Allow Only Integer in Entry Widget in Tkinter Python
In Tkinter, the Entry
widget allows users to input text. However, to ensure that only integers are entered, we can use the validate
and validatecommand
options along with a validation function.
The validate
option is set to 'key'
so that validation happens as the user types, and validatecommand
links to a function that checks whether the entered value is a valid integer.
Example
In this example, we will create a Tkinter window with an Entry widget that only allows integer values. If the user tries to enter non-numeric characters, they will be automatically prevented.
main.py
import tkinter as tk
# Function to validate integer input
def validate_input(P):
if P.isdigit() or P == "":
return True
return False
# Create the main window
root = tk.Tk()
root.title("Allow Only Integer in Entry - tutorialkart.com")
root.geometry("400x200")
# Register validation function
vcmd = root.register(validate_input)
# Create Entry widget with validation
entry = tk.Entry(root, validate="key", validatecommand=(vcmd, "%P"))
entry.pack(pady=20)
# Create a label
label = tk.Label(root, text="Enter an integer:")
label.pack(pady=5)
# Run the application
root.mainloop()
Output in Windows:
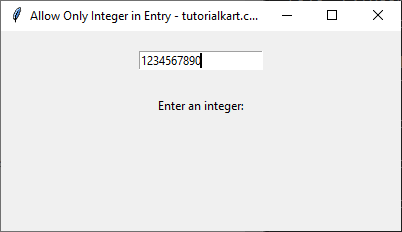
The Entry widget will only accept numeric input. Any attempt to enter letters or special characters will be blocked.
Conclusion
In this tutorial, we learned how to restrict input in a Tkinter Entry widget to only allow integer values. This is achieved using the validate
and validatecommand
options in combination with a validation function. This method helps prevent incorrect user input and ensures data consistency in Tkinter applications.