MySQL – Drop Table
The DROP TABLE
statement in MySQL is used to delete an entire table, including all its data and structure. This operation is permanent and cannot be undone, so it’s important to use DROP TABLE
carefully.
This tutorial covers the syntax, examples of dropping tables, and how to handle cases where a table doesn’t exist.
Syntax – Drop a Table
The basic syntax to drop a table in MySQL is as follows:
DROP TABLE table_name;
where table_name is the name of the table you want to delete.
Example 1: Dropping an Existing Table
In this example, we’ll create a sample database and table, then drop the table using the DROP TABLE
command.
Step 1: Create a Database
To start, we need a database to store our table. Use the CREATE DATABASE
command to create a new database.
CREATE DATABASE school;
This command creates a database named school
. You can change the name if needed.
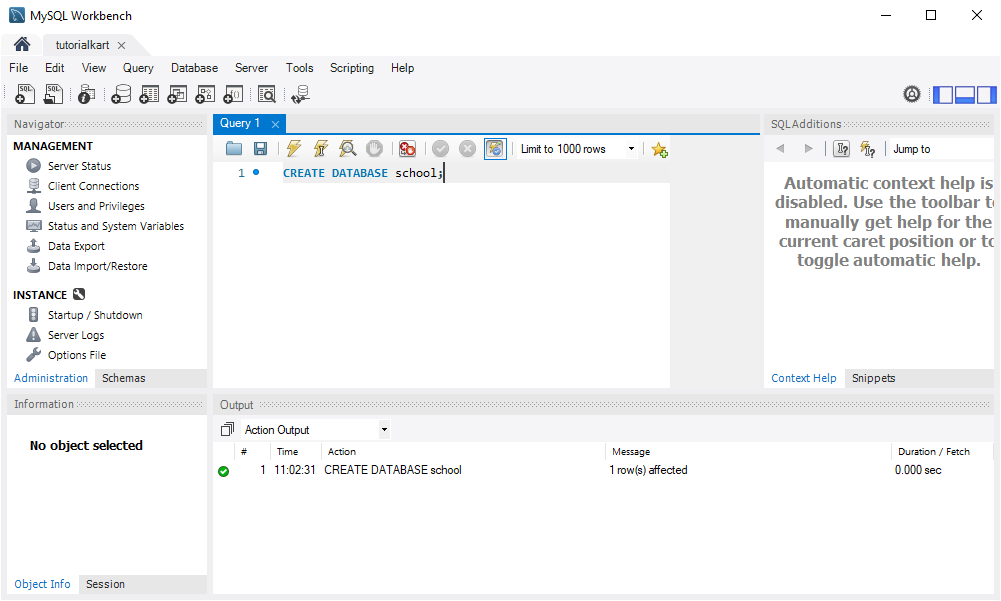
Step 2: Select the Database
After creating the database, use the USE
command to select it so we can create tables and work with it.
USE school;
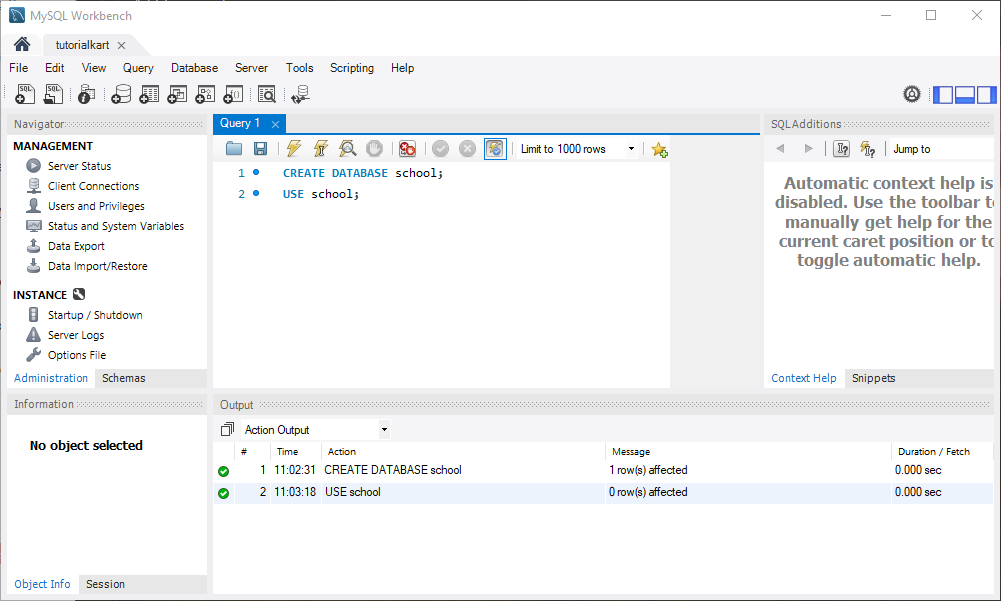
Step 3: Create a Table
Now, let’s create a students
table with columns for storing student details.
