1 What is Natural Language Processing (NLP)?
Natural Language Processing (NLP) is a field of Artificial Intelligence that focuses on enabling computers to understand, interpret, and generate human language. Simply put, NLP helps machines communicate with humans in a natural way.
Definition, Importance, and Applications
Human language is complex and ambiguous, making it difficult for machines to understand. NLP bridges this gap by using computational techniques to process and analyze text or speech.
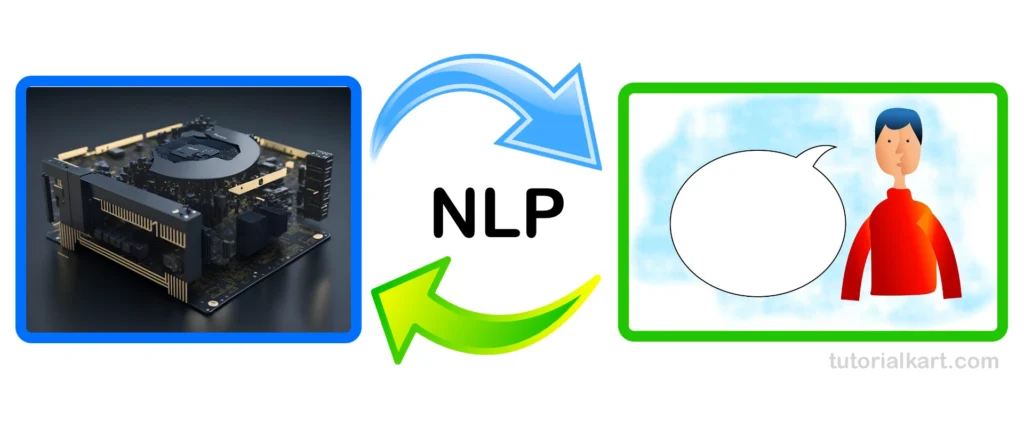
Importance of NLP:
- Helps in automating repetitive tasks such as customer support (Chatbots).
- Improves search engines by understanding user queries.
- Assists in analyzing large volumes of text for insights (e.g., sentiment analysis in reviews).
- Enables language translation (Google Translate).
NLP vs. Traditional Text Processing
Traditional text processing involves simple text manipulation like searching for a word or counting its occurrences. NLP, on the other hand, focuses on understanding the meaning, structure, and context of the text.
Traditional Text Processing | Natural Language Processing |
---|---|
Finds specific words | Understands the context of words |
Keyword-based search | Intent-based search |
Cannot detect sarcasm or tone | Can analyze sentiment and emotions |
Works with exact string matching | Handles variations and synonyms in text |
Ignores grammatical structure | Analyzes sentence structure and meaning |
Basic rule-based processing | Uses machine learning and deep learning models |
Cannot handle ambiguous meanings | Can resolve ambiguity through context |
Fails in complex language tasks like summarization | Can summarize text using AI-based models |
Search and replace operations | Performs sentiment analysis and topic modeling |
Limited to exact phrases and patterns | Can handle multiple languages and dialects |
Cannot generate human-like responses | Powers chatbots and AI-driven text generation |
Does not understand context in sentences | Understands word relationships and dependencies |
Cannot recognize named entities (e.g., places, names) | Uses Named Entity Recognition (NER) to detect names, places, dates |
Primarily works with structured data | Can process unstructured text data (articles, tweets, chats) |
Rule-based grammar checking | AI-powered grammar correction and text enhancement |
Real-World Examples of NLP
1. Chatbots: Virtual assistants like Siri, Alexa, and customer support bots use NLP to understand and respond to human queries.
2. Search Engines: Google and Bing use NLP to suggest and rank search results based on user intent.
3. Speech Recognition: NLP enables voice assistants to convert speech into text and respond accurately.
2 Text Preprocessing Techniques
Before applying NLP techniques, raw text must be cleaned and structured properly. This process is called text preprocessing.
Tokenization
Tokenization is the process of breaking text into smaller pieces (tokens). Tokens can be words, sentences, or even subwords.
Example:
Input: "NLP is fascinating!"
Word Tokens: ["NLP", "is", "fascinating", "!"]
Sentence Tokens: ["NLP is fascinating!"]
Stop-word Removal
Stop-words are common words (like “the”, “is”, “and”) that do not add significant meaning to a sentence. Removing them helps in reducing text size and improving efficiency.
Example:
Input: "The cat is sleeping on the mat."
After Stop-word Removal: ["cat", "sleeping", "mat"]
Stemming vs. Lemmatization
Both techniques reduce words to their root form, but in different ways.
- Stemming: Removes prefixes and suffixes, often leading to incomplete words.
- Lemmatization: Converts words into meaningful base forms using a dictionary.
Example:
Word | Stemming | Lemmatization |
---|---|---|
Running | Runn | Run |
Better | Better | Good |
Case Normalization and Text Cleaning
Text normalization converts text to a standard format, making processing more effective.
- Lowercasing: “Hello” → “hello”
- Removing punctuation: “Hello, World!” → “Hello World”
- Removing extra spaces:
"NLP is fun"
→"NLP is fun"
3 Regular Expressions for Text Processing
Regular expressions (regex) are patterns used to find and manipulate text efficiently.
Pattern Matching in NLP
Regex helps in tasks like extracting phone numbers, emails, or hashtags.
Example:
Pattern: \d{10} (Finds a 10-digit phone number)
Text: "Call me at 9876543210."
Match: 9876543210
Applications of Regex in NLP
- Finding dates in text (e.g., “12/02/2024”)
- Extracting mentions in social media (e.g., “@username”)
- Validating email formats
4 Working with Text in Python (NLTK, SpaCy)
Python provides powerful NLP libraries such as NLTK and SpaCy for text processing.
Loading and Processing Text Data
Using NLTK to tokenize text:
import nltk
nltk.download('punkt')
from nltk.tokenize import word_tokenize
text = "Natural Language Processing is amazing!"
tokens = word_tokenize(text)
print(tokens) # Output: ['Natural', 'Language', 'Processing', 'is', 'amazing', '!']
POS Tagging Basics
Part-of-Speech (POS) tagging assigns grammatical categories (noun, verb, adjective) to words.
from nltk import pos_tag
tokens = word_tokenize("NLP is fun")
pos_tags = pos_tag(tokens)
print(pos_tags) # Output: [('NLP', 'NNP'), ('is', 'VBZ'), ('fun', 'JJ')]
5 Conclusion
This tutorial introduced the basics of NLP, its importance, and fundamental text preprocessing techniques. In the next tutorials, we will explore each of these topics in greater depth.