Call a Function with Arguments on Button Click in Tkinter
In Tkinter, the command
option of a Button widget allows you to specify a function to be executed when the button is clicked. However, passing arguments directly to the function requires a special approach.
To call a function with arguments on a button click, you can use:
- The
lambda
function - The
functools.partial
method
In this tutorial, we will explore both methods with examples.
Examples
1. Calling a Function with Arguments Using lambda
We use a lambda
function to pass arguments to a function when the button is clicked.
main.py
import tkinter as tk
# Function with arguments
def greet(name):
print(f"Hello, {name}!")
root = tk.Tk()
root.title("Tkinter Button Example - tutorialkart.com")
root.geometry("400x200")
# Button calling function with argument using lambda
button = tk.Button(root, text="Greet John", command=lambda: greet("John"))
button.pack(pady=10)
root.mainloop()
Output in Windows:
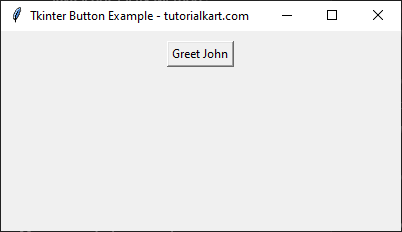
When the button is clicked, Hello, John!
is printed in the console.
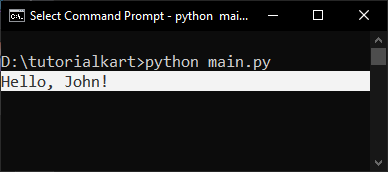
2. Calling a Function with Arguments Using functools.partial
Using functools.partial
allows passing arguments to a function without using lambda
.
main.py
import tkinter as tk
from functools import partial
# Function with arguments
def display_message(msg):
print(msg)
root = tk.Tk()
root.title("Tkinter Button Example - tutorialkart.com")
root.geometry("400x200")
# Button calling function with argument using functools.partial
button = tk.Button(root, text="Show Message", command=partial(display_message, "Welcome to tutorialkart.com!"))
button.pack(pady=10)
root.mainloop()
Output in Windows:
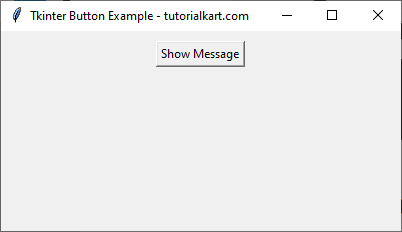
When the button is clicked, Welcome to tutorialkart.com!
is printed in the console.
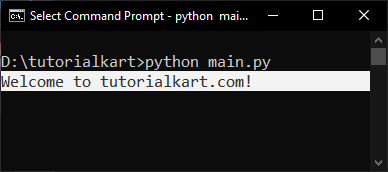
Conclusion
In this tutorial, we explored how to pass arguments to a function when a Tkinter button is clicked using:
lambda
functionsfunctools.partial
Both methods provide flexible ways to execute functions with parameters in Tkinter applications.