Introduction to Lemmatization in NLP
Lemmatization is a fundamental text preprocessing technique in Natural Language Processing (NLP). It involves reducing words to their root or base form while ensuring that the transformed word is a valid word in the dictionary.
For example:
Running → Run
Better → Good
Mice → Mouse
Unlike stemming, which simply chops off word endings, lemmatization considers the meaning of the word and its role in the sentence. It uses linguistic rules and a vocabulary (like WordNet) to ensure the base word (lemma) is meaningful.
Why is Lemmatization Important?
When processing text, different variations of the same word (run, running, ran) can exist. If we don’t normalize them, NLP models may treat them as different words, which can lead to inefficiencies.
Here’s why lemmatization is useful:
- Improves text consistency – Reduces variations of words.
- Enhances search engines – Searching for “run” should return results for “running” and “ran”.
- Optimizes NLP models – Reduces vocabulary size, making models efficient.
- Ensures correct word forms – Unlike stemming, lemmatization produces meaningful words.
Difference Between Lemmatization and Stemming
Stemming and lemmatization both reduce words to their base forms, but they work differently.
Feature | Stemming | Lemmatization |
---|---|---|
Method | Removes prefixes/suffixes | Uses vocabulary & grammar rules |
Word Meaning | May produce incorrect words | Always produces valid words |
Examples | “Running” → “Run”, “Studies” → “Studi” | “Running” → “Run”, “Studies” → “Study” |
Types of Lemmatization
Lemmatization can be divided into different categories based on the word type and context.
1 Verb Lemmatization
Verbs have different tenses, and lemmatization brings them back to their base (infinitive) form.
Playing → Play
Ran → Run
Eats → Eat
2 Noun Lemmatization
Nouns have singular and plural forms. Lemmatization reduces them to their singular form.
Mice → Mouse
Geese → Goose
Children → Child
3 Adjective Lemmatization
Adjectives may have comparative or superlative forms. Lemmatization converts them to their root form.
Better → Good
Worst → Bad
4 Lemmatization of Irregular Words
Some words do not follow standard rules, but lemmatization handles them correctly.
Was → Be
Went → Go
How Lemmatization Works
Lemmatization relies on:
- Dictionary Lookup – Words are mapped to their root forms using dictionaries like WordNet.
- POS (Part-of-Speech) Tagging – The role of the word (noun, verb, adjective) affects how it is lemmatized.
Example: The word “running” can have different lemmatized forms based on POS tagging.
- Verb: “running” → “run”
- Noun: “running” (the act of running) → “running” (unchanged)
Implementing Lemmatization in Python
1 Lemmatization using NLTK
NLTK (Natural Language Toolkit) provides a WordNet-based lemmatizer.
Example: main.py
from nltk.stem import WordNetLemmatizer
from nltk.corpus import wordnet
nltk.download('wordnet')
lemmatizer = WordNetLemmatizer()
# Examples
print(lemmatizer.lemmatize("running", pos=wordnet.VERB)) # Output: run
print(lemmatizer.lemmatize("better", pos=wordnet.ADJ)) # Output: good
Output:
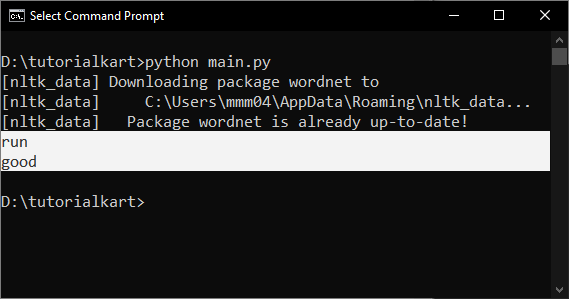
2 Lemmatization using SpaCy
SpaCy is another powerful NLP library that supports lemmatization.
Example: main.py
import spacy
nlp = spacy.load("en_core_web_sm")
doc = nlp("The children were running and playing in the park.")
print([token.lemma_ for token in doc])
Output:
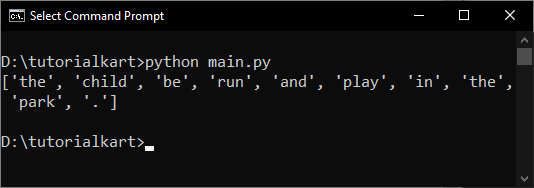
When to Use Lemmatization?
Lemmatization is useful when:
- You need correct words with meaning.
- You’re working with search engines, chatbots, or language models.
- You’re analyzing text where different word forms should be treated as the same (e.g., “run” and “running”).
Challenges in Lemmatization
Despite its benefits, lemmatization has some challenges:
- Computational Cost: Lemmatization is slower than stemming as it requires dictionary lookups.
- Context Dependency: Some words require correct POS tagging for accurate lemmatization.
- Handling Rare Words: Lemmatizers may not recognize uncommon words.
Conclusion
Lemmatization is a crucial text normalization technique in NLP, ensuring words are mapped to their correct root forms while maintaining meaning. Unlike stemming, which often results in incorrect words, lemmatization produces meaningful words that improve text analysis.
In the next tutorials, we will explore:
- Advanced Lemmatization Techniques
- Using Lemmatization in NLP Pipelines
- Combining Lemmatization with Other Preprocessing Steps