Phonetic Matching in MySQL
Phonetic matching allows you to find strings that sound similar to each other, which can be useful when searching for names or words with slight spelling differences.
In MySQL, the SOUNDEX()
function can be used to perform phonetic matching by converting words into sound codes.
You can also use DIFFERENCE() in conjunction with SOUNDEX()
to compare the similarity between strings more effectively.
Using SOUNDEX() for Phonetic Matching in MySQL
The SOUNDEX()
function converts a string into a four-character code based on how it sounds. Words that sound alike produce the same SOUNDEX code, which makes it possible to match names or terms phonetically. The general syntax for SOUNDEX()
is:
SOUNDEX(string);
To demonstrate phonetic matching, we’ll set up a sample table with names, then use SOUNDEX()
to compare similar-sounding names.
Setting Up an Example Table
We’ll create a table called people
with a name
column to store names for our phonetic matching examples.
1. Create a new database called sample_db
(if it doesn’t already exist):
CREATE DATABASE IF NOT EXISTS sample_db;
2. Select the sample_db
database:
USE sample_db;
3. Create the people
table with a name
column:
CREATE TABLE people (
id INT PRIMARY KEY AUTO_INCREMENT,
name VARCHAR(50)
);
4. Insert sample data into the people
table:
INSERT INTO people (name)
VALUES ('Smith'), ('Smyth'), ('Jon'), ('John'), ('Doe'), ('Joan');
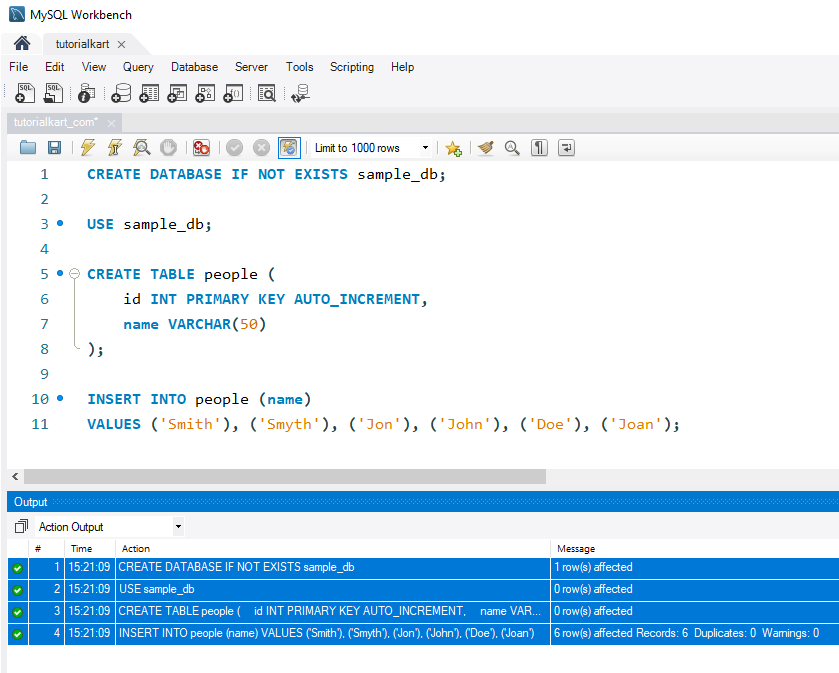
Now that the table is set up, we can use SOUNDEX()
for phonetic matching with this data.
Examples of Phonetic Matching in MySQL
Below are some examples of using SOUNDEX()
for phonetic matching in MySQL.
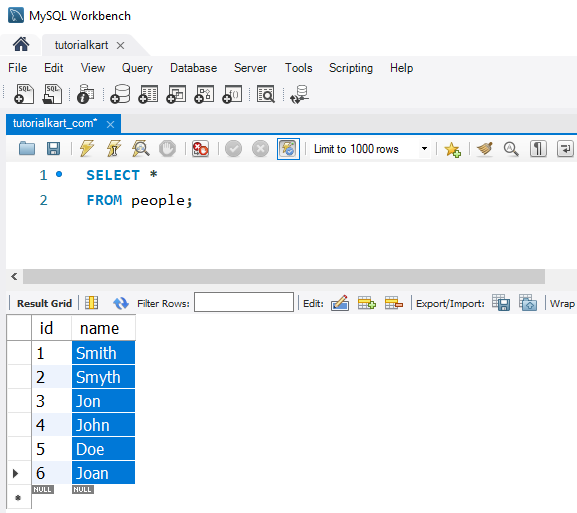
1 Finding Phonetically Similar Names
To find names that sound similar to “Smith” in the people
table:
SELECT name
FROM people
WHERE SOUNDEX(name) = SOUNDEX('Smith');
This query returns names like “Smyth” because they share the same SOUNDEX
code, indicating phonetic similarity.
