Auto-resize Text Widget based on Input in Tkinter Python
In Tkinter, the Text
widget is used for multi-line text input. By default, the widget does not resize itself dynamically as the user types. However, we can enable auto-resizing behavior by dynamically adjusting the widget’s height and width based on the content.
To make a Text
widget auto-resize, we monitor changes in the input and adjust the size accordingly using event bindings
and the update_idletasks()
method.
In this tutorial, we will explore different ways to implement an auto-resizing Text
widget in a Tkinter application.
Examples
1. Auto-resizing Text Widget Based on Line Count
In this example, we create a Text
widget that expands vertically as the number of lines increases.
The program listens for the <KeyRelease>
event, and each time the user types, it checks the number of lines using text_widget.index("end-1c")
. We then update the widget height dynamically by setting text_widget.config(height=new_height)
.
main.py
import tkinter as tk
# Create main application window
root = tk.Tk()
root.title("Auto-resizing Text Widget - tutorialkart.com")
root.geometry("400x200")
def adjust_height(event):
"""Adjust the height of the Text widget dynamically."""
line_count = int(text_widget.index("end-1c").split('.')[0]) # Get the number of lines
new_height = min(max(line_count, 2), 10) # Keep height between 2 and 10 lines
text_widget.config(height=new_height) # Update text widget height
# Create Text widget with initial height
text_widget = tk.Text(root, height=2, width=40, wrap="word")
text_widget.pack(pady=10)
# Bind key release event to adjust height
text_widget.bind("<KeyRelease>", adjust_height)
root.mainloop()
Output in Windows:
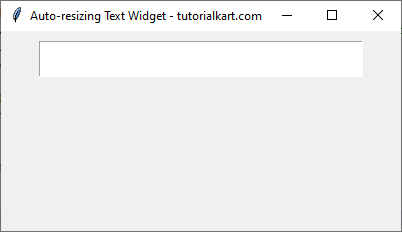
As the user types more lines, the height of the Text
widget increases dynamically, up to a maximum of 10 lines.
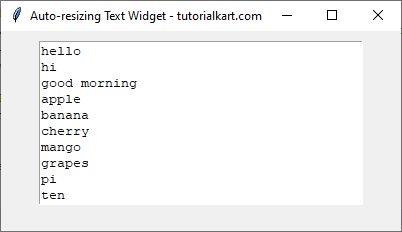
2. Auto-resizing Text Widget Based on Character Count
In this example, we expand the Text
widget dynamically based on the number of characters entered.
The program calculates the number of characters using len(text_widget.get("1.0", "end-1c"))
. We determine the required width by dividing the character count by an average character width per line. If the width exceeds a threshold, we increase the widget’s width.
main.py
import tkinter as tk
root = tk.Tk()
root.title("Auto-resizing Text Widget - tutorialkart.com")
root.geometry("400x200")
def adjust_width(event):
"""Adjust the width of the Text widget based on character count."""
char_count = len(text_widget.get("1.0", "end-1c")) # Get total character count
new_width = min(max(char_count, 20), 50) # Adjust width dynamically
text_widget.config(width=new_width) # Update text widget width
# Create Text widget with initial width
text_widget = tk.Text(root, height=3, width=20, wrap="word")
text_widget.pack(pady=10)
# Bind key release event to adjust width
text_widget.bind("<KeyRelease>", adjust_width)
root.mainloop()
Output in Windows:
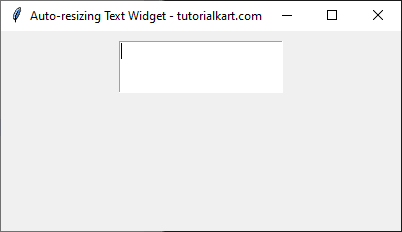
As the user types more characters, the width of the Text
widget expands dynamically, up to a maximum of 50 characters per line.
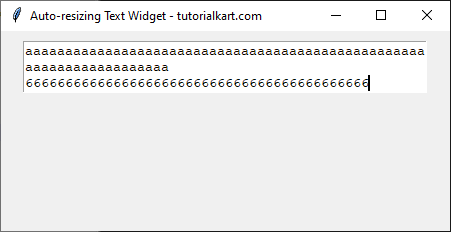
Conclusion
In this tutorial, we explored different methods to auto-resize a Text
widget in Tkinter. We covered:
- Auto-resizing the height of the
Text
widget based on the number of lines. - Auto-resizing the width of the
Text
widget based on the number of characters.