Python Tkinter Entry
Tkinter Entry widget allows user to input a single line of text via GUI application.
In this tutorial, we will learn how to create an Entry widget and how to use it read a string from user.
Create Tkinter Entry Widget
To create an Entry widget in your GUI application, use the following sytnax.
widget = tk.Entry(parent, option, ...)
where parent is the root window or a frame in which we would like to pack this Entry widget. You can provide one or more options supported for Entry widget to change its appearance and behavior.
Example 1 – Tkinter Entry
In this example, we will create a Tkinter Entry widget, and read value entered by user in the Entry widget.
example.py – Python Program
import tkinter as tk
window_main = tk.Tk(className='Tkinter - TutorialKart', )
window_main.geometry("400x200")
entry_1 = tk.StringVar()
entry_widget_1 = tk.Entry(window_main, textvariable=entry_1)
entry_widget_1.pack()
def submitValues():
print(entry_1.get())
submit = tk.Button(window_main, text="Submit", command=submitValues)
submit.pack()
window_main.mainloop()
entry_1 variable is bind to the entry_widget_1 to store the value entered to the Entry widget.
When user clicks submit button, we call submitValues() function. In this function, we are reading the value entered in Entry widget, using entry_1.get().
Output
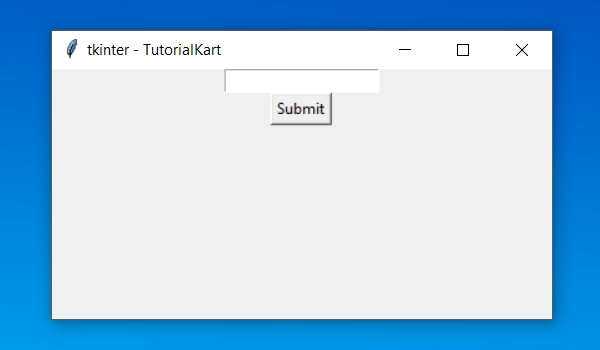
Enter some text in the Entry widget as shown below.
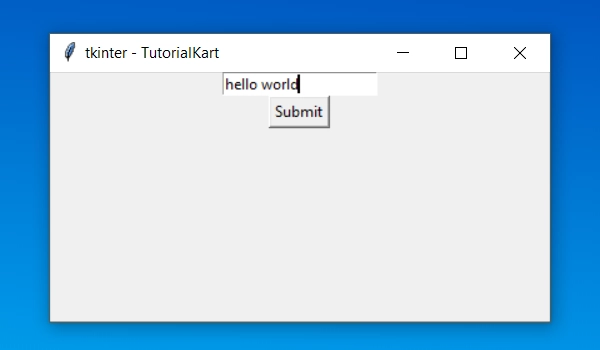
Click on Submit button, and you will get the entry value printed to the console.
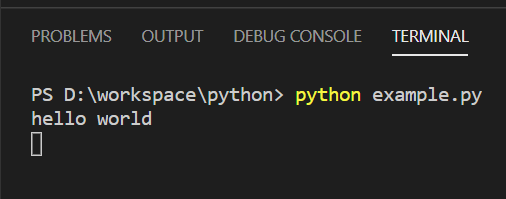
Conclusion
In this Python Tutorial, we learned how to create an Entry widget in our GUI application, and how to work with it, with the help of example programs.