NumPy sinc()
The numpy.sinc()
function computes the normalized sinc function, which is defined as:
sinc(x) = sin(πx) / (πx), for x ≠ 0
sinc(0) = 1
It is widely used in signal processing applications. The function is normalized using π, meaning it differs from the unnormalized sinc function used in pure mathematics.
Syntax
numpy.sinc(x)
Parameters
Parameter | Type | Description |
---|---|---|
x | ndarray | An array (possibly multi-dimensional) containing values for which to calculate sinc(x). |
Return Value
Returns an array of the same shape as x
, where each element is computed as sinc(x)
.
Examples
1. Computing sinc of a Single Value
In this example, we compute the sinc function of a single value.
import numpy as np
# Compute sinc for a single value
x = 0 # sinc(0) should return 1
result = np.sinc(x)
# Print the result
print("sinc(0):", result)
Output:
sinc(0): 1.0

2. Computing sinc for an Array of Values
Here, we compute the sinc function for multiple values in an array.
import numpy as np
# Define an array of values
x_values = np.array([-2, -1, 0, 1, 2])
# Compute sinc values
sinc_values = np.sinc(x_values)
# Print the results
print("Input values:", x_values)
print("Computed sinc values:", sinc_values)
Output:
Input values: [-2 -1 0 1 2]
Computed sinc values: [ 0.45464871 0.84147098 1. 0.84147098 0.45464871]

3. Plotting the sinc Function
We visualize the sinc function over a range of values.
import numpy as np
import matplotlib.pyplot as plt
# Generate values from -10 to 10
x = np.linspace(-10, 10, 1000)
# Compute sinc values
y = np.sinc(x)
# Plot the function
plt.plot(x, y, label="sinc(x)")
plt.axhline(0, color='black', linewidth=0.5, linestyle="--")
plt.axvline(0, color='black', linewidth=0.5, linestyle="--")
plt.title("Plot of sinc(x)")
plt.xlabel("x")
plt.ylabel("sinc(x)")
plt.legend()
plt.grid()
plt.show()
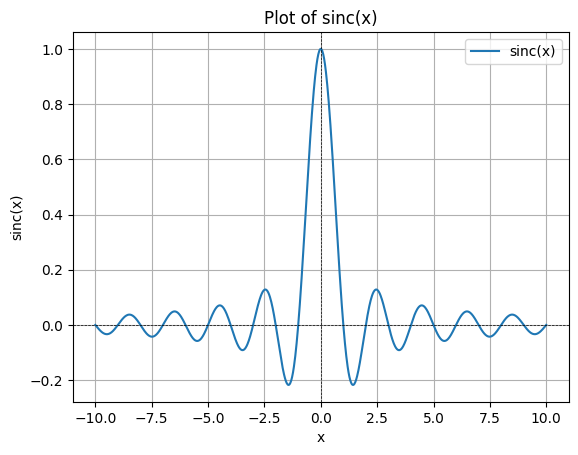
4. Computing Unnormalized sinc Function
The default numpy.sinc()
function is normalized using π. To compute the unnormalized sinc function (common in mathematics), divide x
by π.
import numpy as np
# Define an array of values
x_values = np.array([-2, -1, 0, 1, 2])
# Compute unnormalized sinc values
unnormalized_sinc_values = np.sinc(x_values / np.pi)
# Print the results
print("Input values:", x_values)
print("Unnormalized sinc values:", unnormalized_sinc_values)
Output:
Input values: [-2 -1 0 1 2]
Unnormalized sinc values: [0.45464871 0.84147098 1. 0.84147098 0.45464871]
By dividing x
by π before passing it to np.sinc()
, we get the unnormalized sinc function, commonly used in mathematical contexts.
