Call a Function on Button Click in Tkinter
In Tkinter, you can call a function when a button is clicked using the command
parameter of the Button
widget. This allows you to trigger specific actions when the user interacts with the button.
To call a function on a button click, define the function first and pass it to the command
argument while creating the button.
In this tutorial, we will go through multiple examples demonstrating different ways to call a function when a Tkinter button is clicked.
Examples
1. Calling a Function on Button Click
In this example, we will create a Tkinter button that calls a function to print a message when clicked.
main.py
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Button Click Example - tutorialkart.com")
root.geometry("400x200")
# Function to be called when button is clicked
def on_button_click():
print("Button Clicked!")
# Create a button and set command
button = tk.Button(root, text="Click Me", command=on_button_click)
button.pack(pady=20)
# Run the main event loop
root.mainloop()
Output in Windows
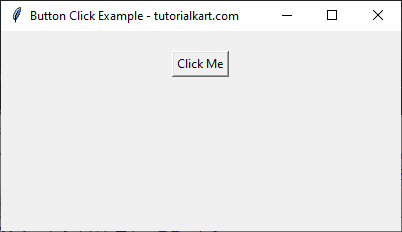
Click the button, and you will see the on_button_click()
function called and the message printed to the console output.
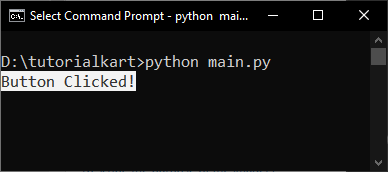
2. Updating Label Text on Button Click
In this example, we will update the text of a label when the button is clicked.
main.py
import tkinter as tk
root = tk.Tk()
root.title("Update Label Example - tutorialkart.com")
root.geometry("400x200")
# Function to update label text
def update_label():
label.config(text="Button was clicked!")
# Create a label
label = tk.Label(root, text="Click the button")
label.pack(pady=10)
# Create a button to update label text
button = tk.Button(root, text="Click Me", command=update_label)
button.pack(pady=10)
root.mainloop()
Output in Windows
Initially, the label displays “Click the button.”
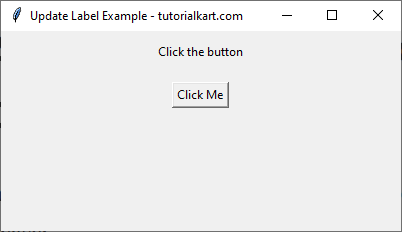
When the button is clicked, it updates to “Button was clicked!”
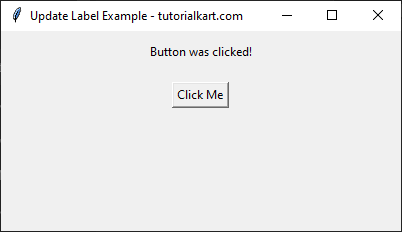
3. Calling a Function with Arguments on Button Click
In this example, we will pass arguments to the function using lambda
when calling it on a button click.
main.py
import tkinter as tk
root = tk.Tk()
root.title("Function with Arguments - tutorialkart.com")
root.geometry("400x200")
# Function with parameters
def greet(name):
print(f"Hello, {name}!")
# Create a button and pass arguments using lambda
button = tk.Button(root, text="Greet John", command=lambda: greet("John"))
button.pack(pady=10)
root.mainloop()
Output in Windows
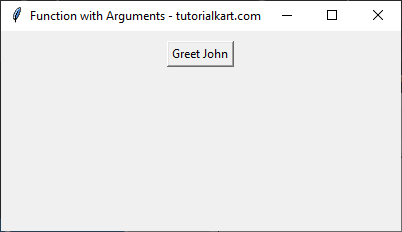
When the button is clicked, “Hello, John!” is printed in the console.
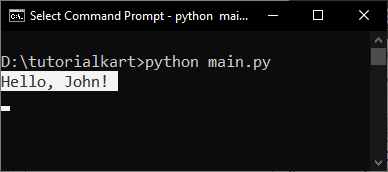
4. Disabling the Button After Click
In this example, the button will be disabled after being clicked once.
main.py
import tkinter as tk
root = tk.Tk()
root.title("Disable Button Example - tutorialkart.com")
root.geometry("400x200")
# Function to disable the button
def disable_button():
button.config(state=tk.DISABLED)
print("Button Disabled")
# Create a button
button = tk.Button(root, text="Click to Disable", command=disable_button)
button.pack(pady=10)
root.mainloop()
Output in Windows
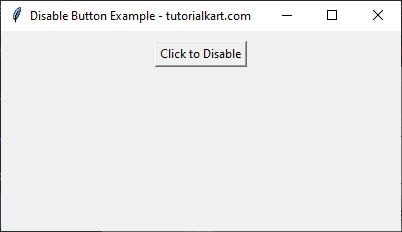
After clicking the button, it gets disabled and cannot be clicked again.
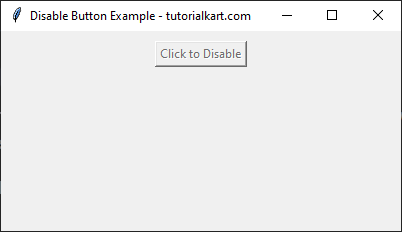
Conclusion
In this tutorial, we explored different ways to call a function when a button is clicked in Tkinter:
- Calling a simple function on a button click.
- Updating a label’s text when a button is clicked.
- Using
lambda
to pass arguments to the function. - Disabling the button after it is clicked.
By using the command
option in the Button widget, you can easily handle user interactions in your Tkinter application.