Reverse Strings in MySQL
In MySQL, you can use the REVERSE()
function to reverse strings directly within your SQL queries.
In MySQL, reversing a string can be useful in various scenarios, such as data formatting, palindrome checking, or simply presenting data in a different orientation.
Using the REVERSE() Function in MySQL
The REVERSE()
function in MySQL takes a string as input and returns it with all characters in reverse order. This function works with various data types, including CHAR
and VARCHAR
, and can handle multi-byte character strings.
Let’s set up an example table and demonstrate how to use the REVERSE()
function in a few practical scenarios.
Setting Up an Example Table
To illustrate the REVERSE()
function, we’ll create a words
table with a text
column containing sample text entries to test the function.
1. Create a new database called sample_db
:
CREATE DATABASE sample_db;
2. Select the sample_db
database to work with:
USE sample_db;
3. Create the words
table with a text
column:
CREATE TABLE words (
id INT PRIMARY KEY AUTO_INCREMENT,
text VARCHAR(255)
);
4. Insert sample data into the words
table:
INSERT INTO words (text)
VALUES ('Hello World'),
('MySQL Function'),
('Palindrome'),
('Reverse this text');
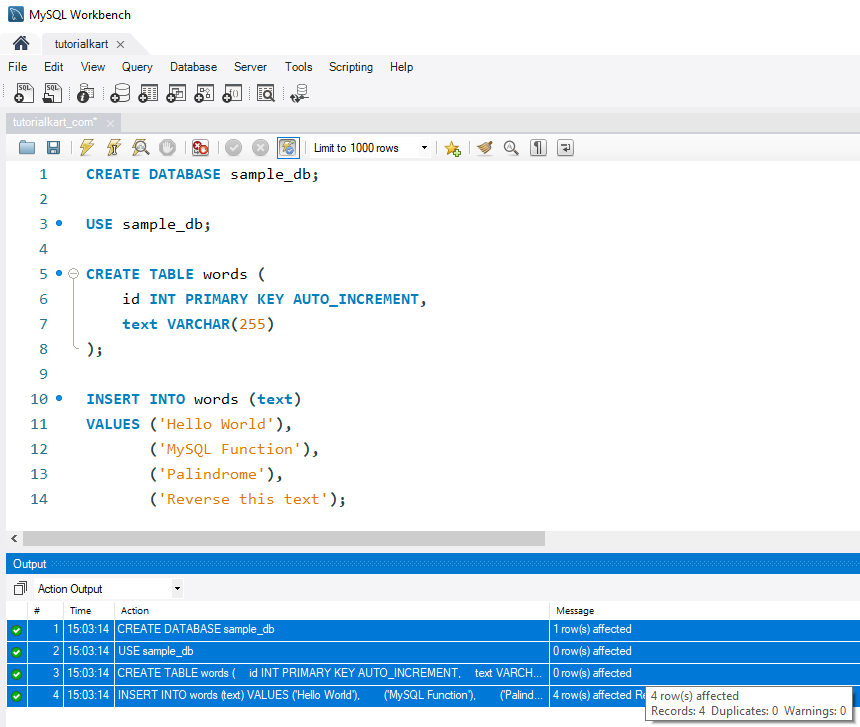
With the table set up, you can use the REVERSE()
function to reverse the text in these entries.
Examples: Reversing Strings in MySQL
Let’s explore a few examples of using REVERSE()
to manipulate text in MySQL.
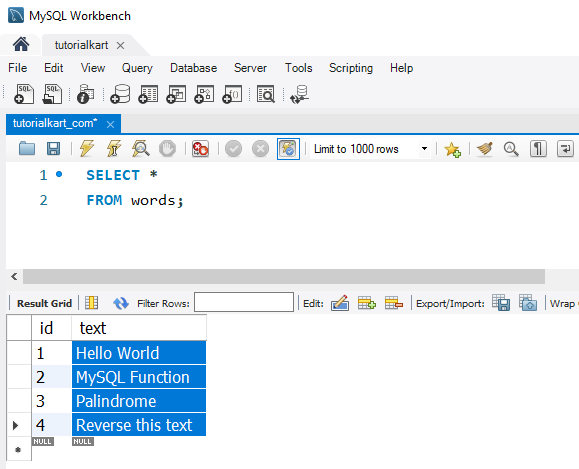
1 Reversing a Text Column
To reverse the text in the text
column for each entry:
SELECT text, REVERSE(text) AS reversed_text
FROM words;
This query returns each original text string alongside its reversed version, allowing you to see how REVERSE()
rearranges the characters.
