Apex Array
Apex Arrays are the collection of similar elements, where the memory is allocated sequently. Each element in the array is located by index and the index value starts with zero.
Array Syntax
</>
Copy
<String> [] arrayOfProducts = new List<String>();
Dynamic declaration
</>
Copy
Datatype[] arrayname =new DataType[size];
Static declaration
</>
Copy
DataType[] arrayname =new DataType[] {20,40};
Difference between Array and Collections
Arrays | Collections |
Array is a collection of similar elements. | It is a collections of homogeneous and heterogeneous elements. |
Arrays can not grow and shrink dynamically. | Collections can grow and shrink dynamically. |
Arrays can be accessed faster and consume less memory. | Collections are slow when compared with arrays and they consume more memory. |
Let us understand the working of an Arrays in Apex by writing a simple program to display Array of strings in PageBlockTable. To create an Apex class in Salesforce, login to Salesforce -> Developer console -> File -> File -> New -> Apex class.
- Enter the Apex class name to create new Apex Class.
Apex Class code
</>
Copy
Public class ArrayExample {
Public String[] myval{set;get;}
Public String name{get;set;}
Public ArrayExample() {
name = 'Prasanth';
myval = new String[] {'Malli','Adarsh','kiran'};
}
}
Visualforce Page
When referring Apex class variables in the Visualforce page, we should use getter and setter method.
</>
Copy
<apex:page controller="ArrayExample" >
<apex:form>
<apex:pageBlock>
<apex:pageBlockTable value="{!myval}" var="a">
<apex:column value="{!a}"/>
</apex:pageBlockTable>
<apex:outputLabel>{!name}</apex:outputLabel>
</apex:pageBlock>
</apex:form>
</apex:page>
Output
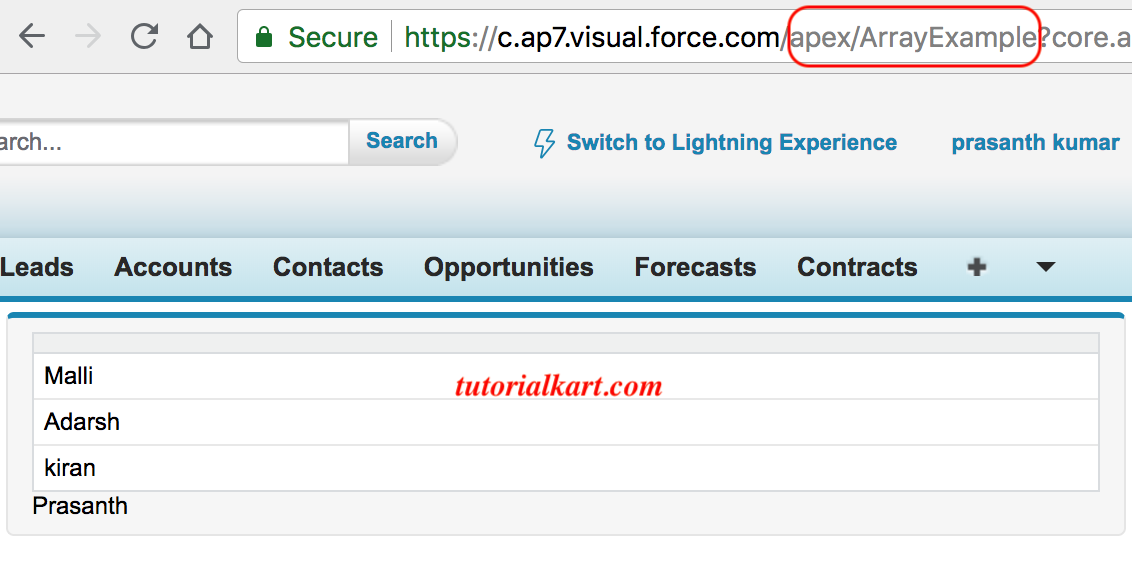