Allow Only Numbers in Text Widget in Tkinter Python
In Tkinter, we can restrict user input in a Text
widget to allow only numeric values using validation techniques. This is useful for applications that require numeric input, such as calculators, data entry forms, or any number-based inputs.
To enforce numeric-only input, we can use custom validation function combined with key events. In this tutorial, we will go through examples demonstrating how to restrict input in a Tkinter Text widget to only allow numbers.
Examples
1. Restricting Input to Numbers in Text Widget Using Event Binding
In this example, we will use the bind()
method to capture keypress events and restrict input to numeric values only.
The program listens for keypress events on the Text widget and allows only numbers (0-9) and the Backspace key. The on_key_press()
function checks the event’s keycode and prevents non-numeric input by returning "break"
to stop the event propagation.
main.py
import tkinter as tk
# Function to handle keypress event
def on_key_press(event):
if event.char.isdigit() or event.keysym == "BackSpace":
return
return "break"
# Create the main window
root = tk.Tk()
root.title("Restrict Input - tutorialkart.com")
root.geometry("400x200")
# Create a Text widget
text_widget = tk.Text(root, height=1, width=20)
text_widget.pack(pady=20)
# Bind keypress event
text_widget.bind("<KeyPress>", on_key_press)
root.mainloop()
Output in Windows:
A Text widget appears where users can enter only numeric values. Any non-numeric characters are automatically blocked when typed.
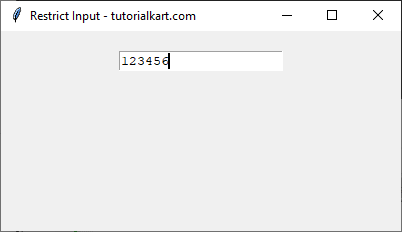
Conclusion
In this tutorial, we explored two different ways to allow only numeric input in a Tkinter Text widget:
- Using
validatecommand
with a validation function. - Using event binding to filter keypress events.