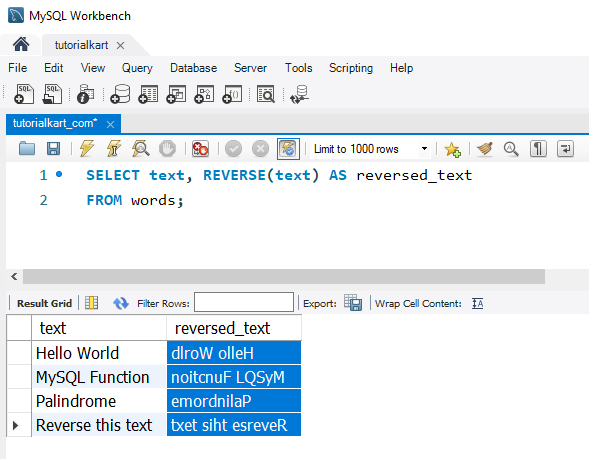
2 Checking for Palindromes Using REVERSE()
To check if each text entry is a palindrome (i.e., it reads the same forward and backward):
SELECT text,
CASE
WHEN text = REVERSE(text) THEN 'Yes'
ELSE 'No'
END AS is_palindrome
FROM words;
This query checks if each text entry matches its reversed form, indicating whether or not it is a palindrome.
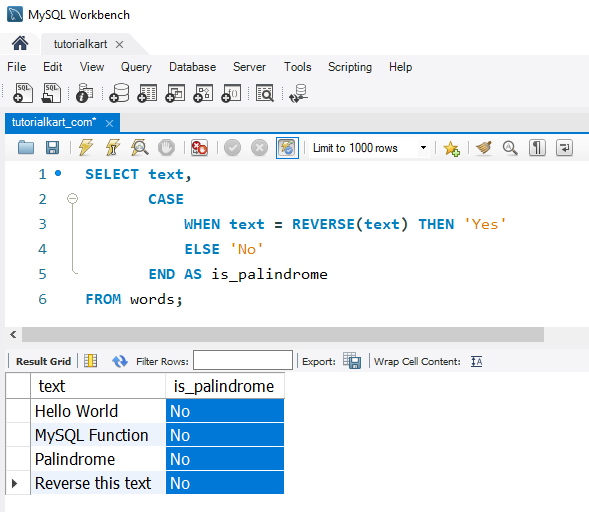
3 Using REVERSE() with CONCAT() for Customized Output
To display a reversed text string with a prefix, such as “Reversed: “:
SELECT CONCAT('Reversed: ', REVERSE(text)) AS modified_text
FROM words;
This query appends the prefix “Reversed: ” before each reversed text, producing a customized output for each entry.
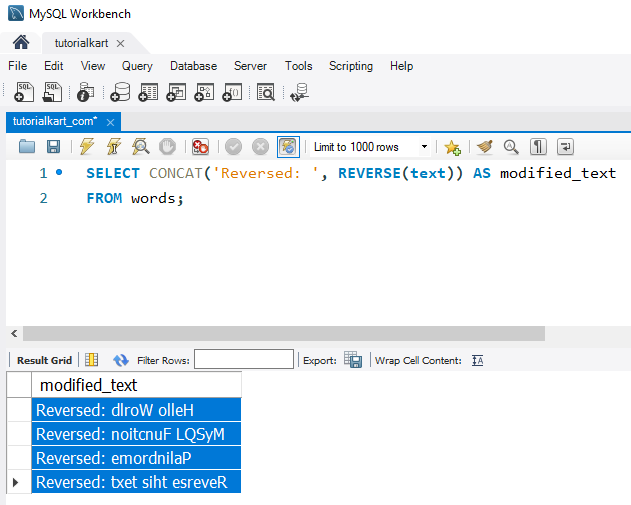