Auto-resize Entry Widget in Tkinter Python
In Tkinter, the Entry
widget is used to accept user input. By default, it has a fixed size, but it can be configured to automatically resize based on window resizing or content size. This can be achieved using the fill
, expand
, and sticky
options.
In this tutorial, we will go through different methods to auto-resize an Entry widget in Tkinter.
Examples
1. Auto-resizing Entry Using pack()
Layout
Using the pack()
layout manager with fill='x'
and expand=True
, we can make the Entry widget stretch horizontally as the window resizes.
main.py
import tkinter as tk
root = tk.Tk()
root.title("Auto-resizing Entry - tutorialkart.com")
root.geometry("400x200")
# Create an Entry widget
entry = tk.Entry(root, font=("Arial", 14))
# Use pack() with fill and expand to resize dynamically
entry.pack(fill="x", expand=True, padx=10, pady=20)
root.mainloop()
Output in Windows:
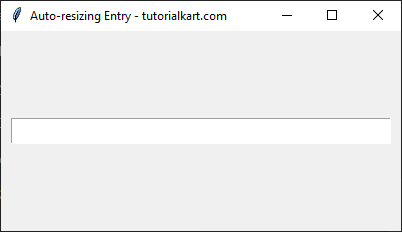
2. Auto-resizing Entry Using grid()
Layout
Using the grid()
layout manager, we set the Entry widget to expand dynamically by configuring row and column weights.
main.py
import tkinter as tk
root = tk.Tk()
root.title("Auto-resizing Entry - tutorialkart.com")
root.geometry("600x100")
# Configure grid layout
root.grid_columnconfigure(0, weight=1)
root.grid_rowconfigure(0, weight=1)
# Create an Entry widget
entry = tk.Entry(root, font=("Arial", 14))
# Use grid() to place it in column 0, row 0, and set it to stretch
entry.grid(row=0, column=0, sticky="ew", padx=10, pady=20)
root.mainloop()
Output in Windows:
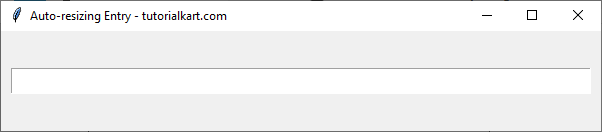
3. Auto-resizing Entry Using place()
with Relative Sizing
The place()
manager allows us to define relwidth=1.0
, ensuring that the Entry widget resizes with the window.
main.py
import tkinter as tk
root = tk.Tk()
root.title("Auto-resizing Entry - tutorialkart.com")
root.geometry("400x200")
# Create an Entry widget
entry = tk.Entry(root, font=("Arial", 14))
# Use place() to make it resize dynamically
entry.place(relx=0.5, rely=0.5, anchor="center", relwidth=0.9)
root.mainloop()
Output in Windows:
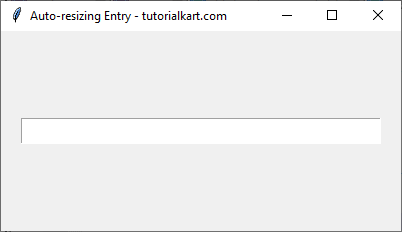
Conclusion
In this tutorial, we explored different ways to auto-resize an Entry widget in Tkinter:
- Using
pack(fill="x", expand=True)
to allow horizontal resizing. - Using
grid(sticky="ew")
with column weight configuration. - Using
place(relwidth=1.0)
for relative positioning.