Python Pillow Tutorial
Pillow is a Python Library that can be used for image processing.
In this Pillow Tutorial, we will learn how to use Pillow library for some of the basic image processing operations.
Install Pillow
We will start with installing pillow package using pip.
To install pillow, run the following pip command.
pip install Pillow
C:\>pip install Pillow
Collecting Pillow
Installing collected packages: Pillow
Successfully installed Pillow-5.4.1
Read Image
You can read an image using Image.open() method of Python Pillow library.
Python Program
from PIL import Image
im = Image.open("image1.png")
Image.open() returns Pillow Image object. The input image can be a binary image, greyscale image or color image.
Show or Display Image
You can show the image read, using Image.show() method. The image is displayed in a window.
Python Program
from PIL import Image
# read an image
im = Image.open("image1.png")
# show the image in a window
im.show()
Output
Run the program, and the default program in your OS to display the image will start to show the image.
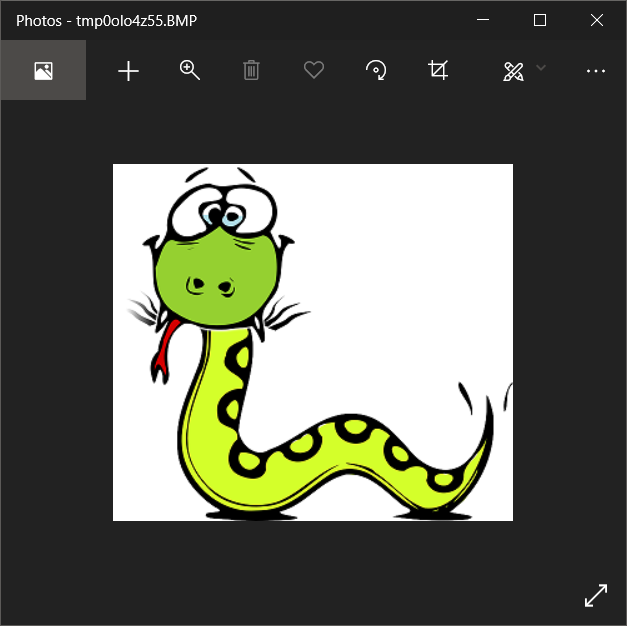
Resize Image
To resize image using PIL, follow these steps.
- Import Image from PIL package.
- Open image using Image.open() method. It returns an object.
- Call resize(size) method on the object. size is a tuple representing the target size.
- resize() method returns the resized image.
You can use this image object to save to persistent storage or show it like in the following program.
Python Program
from PIL import Image
# read an image
im = Image.open("python-image.png")
#resize image
im = im.resize((100, 100))
im.show()
Output
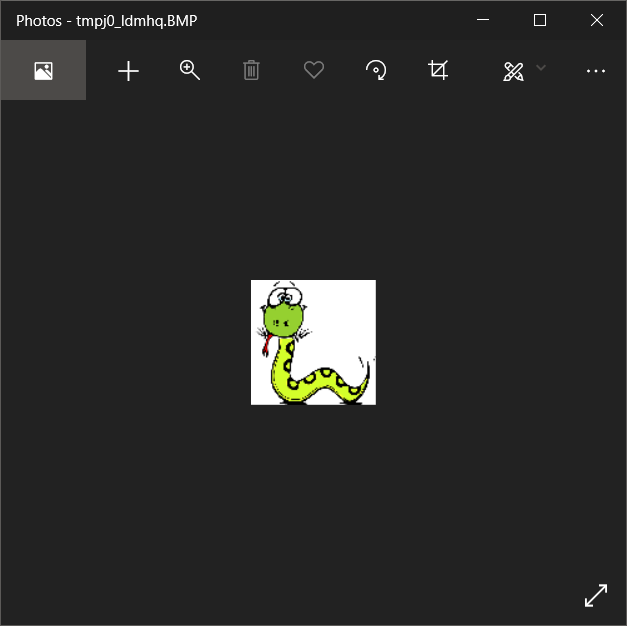
Rotate Image
rotate(angle) method rotates the image by given angle in degrees, and returns the resulting image.