CREATE TABLE students (
id INT AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(50),
rollno INT,
age INT,
class VARCHAR(10)
);
This command creates a students
table with columns for ID, name, roll number, age, and class.
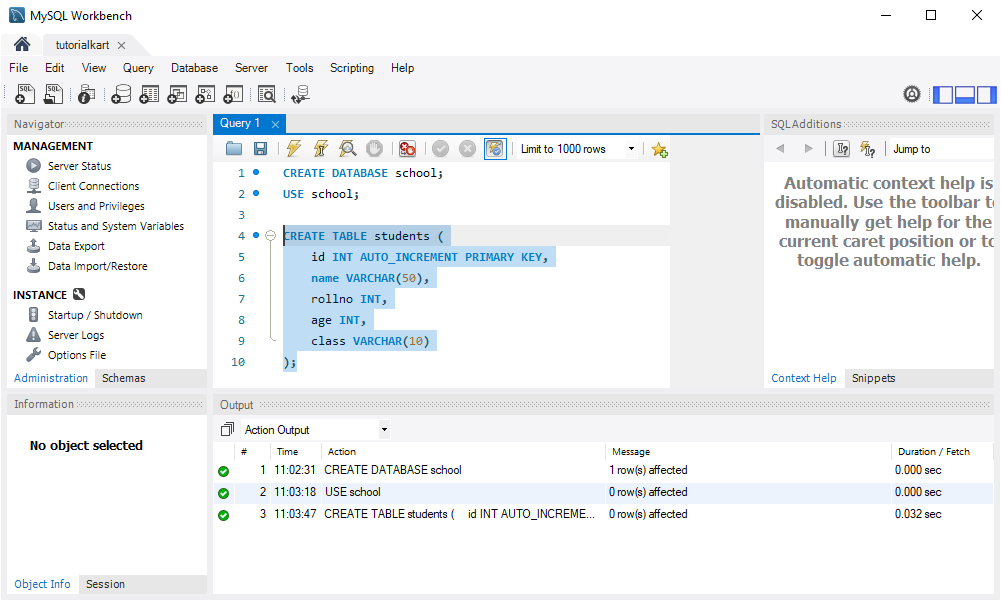
Step 4: Drop the Table
Once the students
table is created, you can delete it using the DROP TABLE
command:
DROP TABLE students;
This command removes the students
table from the school
database, including all its data and structure.
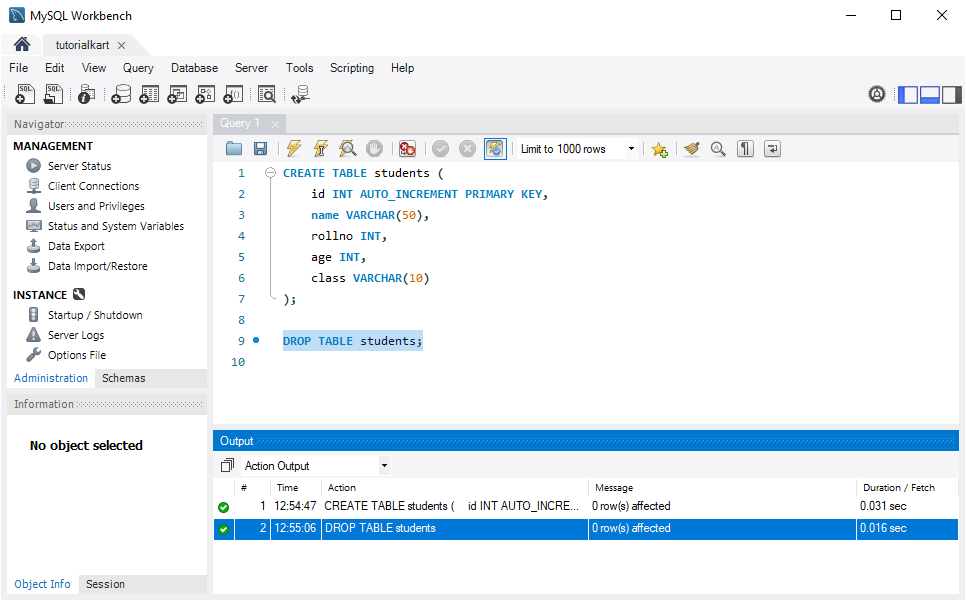
Example 2: Dropping Multiple Tables
You can also delete multiple tables in a single command by separating their names with commas. For example, to drop tables students
and teachers
:
DROP TABLE students, teachers;
This command drops both the students
and teachers
tables.
