Events Available for Text Widget
In Tkinter, the Text
widget allows users to enter and edit multi-line text. Events in Tkinter enable interaction with this widget by detecting user actions like keypresses, mouse clicks, focus changes, and more.
By binding events to a Text widget, we can trigger specific functions when certain actions occur. In this tutorial, we will explore various events available for the Tkinter Text widget along with practical examples.
Examples
1. Detecting Key Press Events
This example detects when a key is pressed inside the Text widget and displays the pressed key in the console.
We create a function on_key_press(event)
that prints the key that was pressed. This function is bound to the <KeyPress>
event using text_widget.bind("<KeyPress>", on_key_press)
.
main.py
import tkinter as tk
root = tk.Tk()
root.title("Text Widget Events - tutorialkart.com")
root.geometry("400x200")
def on_key_press(event):
print(f"Key Pressed: {event.char}")
text_widget = tk.Text(root, height=5, width=30)
text_widget.pack(pady=10)
text_widget.bind("<KeyPress>", on_key_press)
root.mainloop()
Output in Windows:
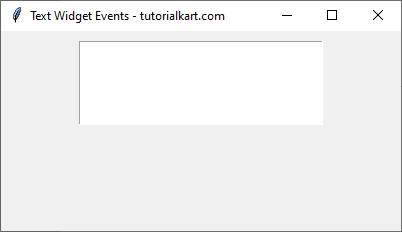
When a key is pressed inside the Text widget, the pressed key appears in the console.
Let us start typing some characters in the text widget, say ‘Hello’.
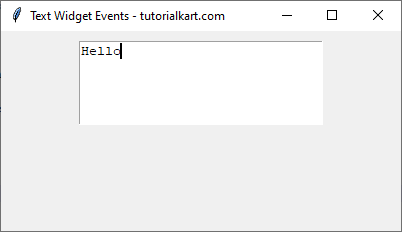
The following is the console output, where the on_key_press() function is called for each key press event performed in the text widget.
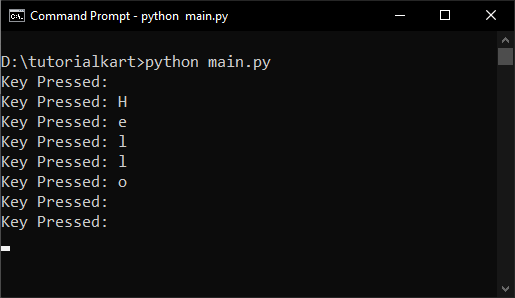
2. Detecting Mouse Click Events
This example detects when the user clicks inside the Text widget and prints the mouse position.
We define the function on_mouse_click(event)
to display the cursor position. This function is bound to the <Button-1>
(left mouse button click) event using text_widget.bind("<Button-1>", on_mouse_click)
.
main.py
import tkinter as tk
root = tk.Tk()
root.title("Text Widget Events - tutorialkart.com")
root.geometry("400x200")
def on_mouse_click(event):
print(f"Mouse clicked at position: {event.x}, {event.y}")
text_widget = tk.Text(root, height=5, width=30)
text_widget.pack(pady=10)
text_widget.bind("<Button-1>", on_mouse_click)
root.mainloop()
Output in Windows:
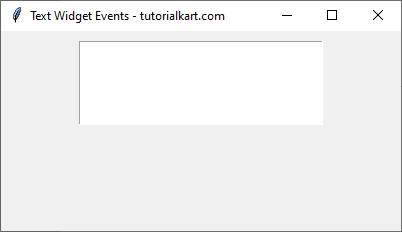
When the user clicks inside the Text widget, the cursor position (x, y) is printed in the console.
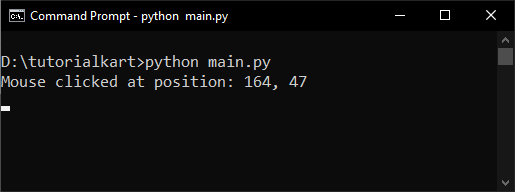
3. Detecting Text Selection Events
This example detects when the user selects text inside the Text widget and prints the selected text.