Python Program
from PIL import Image
# read an image
im = Image.open("python-image.png")
#rotate image
im = im.rotate(90)
im.show()
Output
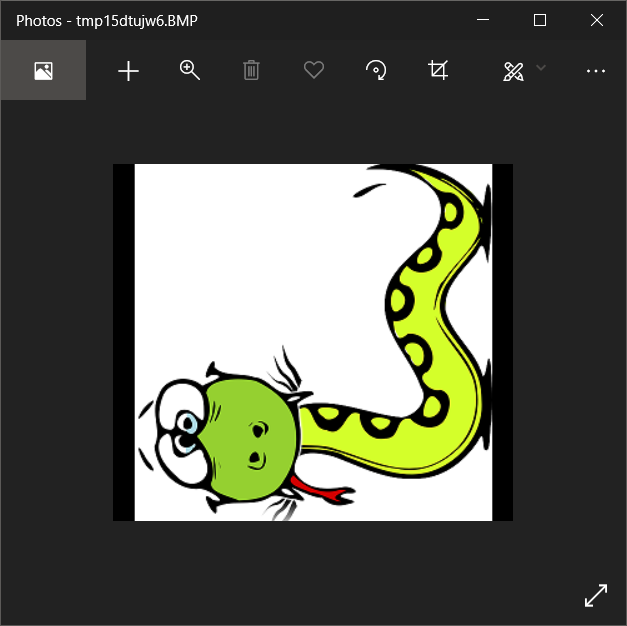
Flip Image
transpose(method) function transposes the image using the given transpose method. method can take these values.
- PIL.Image.FLIP_LEFT_RIGHT
- PIL.Image.FLIP_TOP_BOTTOM
- PIL.Image.ROTATE_90
- PIL.Image.ROTATE_180
- PIL.Image.ROTATE_270
- PIL.Image.TRANSPOSE or PIL.Image.TRANSVERSE.
Let us pass PIL.Image.FLIP_LEFT_RIGHT for method, and flip the image along vertical axis.
Python Program
from PIL import Image
# read an image
im = Image.open("python-image.png")
#resize image
im = im.transpose(Image.FLIP_LEFT_RIGHT)
im.show()
Output
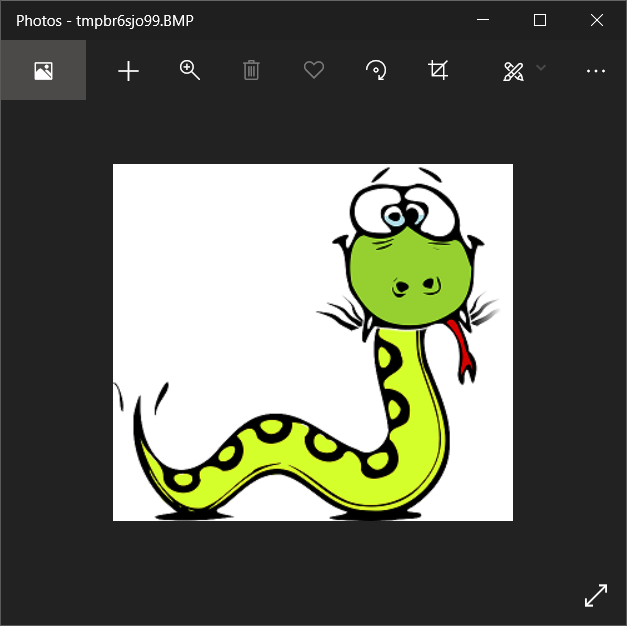
Save Image
Image.save() method saves the image in specified format.
Python Program
from PIL import Image
# read an image
im = Image.open("python-image.png")
#image transformations
im = im.transpose(Image.FLIP_LEFT_RIGHT)
#save image
im.save('result-image.png', 'PNG')
Run this program, and an image file with the name ‘result-image.png’ will be created in the current directory. You may specify the absolute path or relative path along with the file name to save it to a different location.
Conclusion
In this Python Tutorial, we learned how to use Python Pillow library.