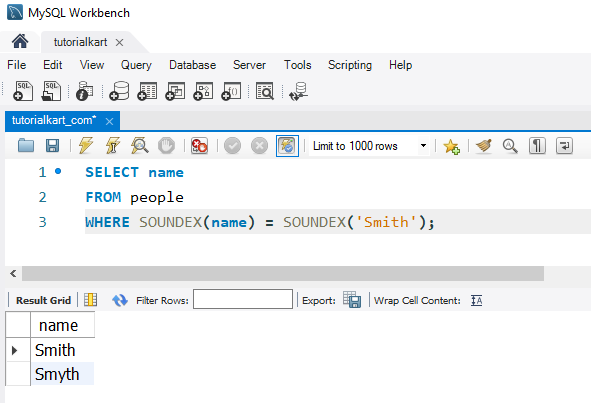
2 Using SOUNDEX with DIFFERENCE to Compare Similarity
In SQL Server, you can combine SOUNDEX
with DIFFERENCE()
to get a similarity score from 0 to 4, with higher scores indicating closer matches. In MySQL, you can use custom functions to achieve similar results, as DIFFERENCE()
is not directly supported.
To retrieve names phonetically similar to “John”:
SELECT name
FROM people
WHERE SOUNDEX(name) = SOUNDEX('John');
This query finds matches for “John” and similar-sounding names like “Jon”. SOUNDEX()
can be combined with other conditions to refine results.
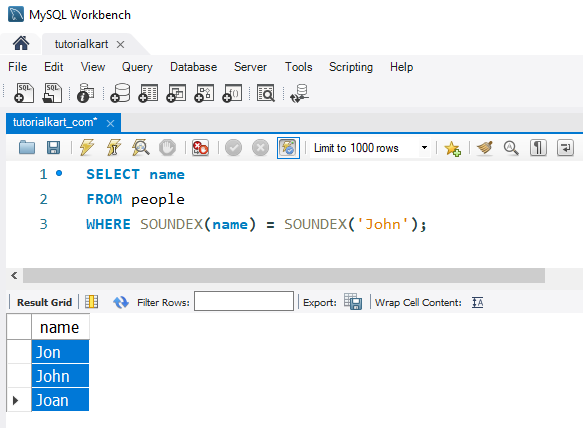
3 Using SOUNDEX to Group Phonetically Similar Names
To identify and group names that sound similar for reporting or standardization purposes:
SELECT SOUNDEX(name) AS sound_code, GROUP_CONCAT(name) AS similar_names
FROM people
GROUP BY sound_code
ORDER BY sound_code;
This query groups names by their SOUNDEX
code, showing phonetically similar names together in the results.
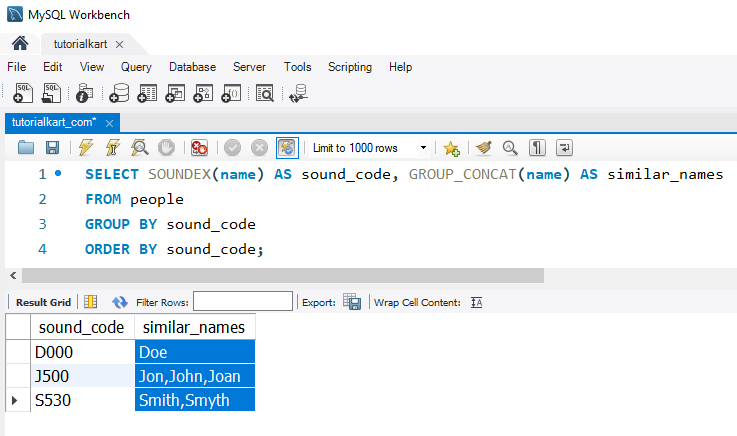