Handling Errors When Table Does Not Exist
If you attempt to drop a table that doesn’t exist, MySQL will return an error. For example, if students
has already been deleted and you try to drop it again:
DROP TABLE students;
You will see an error like this:
Error Code: 1051. Unknown table 'school.students'
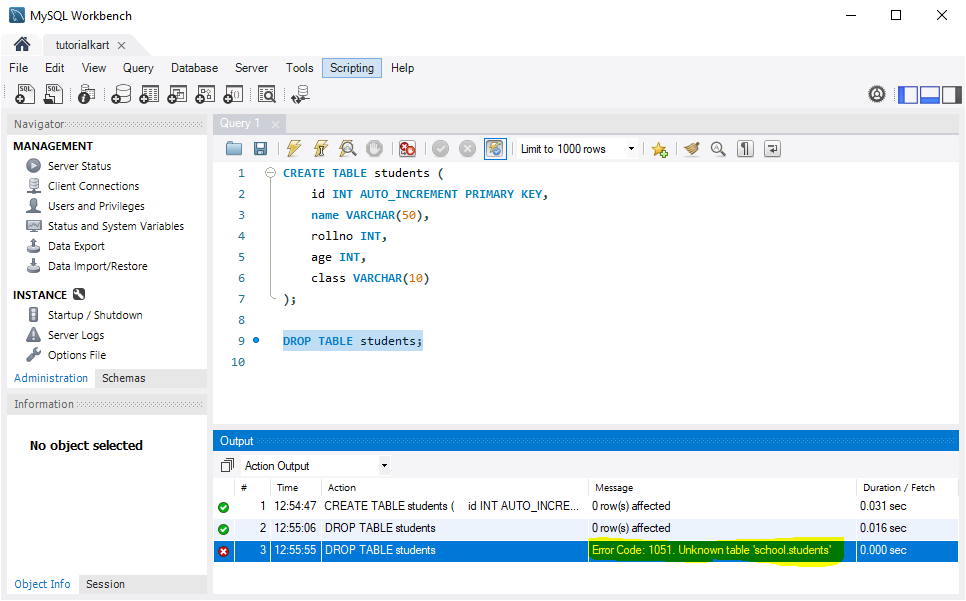
Solution: Use IF EXISTS Clause
To prevent errors when a table doesn’t exist, use the IF EXISTS
clause with DROP TABLE
. This option checks if the table exists before attempting to drop it. If the table isn’t found, MySQL will skip the command without raising an error.
DROP TABLE IF EXISTS students;
This command will only drop the students
table if it exists. If it doesn’t exist, MySQL will execute the command without any errors.
Alternative: Using TRUNCATE Instead of DROP
If you only need to remove all data from a table but want to keep the table structure, use the TRUNCATE TABLE
command instead of DROP TABLE
. TRUNCATE
clears all data but retains the table structure.
TRUNCATE TABLE students;
This command removes all rows from the students
table but keeps the table structure intact.
Conclusion
The DROP TABLE
statement in MySQL is a powerful command to delete an entire table permanently. Use it carefully, as it removes all data and the table structure. Using IF EXISTS
helps prevent errors when a table doesn’t exist, while TRUNCATE TABLE
is an alternative if you only need to clear data but retain the table structure.