Center Align Text in Entry Widget in Tkinter Python
In Tkinter, the justify
option of an Entry widget allows you to align the text inside the input field. It supports the following values:
LEFT
– Aligns text to the left (default).CENTER
– Aligns text in the center.RIGHT
– Aligns text to the right.
In this tutorial, we will explore different methods to center align text in a Tkinter Entry widget.
Examples
1. Center Align Text in Entry Widget Using the justify Option
In this example, we create an Entry widget and set the text alignment to CENTER
using the justify
option.
</>
Copy
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Center Align Entry - tutorialkart.com")
root.geometry("400x200")
# Create an Entry widget with center alignment
entry = tk.Entry(root, justify="center", font=("Arial", 14))
entry.pack(pady=20)
# Run the Tkinter event loop
root.mainloop()
Output in Windows:
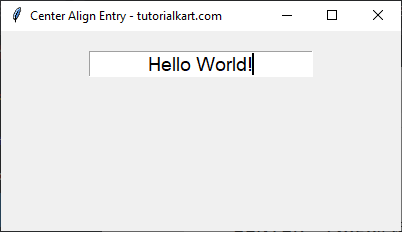
2. Center Align Text in Entry Widget with Default Text
In this example, we add a default text in the Entry widget and ensure it appears centered.
</>
Copy
import tkinter as tk
root = tk.Tk()
root.title("Center Align Entry - tutorialkart.com")
root.geometry("400x200")
# Create an Entry widget with default text and center alignment
entry = tk.Entry(root, justify="center", font=("Arial", 14))
entry.pack(pady=20)
# Insert default text
entry.insert(0, "Enter your name")
root.mainloop()
Output in Windows:
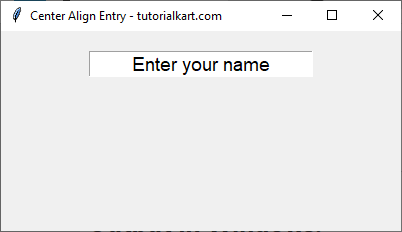
3. Center Align Entry Widget with Custom Width
In this example, we adjust the width of the Entry widget to control the text alignment visually.
</>
Copy
import tkinter as tk
root = tk.Tk()
root.title("Center Align Entry - tutorialkart.com")
root.geometry("400x200")
# Create an Entry widget with center alignment and custom width
entry = tk.Entry(root, justify="center", font=("Arial", 14), width=30)
entry.pack(pady=20)
# Insert default text
entry.insert(0, "Custom Width Example")
root.mainloop()
Output in Windows:
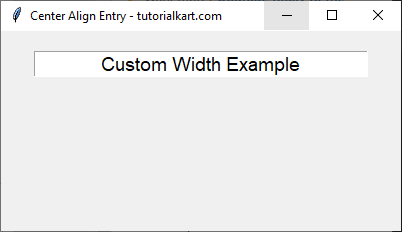
Conclusion
In this tutorial, we explored different ways to center align text in a Tkinter Entry widget using the justify
option. We covered:
- Basic center alignment using
justify="center"
. - Center alignment with default text.
- Adjusting the width for better alignment control.