Set Font Color for Text in Button in Tkinter
In Tkinter, the fg
(foreground) option of a Button widget allows you to set the font color of the text inside the button. The color can be specified in different formats, including:
- 4-bit per color (short hex code) – e.g.,
#rgb
- 8-bit per color (standard hex code) – e.g.,
#rrggbb
- 12-bit per color (extended hex code) – e.g.,
#rrrgggbbb
- Standard color names – e.g.,
'red'
,'blue'
,'green'
To set the font color for a Button in Tkinter, pass the fg
parameter with the required color value while creating the Button.
In this tutorial, we will go through examples using the fg
option to change the text color in a Tkinter Button.
Examples
1. Setting Font Color Using 4-bit Per Color Hex Code
In this example, we will create a Tkinter button and set the text color using a 4-bit per color hex code (#rgb
format).
import tkinter as tk
root = tk.Tk()
root.title("Button Font Color Example")
root.geometry("400x200")
# Create a button with 4-bit per color hex code
button = tk.Button(root, text="Click Me", fg="#f00") # Red color
button.pack(pady=10)
root.mainloop()
Screenshot in Windows
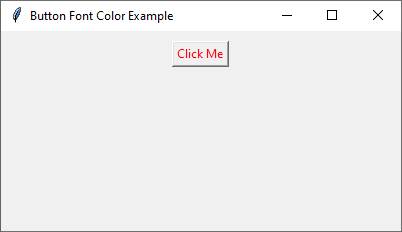
2. Setting Font Color Using 8-bit Per Color Hex Code
In this example, we will set the text color using an 8-bit per color hex code (#rrggbb
format).
import tkinter as tk
root = tk.Tk()
root.title("Button Font Color Example")
root.geometry("400x200")
# Create a button with 8-bit per color hex code
button = tk.Button(root, text="Click Me", fg="#ff853a") # Orange color
button.pack(pady=10)
root.mainloop()
Screenshot in Windows
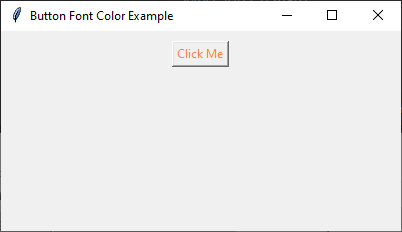
3. Setting Font Color Using 12-bit Per Color Hex Code
In this example, we will set the text color using a 12-bit per color hex code (#rrrgggbbb
format).
import tkinter as tk
root = tk.Tk()
root.title("Button Font Color Example")
root.geometry("400x200")
# Create a button with 12-bit per color hex code
button = tk.Button(root, text="Click Me", fg="#000999000") # Custom shade
button.pack(pady=10)
root.mainloop()
Screenshot in Windows
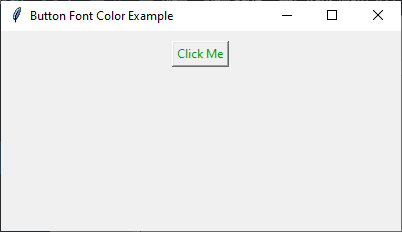
4. Setting Font Color Using Standard Color Names
In this example, we will set the text color using a standard color name like 'red'
, 'blue'
, and 'green'
.
import tkinter as tk
root = tk.Tk()
root.title("Button Font Color Example")
root.geometry("400x200")
# Create buttons with different color names
btn_red = tk.Button(root, text="Red Text", fg="red")
btn_red.pack(pady=5)
btn_blue = tk.Button(root, text="Blue Text", fg="blue")
btn_blue.pack(pady=5)
btn_green = tk.Button(root, text="Green Text", fg="green")
btn_green.pack(pady=5)
root.mainloop()
Screenshot in Windows
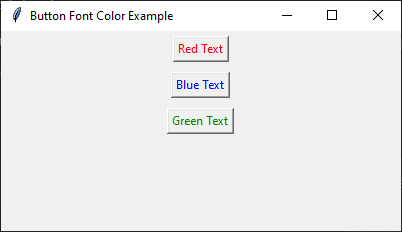
Conclusion
In this tutorial, we explored how to use the fg
option in Tkinter’s Button widget to control the font color of the text. The color can be set using:
- 4-bit per color hex codes (
#rgb
) - 8-bit per color hex codes (
#rrggbb
) - 12-bit per color hex codes (
#rrrgggbbb
) - Standard color names (
'red'
,'blue'
, etc.)
By customizing the text color, you can enhance the visual appeal and usability of your Tkinter application.