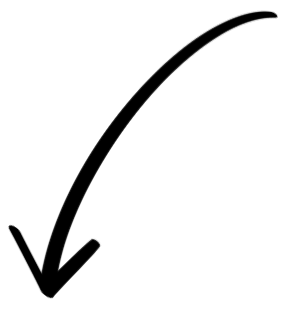
What is JUnit?
JUnit is a Java-based testing framework that supports test-driven development (TDD). It allows developers to write and execute test cases for individual units of code, such as methods or classes. JUnit follows the principle of “write once, test often,” making it a core part of modern software development practices.
JUnit tests are simple Java methods annotated with specific annotations to define test behavior.
This JUnit framework includes features like assertions, annotations, test runners, and integration with build tools like Maven and Gradle.
Reference: To learn about Java programming, please refer Java Tutorials.
Key Features of JUnit
- Annotations: Simplify the definition and management of test cases (e.g.,
@Test
,@BeforeEach
). - Assertions: Provide methods to verify test results (e.g.,
assertEquals
,assertTrue
). - Test Suites: Group multiple test cases for execution.
- Integration: Works seamlessly with build tools (Maven, Gradle) and IDEs (Eclipse, IntelliJ IDEA).
- Support for Testing Lifecycle: Includes setup and teardown methods for test preparation and cleanup.
Setting Up JUnit
To start using JUnit, you need to set it up in your project. Here’s how to configure JUnit using Maven and Gradle:
1 Setting Up with Maven
Add the following dependency to your pom.xml
file:
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter</artifactId>
<version>5.9.2</version>
<scope>test</scope>
</dependency>
2 Setting Up with Gradle
Add the following dependency to your build.gradle
file:
testImplementation 'org.junit.jupiter:junit-jupiter:5.9.2'
Basic Structure of a JUnit Test
A JUnit test is a Java class with test methods annotated using @Test
. These methods contain assertions to validate the behavior of the code being tested. Below is an example of a simple test case:
import static org.junit.jupiter.api.Assertions.*;
import org.junit.jupiter.api.Test;
class CalculatorTest {
@Test
void additionShouldReturnCorrectSum() {
Calculator calculator = new Calculator();
int result = calculator.add(2, 3);
assertEquals(5, result, "2 + 3 should equal 5");
}
}
In this example:
assertEquals(5, result)
: Validates that the result ofcalculator.add(2, 3)
is 5.@Test
: Marks the method as a test case.
Annotations in JUnit
JUnit provides a rich set of annotations to manage test cases and their lifecycle:
@Test
: Marks a method as a test case.@BeforeEach
: Runs before each test method to set up test data.@AfterEach
: Runs after each test method to clean up resources.@BeforeAll
: Runs once before all tests (requires static methods).@AfterAll
: Runs once after all tests (requires static methods).@Disabled
: Disables a test method temporarily.
Example of using lifecycle annotations:
import org.junit.jupiter.api.*;
class ExampleTest {
@BeforeEach
void setUp() {
System.out.println("Setting up...");
}
@Test
void sampleTest() {
System.out.println("Running test...");
assertTrue(1 + 1 == 2);
}
@AfterEach
void tearDown() {
System.out.println("Cleaning up...");
}
}
Assertions in JUnit
Assertions are the core of JUnit testing, providing methods to validate expected results. Commonly used assertions include:
assertEquals(expected, actual)
: Checks if two values are equal.assertTrue(condition)
: Verifies that a condition is true.assertFalse(condition)
: Verifies that a condition is false.assertNull(object)
: Checks if an object is null.assertNotNull(object)
: Checks if an object is not null.assertThrows(exceptionClass, executable)
: Validates that a specific exception is thrown.
Example:
import static org.junit.jupiter.api.Assertions.*;
import org.junit.jupiter.api.Test;
class AssertionTest {
@Test
void testAssertions() {
String str = "JUnit";
String nullStr = null;
assertEquals("JUnit", str);
assertNotNull(str);
assertNull(nullStr);
assertThrows(ArithmeticException.class, () -> { int result = 1 / 0; });
}
}
Running JUnit Tests
JUnit tests can be run using:
- IDE: Most IDEs like IntelliJ IDEA and Eclipse have built-in support for running JUnit tests.
- Command Line: Use build tools like Maven (
mvn test
) or Gradle (gradle test
). - Continuous Integration (CI): Integrate tests into CI pipelines using tools like Jenkins or GitHub Actions.
Conclusion
JUnit framework is used for testing Java applications. By incorporating JUnit into your development workflow, you can ensure higher code quality, reduce bugs, and maintain robust applications. This introductory tutorial provides a foundation for writing and managing tests effectively, and there is much more to explore as you dive deeper into JUnit’s features.