Set Button Height in Tkinter
In Tkinter, the height
option of a Button widget allows you to control its height. The height is measured in text lines when using text and in pixels when using an image. The value assigned to the height
option must be an integer.
To set a specific height for a Button in Tkinter, pass the height
parameter with the required number of text lines or pixels while creating the Button.
In this tutorial, we will go through examples using the height
option to modify the size of a Tkinter Button.
Examples
1. Setting Button Height in Tkinter
In this example, we will create a Tkinter window with buttons of different heights.
The first button will have a height of 2 text lines.
tk.Button(root, text="Submit", height=2)
And the second button will have a height of 4 text lines.
tk.Button(root, text="Login", height=4)
main.py
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Tkinter Button Height Example")
root.geometry("400x200")
# Create buttons with different heights
btn_submit = tk.Button(root, text="Submit", height=2)
btn_submit.pack(pady=10)
btn_login = tk.Button(root, text="Login", height=4)
btn_login.pack(pady=10)
# Run the main event loop
root.mainloop()
Screenshot in macOS
Screenshot in Windows
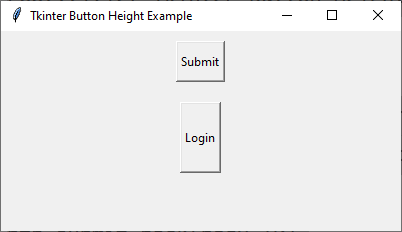
2. Setting Button (with Image) Height in Pixels
In this example, we will create a Button with an image and set the button height to 150 pixels.
tk.Button(root, image=image, command=on_click, height=150)
The actual height of the image is 100 pixels. Since we have specified a height of 150 pixels for the button, the image is placed in the center of the button.
main.py
import tkinter as tk
from tkinter import PhotoImage
root = tk.Tk()
root.title("Image Button Example - tutorialkart.com")
root.geometry("400x200")
def on_click():
print("Image Button Clicked!")
# Load an image
image = PhotoImage(file="button_icon.png") # Replace with a valid image path
# Create an image button
button = tk.Button(root, image=image, command=on_click, height=150)
button.pack(pady=20)
root.mainloop()
Screenshot in macOS
Screenshot in Windows
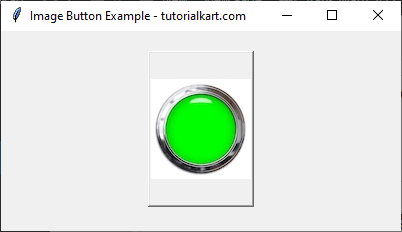
Conclusion
In this tutorial, we explored how to use the height
option in Tkinter’s Button widget to control button height. The height is measured in text lines for text buttons and in pixels for image buttons. By setting different height values, you can adjust the appearance of buttons in your Tkinter application.