The function on_text_select(event)
retrieves the selected text using text_widget.get("sel.first", "sel.last")
. We bind this function to the <ButtonRelease-1>
event, which occurs after the mouse button is released following a selection.
main.py
import tkinter as tk
root = tk.Tk()
root.title("Text Widget Events - tutorialkart.com")
root.geometry("400x200")
def on_text_select(event):
try:
selected_text = text_widget.get("sel.first", "sel.last")
print(f"Selected text: {selected_text}")
except tk.TclError:
pass # No selection
text_widget = tk.Text(root, height=5, width=30)
text_widget.pack(pady=10)
text_widget.bind("<ButtonRelease-1>", on_text_select)
root.mainloop()
Output in Windows:
When the user selects text inside the Text widget, the selected text is printed in the console.
Let us say that we have typed ‘Hello World!’ into the text widget, and then selected the ‘World!’. When we selected the text, on_text_select() function is called.
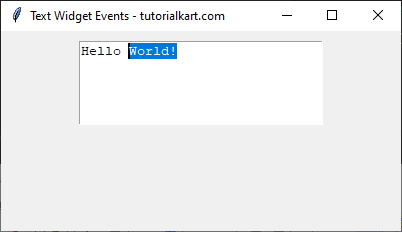
And the function printed the selected text to the console output.
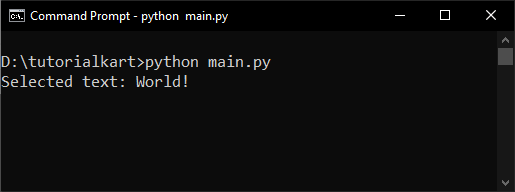
4. Detecting Focus Events
This example detects when the Text widget gains or loses focus.
We define on_focus_in(event)
and on_focus_out(event)
functions to print messages when the Text widget is focused or unfocused. These functions are bound to the <FocusIn>
and <FocusOut>
events, respectively.
main.py
import tkinter as tk
root = tk.Tk()
root.title("Text Widget Events - tutorialkart.com")
root.geometry("400x200")
def on_focus_in(event):
print("Text widget gained focus")
def on_focus_out(event):
print("Text widget lost focus")
text_widget = tk.Text(root, height=5, width=30)
text_widget.pack(pady=10)
text_widget.bind("<FocusIn>", on_focus_in)
text_widget.bind("<FocusOut>", on_focus_out)
root.mainloop()
Output in Windows:
When the user clicks inside the Text widget, “Text widget gained focus” is printed in the console. When the user clicks outside, “Text widget lost focus” is displayed.
Let us say that we clicked inside the text widget.
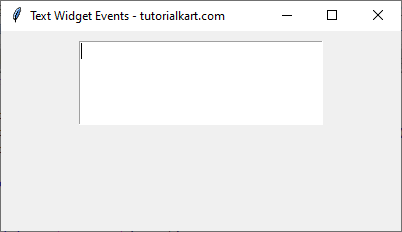
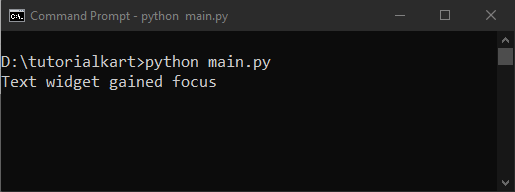
When you click somewhere outside the text widget so that it loses focus, you would get the following output.
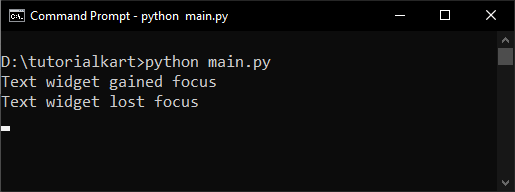
Conclusion
In this tutorial, we explored various events available for the Tkinter Text
widget and how to bind them to functions:
<KeyPress>
– Detects key presses<Button-1>
– Detects mouse clicks<ButtonRelease-1>
– Detects text selection<FocusIn>
and<FocusOut>
– Detect focus changes