Center Align Text in Label in Tkinter Python
In Tkinter, the justify
and anchor
options of a Label
widget allow you to control the alignment of text. To center align the text within a label, we can use:
justify="center"
: Aligns text inside a multi-line label to the center.anchor="center"
: Aligns the label itself to the center of its allocated space.
In this tutorial, we will go through multiple examples demonstrating how to center-align text in a Tkinter Label.
Examples
1. Center Align Text in a Single-Line Label
In this example, we will create a Tkinter window and add a label with center-aligned text.
import tkinter as tk
root = tk.Tk()
root.title("Center Align Label - tutorialkart.com")
root.geometry("400x200")
# Create a label with center-aligned text
label = tk.Label(root, text="Hello, Tkinter!", anchor="center")
label.pack(expand=True)
root.mainloop()
Output in Windows:
A label with the text Hello, Tkinter!
appears at the center of the window.
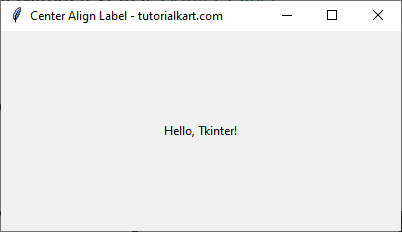
2. Center Align Multi-Line Text in a Label
For multi-line text, we need to use the justify
option to align the text properly.
import tkinter as tk
root = tk.Tk()
root.title("Center Align Label - tutorialkart.com")
root.geometry("400x200")
# Create a label with center-aligned multi-line text
text = "Welcome to Tkinter\nThis is a multi-line label"
label = tk.Label(root, text=text, justify="center")
label.pack(expand=True)
root.mainloop()
Output in Windows:
The multi-line text appears centered inside the label.
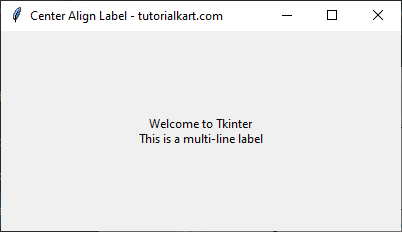
3. Center Align Label Text with Custom Font
In this example, we will set a custom font along with center-aligned text.
import tkinter as tk
root = tk.Tk()
root.title("Center Align Label - tutorialkart.com")
root.geometry("400x200")
# Create a label with custom font and center alignment
label = tk.Label(root, text="Tkinter Label", font=("Arial", 14, "bold"), anchor="center")
label.pack(expand=True)
root.mainloop()
Output in Windows:
The label appears with bold text, centered in the window.
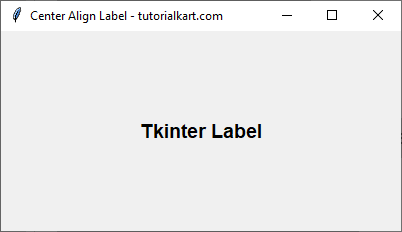
4. Center Align Text with Background and Border
To make the label more visually distinct, we add a background color and a border.
import tkinter as tk
root = tk.Tk()
root.title("Center Align Label - tutorialkart.com")
root.geometry("400x200")
# Create a label with background color and border
label = tk.Label(root, text="Tkinter Label", bg="lightblue", fg="black", borderwidth=2, relief="solid", anchor="center")
label.pack(expand=True, padx=20, pady=20)
root.mainloop()
Output in Windows:
The label appears with a light blue background, black text, and a solid border, centered in the window.
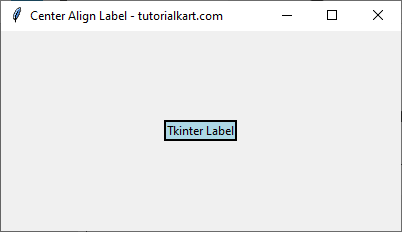
Conclusion
In this tutorial, we explored different ways to center-align text inside a Tkinter Label using:
anchor="center"
for single-line labelsjustify="center"
for multi-line labels- Custom fonts, background colors, and borders
By using these techniques, you can improve the appearance of labels in your Tkinter application.