Auto-resize Entry Widget based on Input in Tkinter Python
In Tkinter, an Entry
widget is used to accept user input. By default, its width remains fixed, but you can make it auto-resize based on the length of the input text. This is useful for dynamically adjusting the size of the input field to enhance the user experience.
The resizing of the Entry
widget is achieved by dynamically updating the width based on the number of characters entered.
In this tutorial, we will go through examples demonstrating how to auto-resize the Entry
widget in a Tkinter application.
Examples
1. Basic Auto-resizing Entry Widget
In this example, the Entry widget’s width adjusts dynamically based on the length of the input.
import tkinter as tk
root = tk.Tk()
root.title("Auto-resize Entry - tutorialkart.com")
root.geometry("400x200")
def update_width(event):
entry.config(width=len(entry.get()) + 1)
# Create an Entry widget
entry = tk.Entry(root, width=10)
entry.pack(pady=20)
# Bind the function to key release event
entry.bind("<KeyRelease>", update_width)
root.mainloop()
Output in Windows:
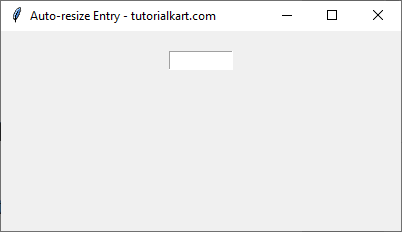
The Entry widget expands or shrinks based on the number of characters entered.
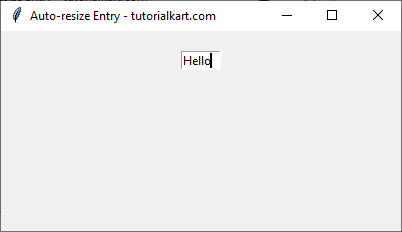
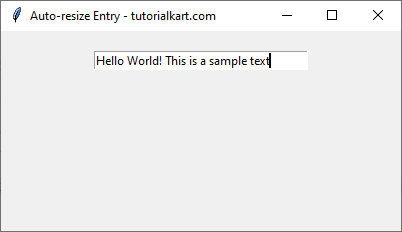
2. Auto-resize with a Maximum Width
To prevent excessive resizing, we can set a maximum width limit.
import tkinter as tk
root = tk.Tk()
root.title("Auto-resize Entry - tutorialkart.com")
root.geometry("400x200")
def update_width(event):
max_width = 30 # Maximum width limit
new_width = min(len(entry.get()) + 1, max_width)
entry.config(width=new_width)
# Create an Entry widget
entry = tk.Entry(root, width=10)
entry.pack(pady=20)
# Bind the function to key release event
entry.bind("<KeyRelease>", update_width)
root.mainloop()
Output in Windows:
Let us start typing in the Entry field.
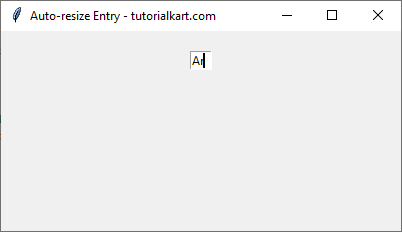
The Entry widget expands as text is entered but does not exceed 30 characters in width.
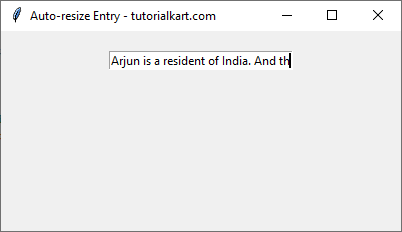
3. Auto-resize with Dynamic Font Scaling
This example adjusts both the widget size and the font size dynamically based on input length.
import tkinter as tk
root = tk.Tk()
root.title("Auto-resize Entry - tutorialkart.com")
root.geometry("400x200")
def update_entry(event):
text_length = len(entry.get())
new_width = text_length + 1
new_font_size = min(20, 8 + text_length // 2) # Increase font size gradually
entry.config(width=new_width, font=("Arial", new_font_size))
# Create an Entry widget
entry = tk.Entry(root, width=10, font=("Arial", 10))
entry.pack(pady=20)
# Bind the function to key release event
entry.bind("<KeyRelease>", update_entry)
root.mainloop()
Output in Windows:
The Entry widget expands and the font size increases as the user types.
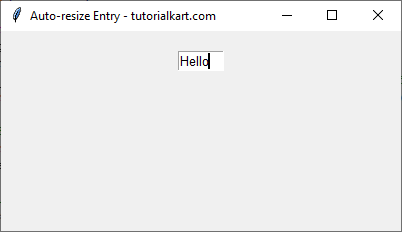
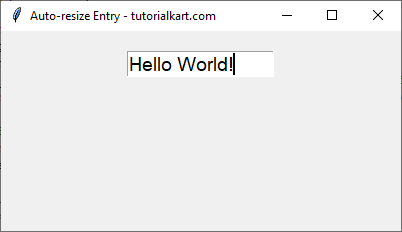
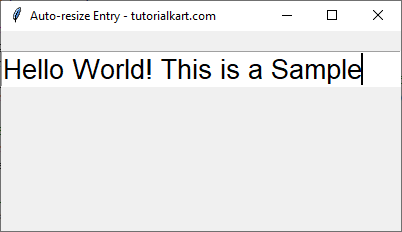
Conclusion
In this tutorial, we explored different ways to make a Tkinter Entry
widget auto-resize based on input:
- Basic auto-resizing by adjusting the width dynamically.
- Setting a maximum width limit to prevent excessive resizing.
- Enhancing the experience by scaling the font size dynamically.