Set Color for Label Text in Tkinter Python
In Tkinter, the fg
(foreground) option of a Label widget allows you to set the text color. The color can be specified using different formats:
- 4-bit per color hex code – e.g.,
#rgb
- 8-bit per color hex code – e.g.,
#rrggbb
- 12-bit per color hex code – e.g.,
#rrrgggbbb
- Standard color names – e.g.,
'red'
,'blue'
,'green'
To set color for label widget in Tkinter, you can set the fg
option with the required color value, while creating the label.
In this tutorial, we will go through examples using the fg
option to modify the text color of a Tkinter Label widget.
Examples
1. Setting Label Text Color Using Standard Color Names
In this example, we will create a Tkinter window and set the text color of Label widgets using standard color names.
main.py
import tkinter as tk
root = tk.Tk()
root.title("tutorialkart.com - Label Text Color")
root.geometry("400x200")
# Create Labels with different colors
label_red = tk.Label(root, text="Red Text", fg="red", font=("Arial", 14))
label_red.pack(pady=5)
label_blue = tk.Label(root, text="Blue Text", fg="blue", font=("Arial", 14))
label_blue.pack(pady=5)
label_green = tk.Label(root, text="Green Text", fg="green", font=("Arial", 14))
label_green.pack(pady=5)
root.mainloop()
Output in Windows:
Three labels with text colors red, blue, and green are displayed.
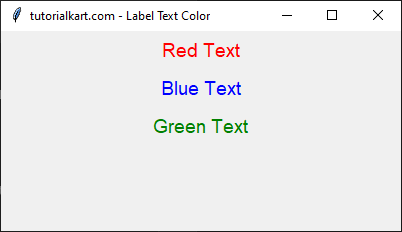
2. Setting Label Text Color Using 4-bit Per Color Hex Code
In this example, we set the label text color using a 4-bit per color hex code (#rgb
format).
main.py
import tkinter as tk
root = tk.Tk()
root.title("tutorialkart.com - Label Text Color")
root.geometry("400x200")
# Create Labels with 4-bit per color hex code
label = tk.Label(root, text="Short Hex Code Text", fg="#f00", font=("Arial", 14)) # Red color
label.pack(pady=10)
root.mainloop()
Output in Windows:
A label with red text using a short hex code appears.
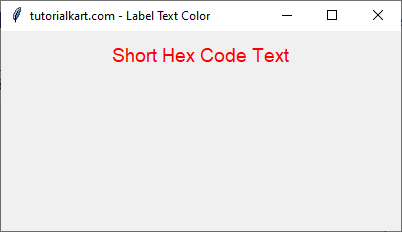
3. Setting Label Text Color Using 8-bit Per Color Hex Code
Here, we set the label text color using an 8-bit per color hex code (#rrggbb
format).
main.py
import tkinter as tk
root = tk.Tk()
root.title("tutorialkart.com - Label Text Color")
root.geometry("400x200")
# Create Label with 8-bit per color hex code
label = tk.Label(root, text="8-bit Hex Code Text", fg="#ff853a", font=("Arial", 14)) # Orange color
label.pack(pady=10)
root.mainloop()
Output in Windows:
A label with orange-colored text using an 8-bit hex code appears.
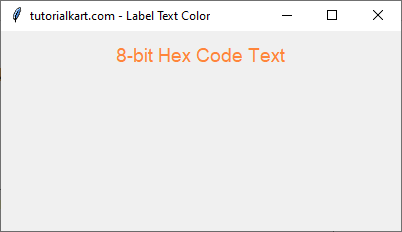
4. Setting Label Text Color Using 12-bit Per Color Hex Code
In this example, we will set the label text color using a 12-bit per color hex code (#rrrgggbbb
format).
main.py
import tkinter as tk
root = tk.Tk()
root.title("tutorialkart.com - Label Text Color")
root.geometry("400x200")
# Create Label with 12-bit per color hex code
label = tk.Label(root, text="12-bit Hex Code Text", fg="#ff8aba53a", font=("Arial", 14))
label.pack(pady=10)
root.mainloop()
Output in Windows:
A label with custom-colored text using a 12-bit hex code appears.
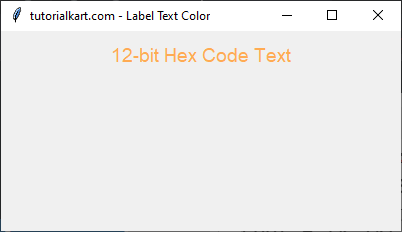
Conclusion
In this tutorial, we explored how to use the fg
option in Tkinter’s Label widget to control text color. The color can be set using:
- Standard color names (
'red'
,'blue'
, etc.) - 4-bit per color hex codes (
#rgb
) - 8-bit per color hex codes (
#rrggbb
) - 12-bit per color hex codes (
#rrrgggbbb
)
By customizing text colors, you can enhance the visual appeal of your Tkinter application.