Call a Function When the Text Changes in a Text Widget in Tkinter Python
In Tkinter, the Text
widget is used to display and edit multiple lines of text. To detect when the text in the widget changes and trigger a function, we use the trace_add()
method with a Tkinter variable or set a custom event binding with the <<Modified>>
event.
Examples
1. Detecting Text Changes Using <<Modified>> Event in a Text Widget
In this example, we will detect when the user types in a Text
widget and display the updated content in the console.
We bind the <<Modified>>
event to a function on_text_change()
, which retrieves the content of the Text
widget and prints it to the console. After processing the event, we reset the modified flag using text_widget.edit_modified(False)
to allow continuous detection.
main.py
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Text Change Detection - tutorialkart.com")
root.geometry("400x200")
def on_text_change(event):
"""Function to handle text changes."""
text_content = text_widget.get("1.0", "end-1c") # Get the current text
print("Current text:", text_content)
text_widget.edit_modified(False) # Reset the modified flag
# Create a Text widget
text_widget = tk.Text(root, wrap="word", height=5)
text_widget.pack(pady=10)
# Bind the <<Modified>> event to the text widget
text_widget.bind("<<Modified>>", on_text_change)
# Run the Tkinter event loop
root.mainloop()
Output in Windows:
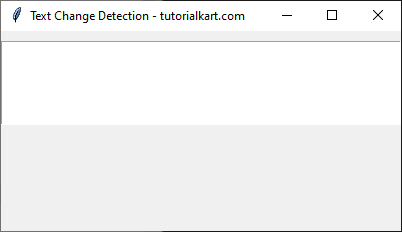
When the user types in the text widget, the updated text is printed in the console.
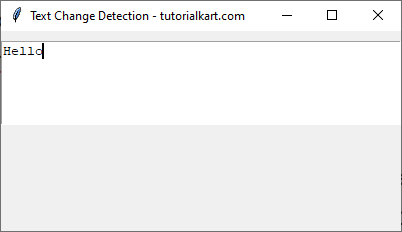
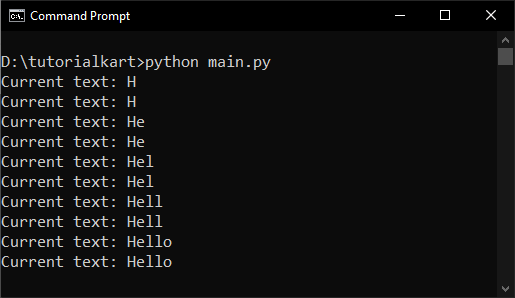
The function is called twice during each change, because once it is called because of a change in the text, and for second time it is called because we are updating the modified flag to False.
2. Counting Characters as Text Changes in Text Widget
In this example, we will count the number of characters in the Text
widget as the user types and display the count in a label.
We define the function update_character_count()
, which retrieves the current text using text_widget.get("1.0", "end-1c")
and calculates its length. This value is then updated in the char_count_label
. We bind this function to the <<Modified>>
event and reset the modified flag using text_widget.edit_modified(False)
to ensure continuous detection.
main.py
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Character Counter - tutorialkart.com")
root.geometry("400x200")
def update_character_count(event):
"""Update the character count whenever text changes."""
text_content = text_widget.get("1.0", "end-1c") # Get text content
char_count_label.config(text=f"Character Count: {len(text_content)}")
text_widget.edit_modified(False) # Reset modified flag
# Create a Text widget
text_widget = tk.Text(root, wrap="word", height=5)
text_widget.pack(pady=10)
# Create a label to display character count
char_count_label = tk.Label(root, text="Character Count: 0")
char_count_label.pack(pady=5)
# Bind the <<Modified>> event to track text changes
text_widget.bind("<<Modified>>", update_character_count)
# Run the Tkinter event loop
root.mainloop()
Output in Windows:
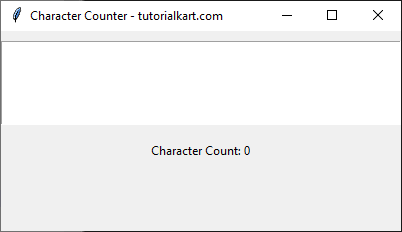
As the user types in the text widget, the character count is updated dynamically in the label.
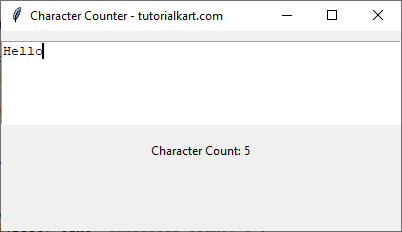
Since we have typed ‘Hello’ which has five characters, the Character Count is 5.
Conclusion
In this tutorial, we explored how to detect text changes in a Tkinter Text
widget using the <<Modified>>
event. We implemented two examples:
- Printing the updated text to the console when the text changes.
- Tracking the number of characters typed and displaying the count in a label.
By binding a function to the <<Modified>>
event and resetting the modified flag using edit_modified(False)
, we ensure that text changes are detected continuously.